How to Write Effective Unit Testing Test Cases
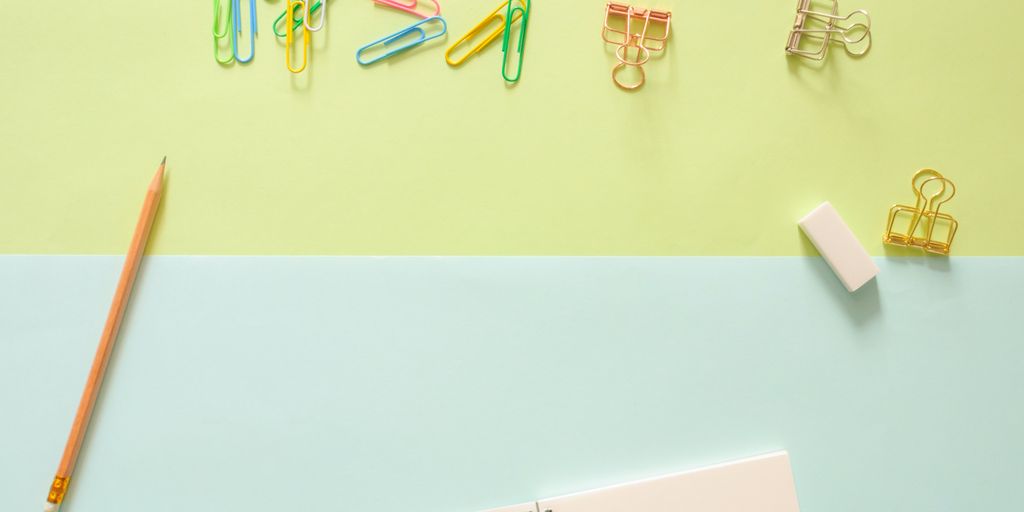
Unit testing is a fundamental practice in software development that helps ensure individual parts of an application work as expected. Writing effective unit testing test cases is crucial for maintaining code quality, reducing bugs, and facilitating easier code refactoring. This article will explore the characteristics, best practices, and strategies for writing effective unit testing test cases.
Key Takeaways
- Effective unit tests should be automated, repeatable, and easy to implement.
- Simple and readable tests are easier to maintain and understand, making debugging and refactoring more straightforward.
- Following the Arrange, Act, Assert pattern helps in structuring tests clearly and logically.
- Decoupling code through unit testing improves code maintainability and makes it easier to test individual components.
- Using descriptive names and organizing test cases properly enhances the readability and manageability of test suites.
Characteristics of Effective Unit Testing Test Cases
Unit testing is a critical aspect of software development, ensuring that individual components of the code work as intended. Effective unit testing test cases share several key characteristics that make them valuable tools in the development process.
Best Practices for Writing Unit Testing Test Cases
Keep Tests Simple and Readable
Write readable, simple tests to ensure your code works as intended. Simple tests are easier to write, maintain, and understand. Additionally, they are easier to refactor. If the test is complex and you need to refactor some code, the tests might break.
Follow the Arrange, Act, Assert Pattern
The Arrange, Act, Assert (AAA) pattern is a common structure for writing unit tests. It involves three steps:
- Arrange: Set up the testing objects and prepare the prerequisites for your test.
- Act: Perform the actual work of the test.
- Assert: Verify that the action of the test produced the expected results.
This pattern helps in keeping the tests clear and structured.
Write Tests Before Fixing Bugs
When you encounter a bug, write a test that replicates the issue before you fix it. This ensures that the bug is well understood and that the fix is verified by the test. It also helps in preventing the same bug from reoccurring in the future.
Decoupling Code Through Unit Testing
Decoupling code is a fundamental practice in software development that enhances the modularity and maintainability of your codebase. Writing tests for your code will naturally decouple your code, because it would be more difficult to test otherwise. This section explores the importance and strategies of decoupling code through unit testing.
