A Step-by-Step Unit Test Example for Beginners
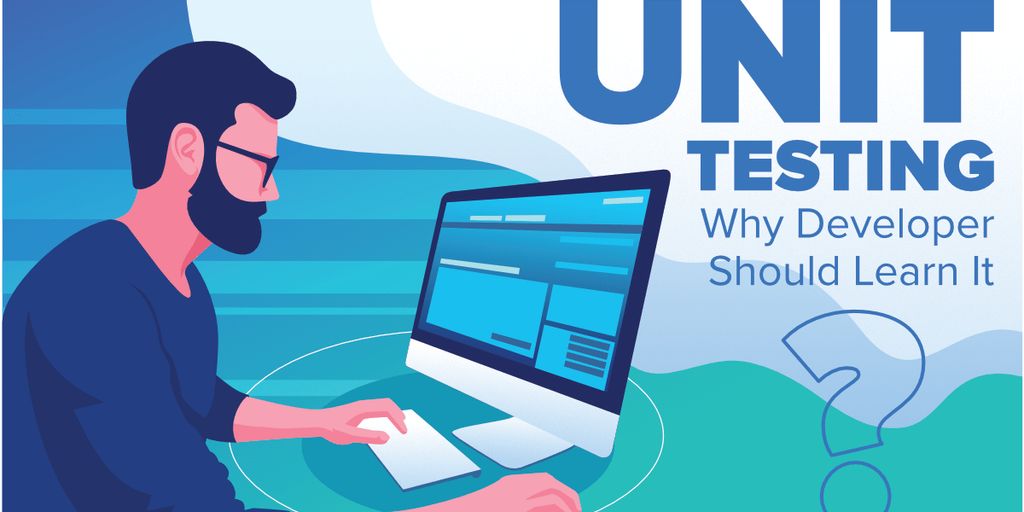
Unit testing is a crucial practice in software development that ensures individual units of code function as expected. This article provides a comprehensive, step-by-step guide to unit testing, aimed at beginners. Whether you’re new to testing or looking to solidify your understanding, this guide will walk you through the basics, setup, writing, and improving your tests, as well as exploring popular frameworks.
Key Takeaways
- Unit testing helps ensure that individual units of code work correctly, improving overall software quality.
- Setting up the right development environment is essential for effective unit testing.
- Writing your first unit test involves creating sample classes, writing test methods, and analyzing the results.
- Advanced techniques like mocking dependencies and parameterized tests can enhance your unit testing strategy.
- Continuous integration and maintaining test isolation are best practices for effective unit testing.
Understanding Unit Testing Basics
Unit testing is a type of software testing where individual units or components of a software are tested. The purpose is to validate that each unit of the software performs as designed. Unit testing is crucial for ensuring the quality and reliability of your codebase.
Definition and Purpose
Unit testing involves testing the smallest parts of an application, like functions or methods, in isolation from the rest of the application. The main goal is to ensure that each unit is working correctly. This type of testing is usually automated and helps in identifying bugs early in the development cycle.
Benefits of Unit Testing
Unit testing offers several benefits:
- Early Bug Detection: Identifies issues at an early stage, reducing the cost of fixing bugs.
- Code Quality: Improves the overall quality of the code by enforcing good design principles.
- Documentation: Acts as a form of documentation for the code, making it easier to understand.
- Refactoring: Facilitates safe refactoring by ensuring existing functionality is not broken.
Common Terminologies
- Test Case: A set of conditions or variables under which a tester determines whether a unit of software is working as intended.
- Test Suite: A collection of test cases intended to test a behavior or a set of behaviors of software programs.
- Mock Object: Simulated objects that mimic the behavior of real objects in controlled ways.
- Test Runner: A component that executes test cases and provides the results to the user.
Setting Up Your Development Environment
To get started with unit testing, it’s crucial to set up your development environment properly. This ensures that you have all the necessary tools and libraries to write, run, and analyze your tests effectively.
Writing Your First Unit Test
Now that we have finished setting up our project and its dependencies, we are now ready to write our unit test! You are now ready to write your first unit test! But where in your directory structure should your unit test reside? Obey the Maven naming conventions to ensure Maven is able to find your tests. That is, postfix your test class with “Test” and put the test files under: src/test/<package-path-to-class-under-test>
Advanced Unit Testing Techniques
Mocking is a technique used to simulate the behavior of complex, real objects in a controlled way. This is particularly useful when you need to test components that interact with external systems or services. By using mocks, you can isolate the unit under test and ensure that your tests are focused and reliable. Popular mocking frameworks include Mockito for Java, unittest.mock for Python, and Moq for .NET.
