The Ultimate Guide to Unit Testing: Tips and Techniques
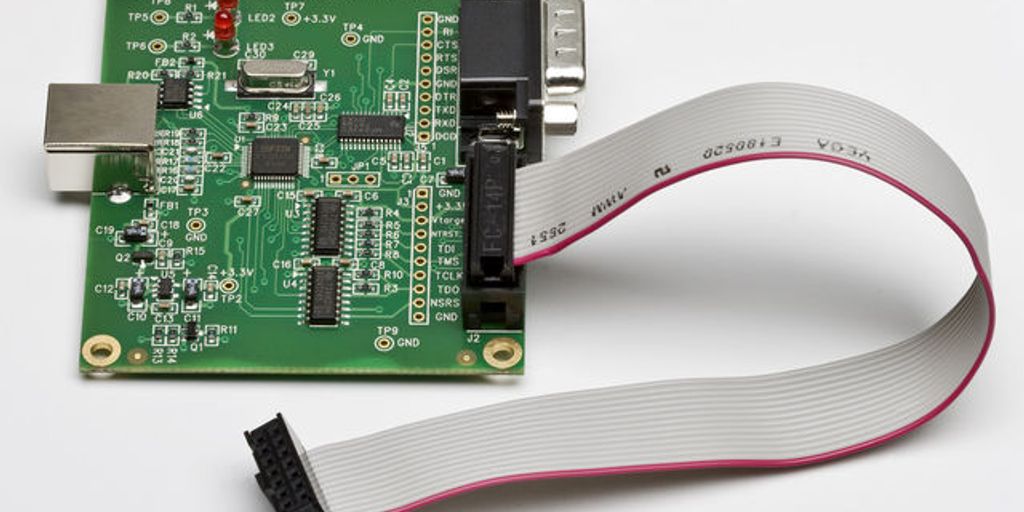
The Ultimate Guide to Unit Testing provides a comprehensive roadmap for both novice and experienced developers looking to enhance their testing skills. This guide covers everything from the basics of unit testing to advanced techniques and automation, ensuring that you can build robust and reliable software. By following the outlined tips and techniques, you’ll gain a deeper understanding of how to effectively implement and optimize unit tests in your development projects.
Key Takeaways
- Understand the core principles and benefits of unit testing to ensure software reliability.
- Learn how to set up a conducive testing environment with the right tools and configurations.
- Master the art of writing clear and effective unit tests that adhere to best practices.
- Explore advanced unit testing techniques to handle complex testing scenarios.
- Implement automation and continuous integration to streamline testing processes and improve efficiency.
Understanding the Basics of Unit Testing
Definition and Importance
Unit testing is a fundamental practice in software development, ensuring that individual components function as expected. It serves as the first line of defense in identifying bugs and issues early in the development cycle, significantly reducing the cost and effort required for later bug fixes.
Key Components of a Unit Test
A unit test typically includes three main components: the unit under test, the test case, and the test result. Each component plays a crucial role in the effectiveness of the test:
- Unit under test: The smallest piece of code that can be isolated in a system for testing.
- Test case: A set of conditions under which a test will determine if a specific behavior or set of behaviors is working as expected.
- Test result: The outcome of the test, usually denoted as passed or failed.
The Role of Unit Testing in Software Development
Unit testing is integral to maintaining high-quality software. It allows developers to make changes confidently, knowing that any new errors will be caught quickly. By integrating unit testing into the development process, teams can ensure that their software is robust, scalable, and maintainable over time.
Setting Up Your Testing Environment
Choosing the Right Tools and Frameworks
Selecting the appropriate tools and frameworks is crucial for effective unit testing. Consider factors like language support, community backing, and integration capabilities. Popular choices include JUnit for Java, pytest for Python, and Mocha for JavaScript.
Configuring Your Development Environment for Testing
Ensure your development environment is optimized for testing by setting up dedicated test databases, configuring server access, and establishing clear paths for test data. This setup reduces conflicts and enhances test reliability.
Best Practices for Test Data Management
Effective test data management involves maintaining data integrity and ensuring data isolation. Use techniques such as:
- Using mock data for tests that require database interactions
- Employing data masking to protect sensitive information
- Refreshing data sets regularly to avoid stale data
Writing Effective Unit Tests
Structuring Your Tests Clearly
Clarity in test structure is crucial for understanding and maintaining tests. Ensure each test is focused on a single functionality, and use descriptive names for test functions.
Applying the AAA Pattern: Arrange, Act, Assert
The AAA (Arrange, Act, Assert) pattern is a powerful way to design your tests. This pattern helps in separating the setup from the test logic and the verification steps, making your tests cleaner and easier to understand.
