Best Practices in Unit Testing: Testing for Robustness
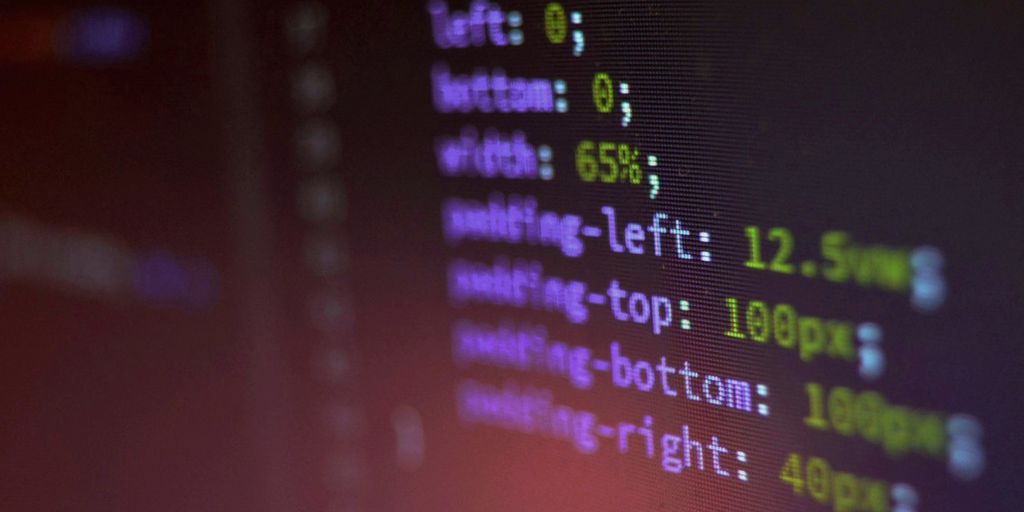
Unit testing is an essential aspect of software development that ensures each unit of code works as intended. By focusing on best practices in unit testing, developers can create robust and reliable software systems. This article explores various strategies and techniques to enhance the effectiveness of unit tests, covering everything from the basics of unit testing to advanced methodologies and tools.
Key Takeaways
- Understanding the core principles of unit testing is fundamental for building a solid testing framework.
- Designing comprehensive test cases that cover edge cases and boundary values is crucial for robust software.
- Utilizing mocks and stubs effectively can greatly enhance the isolation and accuracy of unit tests.
- Implementing Test-Driven Development (TDD) can lead to higher quality code and better designed software systems.
- Integrating continuous integration and leveraging advanced testing tools can significantly improve the scalability and performance of unit tests.
Understanding the Fundamentals of Unit Testing
Defining Unit Testing
Unit Testing is a process where individual units or components of a software are tested to validate that each unit performs as designed. This testing level focuses on the smallest part of the software design, which is often a function or a procedure in isolation from the rest of the application.
Core Principles
The core principles of unit testing include writing test cases that are independent of each other, covering all possible input scenarios, and ensuring that tests are repeatable and maintainable. Emphasizing these principles ensures that the software behaves as expected under various conditions.
The Importance of Isolation
Isolation is crucial in unit testing as it ensures that the tests are not affected by external factors or the state of other units. This approach helps in identifying and fixing defects more efficiently, leading to a more robust and reliable software product.
Designing Test Cases for Maximum Coverage
Identifying Edge Cases
To ensure comprehensive test coverage, identifying edge cases is crucial. These are conditions that occur at the extreme ends of the operating parameters, which may cause unexpected behaviors or failures. For example, testing how a software handles zero, negative values, or extremely high values can reveal vulnerabilities.
Using Equivalence Partitioning
Equivalence partitioning helps in reducing the number of test cases by grouping inputs that should be treated the same into ‘equivalence classes’. This method ensures that each partition is tested at least once, promoting efficient test coverage without redundancy.
Boundary Value Analysis
Boundary value analysis (BVA) is a technique used to identify errors at boundaries rather than within the ranges themselves. It involves testing at the edges of input ranges. For instance, if an input range is from 1 to 100, BVA would test at 0, 1, 100, and 101. This method is particularly effective in finding the maximum statement coverage.
Leveraging Mocks and Stubs
When to Use Mocks vs. Stubs
Mocks and stubs are both used to simulate the behavior of software components, but they serve different purposes. Use mocks to verify interactions between objects, ensuring that they communicate as expected during tests. Stubs, on the other hand, are best used to provide predetermined responses to calls made during the test, focusing more on state verification rather than interactions.
Best Practices for Mocking
To effectively use mocking in unit tests, follow these guidelines:
- Always isolate the unit being tested to ensure that tests are not affected by external dependencies.
- Use mocking frameworks that are well-documented and widely supported in the community.
- Keep mocks as simple as possible to avoid introducing complexity into tests.
Integrating Third-Party Tools
Incorporating third-party tools for mocking and stubbing can enhance testing efficiency and coverage. Tools like Mockito, Sinon, and WireMock offer advanced features that can handle various testing scenarios. Evaluate tools based on the following criteria:
- Ease of integration with existing test frameworks
- Support for different programming languages
- Availability of community support and documentation
Implementing Test-Driven Development (TDD)
The TDD Cycle
Test-Driven Development (TDD) is a software development approach where tests are written before the actual code. The cycle of TDD involves writing a test, seeing it fail, writing code to pass the test, and then refactoring the code. This cycle ensures that the code meets the requirements and works as expected from the very beginning.
