Best Tools for Effective Unit Testing
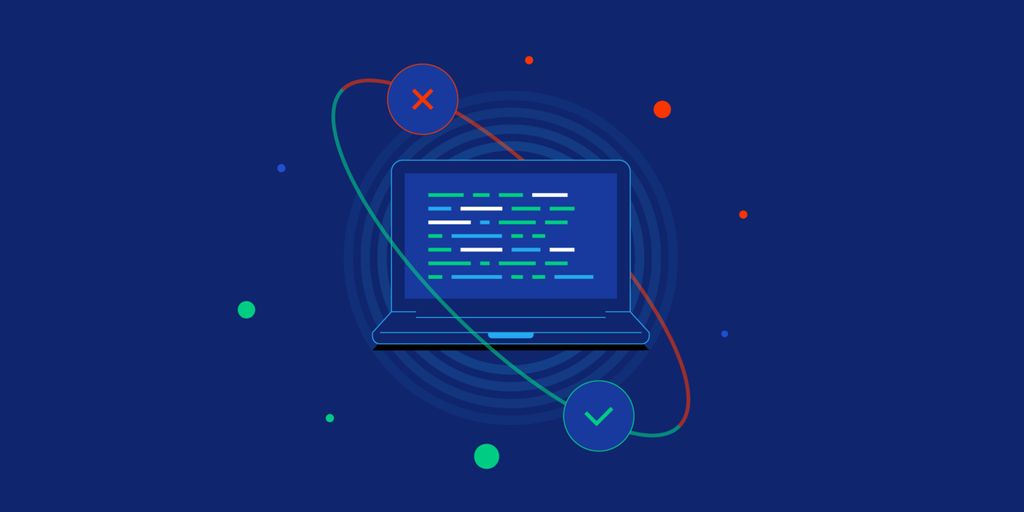
Unit testing is a crucial step in software development. It involves testing individual parts of your code to make sure they work correctly. Using the right tools can make this process easier and more effective. Here are some of the best tools for unit testing.
Key Takeaways
- JUnit is a popular tool for Java developers.
- NUnit is widely used for .NET applications.
- TestNG offers advanced features for testing in Java.
- Mocha is a great choice for JavaScript testing.
- PyTest is a favorite among Python developers.
1. JUnit
JUnit is a popular open-source unit testing framework for Java. It helps developers write and run repeatable tests. JUnit uses annotations like @Test to mark test methods and @Before and @After for setting up and tearing down test data.
Key Features:
- No need to create class objects or define the main method to run tests.
- Provides an assertion library to check test results.
- Widely adopted with strong community support.
- Seamlessly integrates with IDEs like Eclipse and IntelliJ IDEA.
Pros of JUnit:
- Boosts developer efficiency by ensuring code consistency.
- Reduces debugging time.
- Supports test-driven development, encouraging testing before coding.
2. NUnit
NUnit is an open-source unit testing framework specifically designed for .NET languages. It is similar to JUnit but tailored for the .NET environment. NUnit supports data-driven tests and can run tests in parallel, making it a powerful tool for developers.
Key Features
- Supports data-driven tests
- Allows parallel test execution
- Uses attributes like
[Test]
and[TestFixture]
for identifying tests and test classes - Provides a console runner for batch execution of automated tests
Pros of NUnit
- Strong parameterized testing capabilities
- Suitable for parallel test execution
- Robust assertion library
Cons of NUnit
- Limited to .NET languages
- May have a steeper learning curve for beginners
NUnit is a reliable choice for developers working within the .NET ecosystem, offering extensive support for test-driven development and a variety of features to streamline the testing process.
3. TestNG
TestNG, which stands for Test Next Generation, is a powerful testing framework that gives you great control over your tests. It combines features from both JUnit and NUnit, making it a versatile choice for developers. TestNG supports various types of tests, including unit, functional, and integration tests, which makes it one of the most effective tools for unit testing.
Key Features:
- Open-source: TestNG is free to use and is designed for the Java programming language.
- Concurrent Testing: It allows multiple tests to run at the same time, speeding up the testing process.
- Annotations: TestNG uses annotations to make test code easier to read and manage.
- Data-driven Testing: You can run the same test with different data sets, which is useful for checking how your code behaves under various conditions.
In summary, TestNG is a robust framework that enhances the testing experience by providing flexibility and powerful features. It is especially useful for developers looking to improve their unit testing practices.
4. Mocha
Mocha is a flexible JavaScript test framework that runs on Node.js and in the browser, making it easy to test asynchronous code. It supports both behavior-driven development (BDD) and test-driven development (TDD), along with other testing styles.
Key Features
- Wide range of plugins and integrations.
- Rich reporting and mapping exceptions to test cases.
- Easy asynchronous testing support.
Pros of Mocha
- Extensive support for generating meta-suites and test cases.
- Allows tests to run serially, which helps in mapping exceptions to the correct test cases.
- Suitable for both frontend and backend testing.
Cons of Mocha
- Requires assertion libraries (like Chai) for assertions, as it does not come bundled with one.
- Can be slow with large test suites.
