A Practical Unit Testing Sample for Beginners
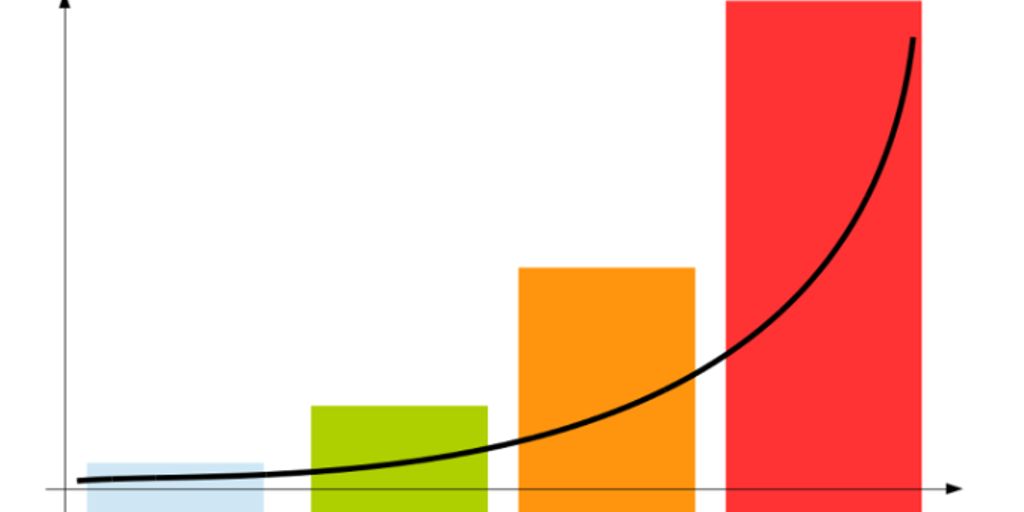
Unit testing is a way to make sure each small part of your code works as it should. For beginners, it’s best to start by testing simple utility classes. These classes usually don’t rely on other parts of the program and have clear inputs and outputs. In this article, we will guide you through the basics, setting up your environment, writing your first test, and best practices. By the end, you’ll know how to troubleshoot issues and integrate unit tests into your workflow.
Key Takeaways
- Start with simple utility classes for easy learning.
- Unit testing helps catch bugs early.
- Choose the right tools and set up your environment properly.
- Always write clear and focused test cases.
- Automate your tests for better efficiency.
Understanding the Basics of Unit Testing
What is Unit Testing?
Unit testing is a fundamental aspect of software testing where individual components or functions of a software application are tested in isolation. This helps ensure that each part works correctly on its own. By focusing on small, manageable pieces of code, developers can catch bugs early and fix them before they become bigger problems.
Why Unit Testing is Important
Unit testing offers several benefits:
- Early Bug Detection: Catch issues at the earliest stage of development.
- Code Quality: Improve the overall quality of the codebase.
- Simplified Refactoring: Make changes to the code with confidence, knowing that tests will catch any errors.
- Documentation: Serve as a form of documentation, showing how the code is supposed to work.
Common Terminologies in Unit Testing
Understanding the language of unit testing is crucial. Here are some key terms:
- Test Case: A set of conditions or variables used to determine if a unit of code works as expected.
- Test Suite: A collection of test cases that are intended to be used together.
- Mock Object: A simulated object that mimics the behavior of real objects in controlled ways.
- Assertion: A statement that checks if a condition is true, used to validate the outcome of a test.
By mastering these basics, you’ll be well on your way to becoming proficient in unit testing.
Setting Up Your Unit Testing Environment
Choosing the Right Tools
Selecting the appropriate tools for unit testing is crucial. The right tools can make your testing process smoother and more efficient. Popular choices include JUnit for Java, NUnit for .NET, and pytest for Python. Consider the language and framework you are using when making your selection.
Installing JUnit for Java
To get started with JUnit, you need to install it in your development environment. Follow these steps:
- Download the JUnit library from the official website.
- Add the JUnit library to your project’s build path.
- Ensure you have a compatible version of Java installed.
Configuring Your Project for Unit Testing
Once JUnit is installed, you need to configure your project to use it. This involves setting up the directory structure and build tools. Typically, unit tests are stored in a separate directory, such as src/test/java
for Maven projects. Make sure your build tool, like Maven or Gradle, is configured to include this directory in the test phase.
Writing Your First Unit Test
Creating a Simple Utility Class
If you are new to unit testing, start by writing tests for a utility class. Utility classes usually have simple input and output, making them easier to test. This approach helps you get comfortable with the basics of unit testing without dealing with complex dependencies.
Writing Test Cases with JUnit
To write test cases, you can use a unit test framework like JUnit. JUnit 5 is a popular choice for Java developers. It provides a structured way to create and run tests. You can follow a junit 5 tutorial to learn how to write unit tests with the JUnit 5 framework.
Running and Analyzing Your Tests
Once you’ve written your test cases, the next step is to run them. Most IDEs, like Eclipse, offer built-in tools to run and analyze your tests. When you run your tests, you’ll see which ones pass and which ones fail. This feedback is crucial for improving your code. Remember, unit tests should be written before any other type of test to ensure your code works as expected from the start.