Benefits of TDD
Implementing TDD can lead to higher quality software and better design decisions. It encourages developers to think through their design before coding, which can clarify requirements and reduce bugs. Additionally, TDD can improve the maintainability and extensibility of the codebase.
Common Pitfalls in TDD
While TDD offers numerous benefits, there are common pitfalls that developers should be aware of:
- Rushing through the cycle without proper refactoring
- Writing overly complex tests that are hard to maintain
- Not fully understanding the requirements leading to inadequate tests
Avoiding these pitfalls is crucial for leveraging the full potential of TDD.
Continuous Integration and Testing
Setting Up a CI Pipeline
Setting up a Continuous Integration (CI) pipeline is crucial for automating the testing process and ensuring that all code commits are verified automatically. Key steps include selecting a CI tool, configuring the build environment, and defining the build and test scripts.
Automating Unit Tests
Automating unit tests within a CI pipeline ensures that tests are run consistently and results are immediately available. It’s important to integrate unit tests into the build process so that any failures halt the build, prompting immediate attention.
Monitoring and Reporting Test Results
Effective monitoring and reporting of test results are essential for maintaining the health of the application. This involves setting up dashboards to track test success rates and configuring notifications to alert the team about test failures or performance issues.
Handling Exceptions and Errors in Tests
Writing Tests for Exception Handling
When writing unit tests, it’s crucial to include scenarios that might trigger exceptions in your application. This ensures that your software behaves as expected under adverse conditions. Testing for exceptions can prevent many issues from reaching production.
Asserting Exceptions
To effectively assert exceptions, use specific assertions that check for the type and message of the exception. This helps in verifying that your application responds correctly under error conditions. It’s important to have consistent error messages to make debugging easier.
Best Practices for Error Logging
Error logging within tests should be both informative and minimal to avoid clutter. Log only the essential information that would aid in troubleshooting. Here are some best practices:
- Include timestamp and error severity
- Provide context with error messages
- Use centralized logging for easier monitoring
Optimizing Test Performance and Scalability
Strategies for Speeding Up Tests
To enhance the efficiency of unit tests, consider parallel execution, reducing external dependencies, and optimizing setup and teardown processes. Implementing caching mechanisms can also significantly reduce test execution time.
Balancing Thoroughness and Performance
It’s crucial to strike a balance between comprehensive testing and maintaining swift performance. Prioritize critical paths in testing and use risk-based testing to focus on high-impact areas.
Scaling Tests with Your Application
As applications grow, tests must scale accordingly. Utilize modular testing frameworks and continuously refactor tests to improve maintainability and scalability. Implement load testing to assess the application’s performance under various levels of demand.
Advanced Techniques and Tools
Parameterized Testing
Parameterized testing allows developers to run the same test with different inputs, significantly enhancing test efficiency and coverage. This technique helps in identifying unexpected behaviors across a range of input values. It is particularly useful when dealing with complex algorithms or data-driven applications.
Using Code Coverage Tools
Code coverage tools measure the extent to which the source code is executed during testing. This metric helps in identifying untested parts of a codebase, ensuring that most, if not all, paths are covered. High code coverage, while not perfect, often correlates with higher software quality.
Exploring Mutation Testing
Mutation testing involves modifying certain parts of the source code to check if the tests can detect these changes. It’s a way to verify the effectiveness of the existing tests and can reveal necessary improvements in test cases. This method pushes the robustness of unit tests to new levels, making them more resilient against potential errors in code.
Conclusion
In conclusion, adhering to best practices in unit testing is crucial for developing robust software applications. By focusing on thorough test coverage, handling edge cases, and continuously refining testing strategies, developers can significantly enhance the reliability and maintainability of their code. Remember, effective unit testing not only catches errors early but also supports agile development processes, making it easier to adapt to changes and integrate new features. As technology evolves, so should our testing methodologies, ensuring that they remain effective in identifying potential issues and improving overall software quality.
Frequently Asked Questions
What is unit testing and why is it important?
Unit testing involves testing individual components of software to ensure they work as expected. It’s crucial for verifying the functionality of each part, ensuring quality, and facilitating easier maintenance and updates.
How can I identify edge cases in unit testing?
Identifying edge cases involves thinking critically about the inputs that could affect the system’s boundaries or unusual conditions. This might include extreme values, null values, or unexpected user behaviors.
What are mocks and stubs, and when should I use them?
Mocks and stubs are types of test doubles used to simulate the behavior of real components. Mocks are used for verifying interactions between objects, while stubs provide predetermined responses to calls made during the test.
What is Test-Driven Development (TDD)?
TDD is a software development approach where tests are written before writing the functional code. It helps ensure the code meets the required specifications from the start and can lead to cleaner, more error-free code.
How do I set up a Continuous Integration (CI) pipeline?
Setting up a CI pipeline involves configuring a system that automatically builds, tests, and sometimes deploys your software whenever changes are made. This usually requires selecting a CI tool, setting up a source code repository, and configuring build and test scripts.
What strategies can I use to optimize the performance of my unit tests?
To optimize test performance, consider running tests in parallel, reducing dependencies, mocking slow components, and focusing on critical paths. Balancing thoroughness with performance is key to maintaining both speed and quality.