Testing Different Scenarios in Unit Testing
Happy Path Testing
Happy path testing focuses on the most common and straightforward scenarios where everything works as expected. Covering the happy path first has many advantages, such as ensuring that the basic functionality of the code is correct before moving on to more complex cases. This type of testing helps in quickly validating the core features and provides a solid foundation for further testing.
Edge Case Testing
Edge case testing involves testing the boundaries and limits of the software. These are scenarios that occur at the extreme ends of the input spectrum. For example, testing with the maximum and minimum values, empty inputs, or very large inputs. Edge case testing is crucial because it helps identify potential issues that may not be apparent during regular usage.
Negative Testing
Negative testing, also known as failure testing, aims to ensure that the software can gracefully handle invalid input or unexpected user behavior. This type of testing is essential for verifying the robustness and reliability of the software. By intentionally providing incorrect or unexpected inputs, negative testing helps in identifying how the system behaves under adverse conditions and ensures that it fails gracefully without causing significant issues.
Improving Test Performance and Reliability
Improving the performance and reliability of your unit tests is crucial for maintaining a robust and efficient testing suite. Fast and reliable tests ensure that developers can quickly identify issues and maintain high code quality.
Organizing and Naming Unit Testing Test Cases
Using Descriptive Names
The name of your test should consist of three parts:
- The name of the method being tested.
- The scenario under which it’s being tested.
- The expected behavior when the scenario is invoked.
Don’t be afraid of long, descriptive names. For example, it('should return 0 for an empty cart')
is much better than it('works for 0')
or it('empty cart')
. Good test names also work as a table of contents when using tests as code documentation. You can quickly find the right test by looking at their names.
Grouping Related Tests
Grouping related tests helps in understanding the functionality being tested and makes the test suite easier to navigate. Consider grouping tests by the functionality they cover or by the class/module they belong to. This can be done using nested describe blocks in frameworks like Mocha or JUnit test suites.
Maintaining Test Suites
Maintaining test suites is crucial for long-term project health. Regularly review and refactor your test cases to ensure they remain relevant and efficient. Remove obsolete tests and update existing ones as the codebase evolves. A well-maintained test suite increases trust in your tests and the code they validate.
Conclusion
In conclusion, writing effective unit testing test cases is a crucial skill for any software development team aiming to enhance code quality and maintainability. By adhering to best practices such as writing simple and readable tests, ensuring tests are automated and repeatable, and decoupling code to facilitate easier testing, teams can significantly reduce development and maintenance costs. Remember, a good unit test is not only about verifying functionality but also about making the codebase more robust and easier to manage. Implement these strategies to ensure your unit tests are both efficient and effective, ultimately leading to a more reliable and maintainable software product.
Frequently Asked Questions
What is unit testing?
Unit testing is a software testing method where individual units or components of a software are tested in isolation from the rest of the application. The goal is to validate that each unit performs as expected.
Why is unit testing important?
Unit testing is important because it helps ensure that individual parts of the code work correctly. This leads to higher code quality, easier maintenance, and quicker identification of bugs.
What are the characteristics of effective unit test cases?
Effective unit test cases should be automated, repeatable, easy to implement, trustworthy, maintainable, and quick to run. They should also be simple and readable.
When should I write unit tests?
It’s best to write unit tests before fixing bugs or adding new features. This practice, known as Test-Driven Development (TDD), helps ensure that the new code works as expected and does not break existing functionality.
What is the Arrange, Act, Assert pattern?
The Arrange, Act, Assert (AAA) pattern is a common structure for writing unit tests. ‘Arrange’ sets up the test scenario, ‘Act’ performs the action being tested, and ‘Assert’ verifies the outcome.
How can I improve the performance and reliability of my unit tests?
To improve performance and reliability, make sure your tests are stateless, deterministic, and performant. This means each test should produce the same result every time it runs, regardless of external factors.