Best Practices for Effective Unit Testing
Keeping Tests Simple and Focused
When writing unit tests, it’s crucial to keep them simple and focused. Each test should cover a single aspect of the code. This makes it easier to identify what went wrong if a test fails. Avoid writing complex tests that try to cover multiple scenarios at once.
Using Mocks and Stubs
Mocks and stubs are useful tools in unit testing. They help simulate the behavior of complex objects and systems, allowing you to test your code in isolation. Here are some tips for using mocks and stubs effectively:
- Use mocks to verify interactions between objects.
- Use stubs to provide predefined responses to method calls.
- Avoid overusing mocks and stubs, as they can make tests harder to understand.
Ensuring Tests are Repeatable
Tests should be repeatable and produce the same results every time they are run. This means avoiding dependencies on external systems or data that can change. To ensure repeatability:
- Use fixed data sets for testing.
- Avoid relying on real-time data or external services.
- Clean up any changes made during the test after it completes.
By following these best practices, you can create effective and reliable unit tests that help maintain the quality of your code.
Troubleshooting Common Unit Testing Issues
Dealing with Failing Tests
When a test fails, it can be frustrating. Start by checking the error message to understand what went wrong. Sometimes, the issue might be with the test itself rather than the code. Make sure your test cases are up-to-date and reflect the current state of your code. If you encounter persistent failures, consider revisiting your test logic and the assumptions you made.
Handling Dependencies
Dependencies can complicate unit testing. To manage them, use mocks and stubs to simulate the behavior of complex objects. This helps isolate the unit you’re testing and ensures that external factors don’t affect your results. Remember, the goal is to test a single unit of code in isolation.
Improving Test Coverage
Improving test coverage means ensuring that all parts of your code are tested. Use code coverage tools to identify untested areas. Aim for high coverage, but don’t obsess over it. Focus on critical parts of your application and make sure they are thoroughly tested. This will help you catch more bugs and improve the reliability of your software.
Integrating Unit Tests into Your Development Workflow
Automating Unit Tests
Automating unit tests is crucial for saving time and effort. By making unit test execution a part of your automated CI workflow, you’ll get feedback on code quality earlier in the development process when problems are faster, cheaper, and easier to fix. Automating unit testing through an established testing framework and process helps boost efficiency and collaboration in your team, leading to enhanced productivity. Building unit testing into your CI/CD pipeline also helps prevent regressions.
Continuous Integration and Unit Testing
Continuous Integration (CI) is a practice where developers frequently integrate their code into a shared repository. Each integration is verified by an automated build and tests. This practice helps catch issues early and ensures that the codebase remains stable. Integrating unit tests into your CI process means that every time code is committed, unit tests are run automatically, providing immediate feedback on any issues.
Monitoring and Reporting Test Results
Monitoring and reporting test results are essential for understanding the health of your codebase. Tools like Jenkins, Travis CI, or CircleCI can be used to automate the running of unit tests and generate reports. These reports help identify failing tests, track test coverage, and monitor trends over time. Regularly reviewing these reports can help you spot patterns and address recurring issues, leading to more robust and reliable code.
Advanced Unit Testing Techniques
Parameterized Tests
Parameterized tests allow you to run the same test with different inputs. This is useful when you want to test a function with multiple sets of data. By using parameterized tests, you can avoid writing repetitive code and make your tests more efficient. For example, if you have a function that adds two numbers, you can test it with various pairs of numbers without writing separate test cases for each pair.
Test-Driven Development (TDD)
Test-Driven Development (TDD) is a technique where you write tests before writing the actual code. This approach helps you think about the requirements and design of your code before implementation. The process usually involves writing a failing test, writing the minimum code to pass the test, and then refactoring the code. TDD can lead to better-designed, more maintainable code and can help catch bugs early in the development process.
Behavior-Driven Development (BDD)
Behavior-Driven Development (BDD) is similar to TDD but focuses more on the behavior of the application from the user’s perspective. In BDD, tests are written in a more natural language that describes the expected behavior of the application. This makes it easier for non-technical stakeholders to understand the tests and participate in the development process. BDD encourages collaboration between developers, testers, and business stakeholders to ensure that the software meets the user’s needs.
Conclusion
Unit testing might seem tricky at first, but with practice, it becomes easier. Start with simple utility classes and use tools like JUnit to help you. Remember, unit tests are there to make sure your code works as expected. As you get better, try to follow best practices to get the most out of your tests. Keep learning and testing, and soon it will be a natural part of your coding process. Happy testing!
Frequently Asked Questions
What is unit testing?
Unit testing is a way to check if small parts of your code, called units, work correctly. It helps make sure that each part works on its own.
Why is unit testing important?
Unit testing is important because it helps find problems early, makes your code better, and saves time in the long run.
What tools do I need for unit testing?
Some popular tools for unit testing include JUnit for Java, NUnit for .NET, and PyTest for Python. Choose the one that works best for your programming language.
How do I start with unit testing?
Start by writing tests for simple utility classes. These classes usually have simple inputs and outputs, making them easier to test.
What are mocks and stubs?
Mocks and stubs are fake objects used in unit testing to stand in for real objects. They help you test parts of your code without relying on external systems.
How can I improve my unit test coverage?
To improve test coverage, write tests for all possible cases, including edge cases. Make sure to test both common and uncommon scenarios.