Parameterized tests allow you to run the same test logic with different inputs. This is useful for testing a variety of scenarios without writing multiple test cases. Frameworks like JUnit for Java and PyTest for Python support parameterized tests. Here’s a simple example in JUnit:
@RunWith(Parameterized.class)
public class ExampleTest {
@Parameterized.Parameters
public static Collection<Object[]> data() {
return Arrays.asList(new Object[][] { {1, 2, 3}, {4, 5, 9}, {7, 8, 15} });
}
private int a;
private int b;
private int expected;
public ExampleTest(int a, int b, int expected) {
this.a = a;
this.b = b;
this.expected = expected;
}
@Test
public void testAddition() {
assertEquals(expected, a + b);
}
}
Testing how your code handles exceptions is crucial for building robust applications. You can use unit tests to ensure that your methods throw the correct exceptions under various conditions. For example, in JUnit, you can use the expected
attribute of the @Test
annotation to specify the exception you expect:
@Test(expected = IllegalArgumentException.class)
public void testException() {
myObject.methodThatShouldThrowException();
}
By mastering these advanced techniques, you can significantly improve the quality and reliability of your unit tests.
Best Practices for Effective Unit Testing
Maintaining Test Isolation
One of the key principles of unit testing is to maintain test isolation. This means that each test should be independent and not rely on the state left by another test. This can be achieved by setting up the necessary preconditions before each test and cleaning up afterward.
Writing Readable Tests
Readable tests are crucial for maintaining and understanding your test suite. Use descriptive names for your test methods and organize your tests logically. Avoid complex logic within your tests; they should be straightforward and easy to follow.
Continuous Integration and Testing
Integrating your unit tests into a continuous integration (CI) pipeline ensures that tests are run automatically with every code change. This helps in catching issues early and maintaining code quality. Popular CI tools include Jenkins, Travis CI, and CircleCI.
Debugging and Improving Your Tests
Identifying Flaky Tests
Flaky tests are those that sometimes pass and sometimes fail without any changes to the code. These tests can be a significant source of frustration and can undermine the confidence in your test suite. When a test fails, you want to understand what went wrong. To identify flaky tests, you can:
- Run tests multiple times to see if they fail intermittently.
- Check for dependencies on external systems or shared states.
- Ensure that tests are isolated and do not interfere with each other.
Improving Test Coverage
Improving test coverage means ensuring that a larger portion of your codebase is tested. This can help in catching more bugs and ensuring the reliability of your software. One of the most powerful things about unit tests is that they provide excellent feedback on the design of your code. To improve test coverage:
- Identify untested parts of your code using coverage tools.
- Write tests for uncovered code paths.
- Refactor code to make it more testable if necessary.
Refactoring Tests
Refactoring tests is about making your tests more efficient and easier to understand. When a test becomes cumbersome, it might indicate a design problem in your code. To refactor tests:
- Simplify the setup logic to make it more readable.
- Remove any redundant or duplicate tests.
- Ensure that each test is focused on a single aspect of the code.
By following these steps, you can significantly enhance the reliability and maintainability of your test suite, making software testing a critical debugging tool in your development process.
Exploring Popular Unit Testing Frameworks
JUnit is one of the most popular unit testing frameworks for Java. It provides annotations to identify test methods and assertions to test expected results. JUnit’s simplicity and ease of use make it a favorite among Java developers. Here are some key features:
- Annotations for test lifecycle management
- Assertions for testing expected results
- Integration with IDEs and build tools
PyTest is a powerful testing framework for Python. It supports fixtures for managing test setup and teardown, and it can run tests in parallel. PyTest’s flexibility and rich plugin architecture make it suitable for both simple and complex testing needs. Key features include:
- Fixtures for setup and teardown
- Parameterized testing
- Rich plugin architecture
NUnit is a widely-used unit testing framework for .NET applications. It supports data-driven tests and has a strong assertion library. NUnit’s compatibility with various .NET languages and its extensive documentation make it a reliable choice for .NET developers. Key features include:
- Data-driven tests
- Strong assertion library
- Compatibility with multiple .NET languages
Conclusion
Unit testing is an indispensable practice for ensuring the reliability and functionality of your code. By following the step-by-step guide provided in this article, beginners can gain a solid foundation in writing and running unit tests. From setting up the testing framework to writing your first test and interpreting the results, each step is designed to build your confidence and skills. Remember, unit testing not only helps in catching bugs early but also makes your code more maintainable and robust. As you continue to practice and explore more advanced topics, you’ll find that unit testing becomes an integral part of your development workflow. Happy testing!
Frequently Asked Questions
What is unit testing?
Unit testing is a software testing method where individual units or components of a software are tested in isolation to ensure they work as expected.
Why is unit testing important?
Unit testing helps in identifying bugs early in the development process, improves code quality, and ensures that each component works correctly before integration.
What tools are required for unit testing in Java?
For unit testing in Java, you typically need a testing framework like JUnit, a build tool like Maven or Gradle, and an Integrated Development Environment (IDE) like IntelliJ IDEA or Eclipse.
How do you write a simple unit test in Java?
To write a simple unit test in Java, you create a test class, write test methods using assertions to check expected outcomes, and run the tests using a testing framework like JUnit.
What are mocks in unit testing?
Mocks are simulated objects that mimic the behavior of real objects in controlled ways. They are used in unit testing to isolate the unit being tested from its dependencies.
What is the difference between unit testing and integration testing?
Unit testing focuses on testing individual units or components in isolation, while integration testing focuses on testing the interactions between different units or components to ensure they work together as expected.