For those looking to master Mocha, check out our [detailed guide on Mocha testing](https://example.com/mocha-js-tutorial).
5. Jasmine
Jasmine is a popular unit testing framework for JavaScript that follows behavior-driven development (BDD). It is a free tool that supports asynchronous testing and runs on any JavaScript-enabled platform. Jasmine is influenced by other unit testing frameworks but stands out because it does not require a Document Object Model (DOM) and has a simple syntax for writing test cases.
Key Features
- Supports asynchronous specifications
- Runs on any JavaScript-enabled platform
- Does not require DOM
- Simple syntax for writing test cases
Current Version
The current version of Jasmine is 2.4.1.
Official Link
For more information, visit the official Jasmine website.
6. Karma
Karma is an open-source testing framework designed to make testing JavaScript code in real browsers simple and efficient. It is particularly useful for testing front-end applications, especially those built with AngularJS. Karma’s test runner can start a web server, serve your JavaScript files, and run tests on real devices.
Key Features
- Supports test-driven development (TDD)
- Easily integrates with CI/CD tools like Jenkins, Travis, and Semaphore
- Facilitates easy debugging
- Automates regression testing
- Ideal for testing Angular applications
7. PyTest
PyTest is a popular unit testing tool for Python that stands out for its simplicity and power. It is a no-boilerplate alternative to Python’s standard unittest module. PyTest does not require classes for tests, supports parameterized testing, and can run unittest and nose test suites out of the box.
Pros of PyTest
- Simple syntax, making tests easy to write and read.
- Powerful fixtures system for managing test state.
- Extensive plugin system.
Cons of PyTest
- The learning curve for its advanced features.
- Sometimes slower than unittest for large test suites.
Use Case
Great for Python developers at all levels, offering powerful yet user-friendly features for a wide range of testing needs.
8. RSpec
RSpec is a popular testing tool for Ruby, designed to make test-driven development (TDD) easier and more efficient. It focuses on behavior-driven development (BDD), which encourages writing tests in a way that describes the behavior of the application.
Some best practices for using RSpec include:
- Focus on a single unit: Each test should target a specific part of the code.
- Use the arrange, act, assert pattern: This helps in structuring tests clearly.
- Write independent tests: Tests should not rely on each other to avoid cascading failures.
- Write clear and readable tests: This makes it easier for others to understand and maintain the tests.
By following these practices, you can ensure your tests are effective and maintainable.
9. PHPUnit
PHPUnit is a testing tool created with the PHP programming language. It was developed in 2001 and follows the xUnit architecture, similar to other unit testing frameworks like JUnit and NUnit. PHPUnit helps developers find hidden problems in new code by using assertions to check if the software unit gives the expected result.
Key Features
- Supports annotations to identify test methods.
- Provides setup and teardown methods.
- Offers logging and code coverage analysis.
- Results can be output in different formats like JUnit XML and TestDox.
Pros
- Widely used and well-documented.
- Supports data provider methods for data-driven tests.
- Integrates well with many PHP projects and frameworks.
Cons
- Runs via command line and cannot be run directly in the browser.
Writing Tests for PHPUnit
Unit tests are primarily written as a good practice to help developers identify and fix bugs, to refactor code, and to serve as documentation for a unit of work.
10. Mockito
Mockito is a popular unit testing framework for Java. It is mainly used to mock interfaces, allowing developers to add dummy functionalities for unit testing. Mockito simplifies the creation of mock objects by using the Java Reflection API.
Key Features:
- Methods like
verify()
andmock()
are extensively used in testing Java applications. - Supports annotation features for testing mocks.
- Mockito is powerful because it eliminates the need to write mock objects manually.
- Provides better readability for error messages.
- Supports safe refactoring, exception handling, and return value support.
Mockito records the interaction with mock objects and allows you to check if the mock object was used correctly, for example, if a certain method has been called on the mock.
Conclusion
In conclusion, choosing the right unit testing tool is essential for building reliable and maintainable software. Each tool has its own strengths and weaknesses, so it’s important to consider your project’s specific needs and your team’s expertise. Whether you opt for a free or paid tool, the goal remains the same: to ensure your code works as expected and to catch issues early in the development process. By investing time in selecting the right tool, you set your project up for success and make your development workflow smoother and more efficient.
Frequently Asked Questions
What is unit testing?
Unit testing is a way to check if small parts of your code, called units, work correctly. It helps find bugs early in the development process.
Why is unit testing important?
Unit testing is important because it ensures that each part of your code works as expected. This makes your software more reliable and easier to maintain.
What is JUnit used for?
JUnit is a unit testing tool for Java programming. It helps you write and run tests to make sure your Java code works as it should.
Can I use NUnit for C# projects?
Yes, NUnit is a popular unit testing tool for C# projects. It helps you test your C# code to ensure it behaves correctly.
How does TestNG differ from JUnit?
TestNG is similar to JUnit but offers more features like parallel testing and better test configuration. It’s also used for Java projects.
What is the role of Mockito in unit testing?
Mockito is a tool used to create mock objects in unit tests. This helps you test parts of your code in isolation by simulating the behavior of real objects.