Dealing with Dependencies and Mocking
Handling dependencies in unit tests can be challenging. Use mocking to isolate the unit being tested, ensuring that tests are not affected by external factors. This approach helps in achieving more reliable and effective unit testing.
Advanced Unit Testing Techniques
Parameterized Testing
Parameterized testing allows you to run the same test with various inputs, enhancing coverage and efficiency. This technique is particularly useful when you want to test different scenarios with the same logic but varying data. It ensures that all cases are tested without duplicating code.
Using Stubs and Spies
Stubs and spies are integral for isolating the unit under test by replacing certain parts of the system. Stubs provide predetermined responses, while spies gather information about function calls. This approach helps in verifying that the units interact correctly without relying on their real implementations.
Integration vs. Unit Testing
While unit testing focuses on the smallest possible unit of code, integration testing checks the interactions between modules. It’s crucial to understand the distinction to ensure that both testing levels are effectively applied in the software development process. Integration tests are typically more complex and require a broader setup compared to unit tests.
Automating and Integrating Unit Tests
Setting Up Continuous Integration
Continuous Integration (CI) is a critical component in the modern development pipeline, ensuring that code changes are automatically built, tested, and merged. Automating tests in CI/CD pipelines is not just a convenience but a necessity to maintain code quality and project velocity.
Automating Test Execution
Automating test execution involves configuring your CI tools to run tests automatically upon code commits or scheduled intervals. This practice helps in identifying issues early and keeping the software in a releasable state at all times.
Monitoring Test Results and Metrics
It’s essential to monitor the outcomes of automated tests to gauge the health of the software. Tools and dashboards are typically used to track failure rates, test coverage, and other critical metrics, providing insights that guide future testing and development efforts.
Handling Common Unit Testing Challenges
Dealing with Flaky Tests
Flaky tests can severely disrupt the reliability of your testing suite. Identify common causes such as asynchronous operations, network dependencies, and non-deterministic data. Strategies to mitigate flakiness include using retries, setting up appropriate timeouts, and ensuring consistent testing environments.
Testing in Legacy Codebases
Legacy codebases often lack sufficient test coverage, making them brittle and hard to maintain. Start by introducing tests for critical paths first, then gradually increase coverage. Refactoring code to make it more testable can also be beneficial, but should be done cautiously to avoid introducing new bugs.
Improving Test Coverage
To enhance the effectiveness of your tests, aim for higher test coverage. This doesn’t mean striving for 100% coverage but focusing on critical areas that affect the application’s functionality. Use coverage tools to identify untested parts of your code and prioritize them in your testing efforts.
Optimizing Unit Test Performance
Speeding Up Test Execution
To reduce the execution time of unit tests, consider implementing parallel testing and optimizing the code within the tests. Utilizing caching and avoiding unnecessary database interactions can also significantly decrease test times.
Reducing Test Redundancies
Identify and eliminate redundant tests to streamline your test suite. This can be achieved by:
- Reviewing test cases for overlap
- Combining similar tests
- Removing outdated or irrelevant tests
Leveraging Parallel Execution
Parallel execution can dramatically improve the performance of your testing suite. Ensure that your tests are designed to run independently to avoid conflicts. This approach not only speeds up the process but also maximizes the utilization of available hardware resources.
Conclusion
In conclusion, mastering unit testing is essential for developing reliable and maintainable software. By integrating the tips and techniques discussed in this guide, developers can enhance their testing strategies, ensure code quality, and facilitate easier maintenance and updates. Remember, effective unit testing not only catches errors early but also supports agile development practices by allowing for rapid iterations. Embrace these practices to make your development process more efficient and your software products more robust.
Frequently Asked Questions
What is unit testing and why is it important?
Unit testing involves testing individual components of software to ensure they work as expected. It’s important because it helps catch bugs early, improves code quality, and facilitates changes in code with confidence.
How do I choose the right tools for unit testing?
Select tools that integrate well with your development environment and support the programming languages you use. Popular unit testing frameworks include JUnit for Java, NUnit for .NET, and Jest for JavaScript.
What is the AAA pattern in unit testing?
The AAA (Arrange, Act, Assert) pattern is a common approach for writing clear and structured unit tests. Arrange sets up the test conditions, Act performs the test, and Assert checks if the test outcome is as expected.
How can I handle dependencies when writing unit tests?
Use mocking frameworks to simulate dependencies. This allows you to isolate the unit of code being tested and ensures your tests are not affected by external factors.
What is the difference between unit testing and integration testing?
Unit testing checks individual components for correct behavior, whereas integration testing verifies that different modules or services work together as expected.
How can I improve the performance of my unit tests?
To improve performance, focus on reducing the complexity of tests, reusing test setups, and running tests in parallel when possible.