Crafting Quality Software: A Guide to Unit Testing Test Cases
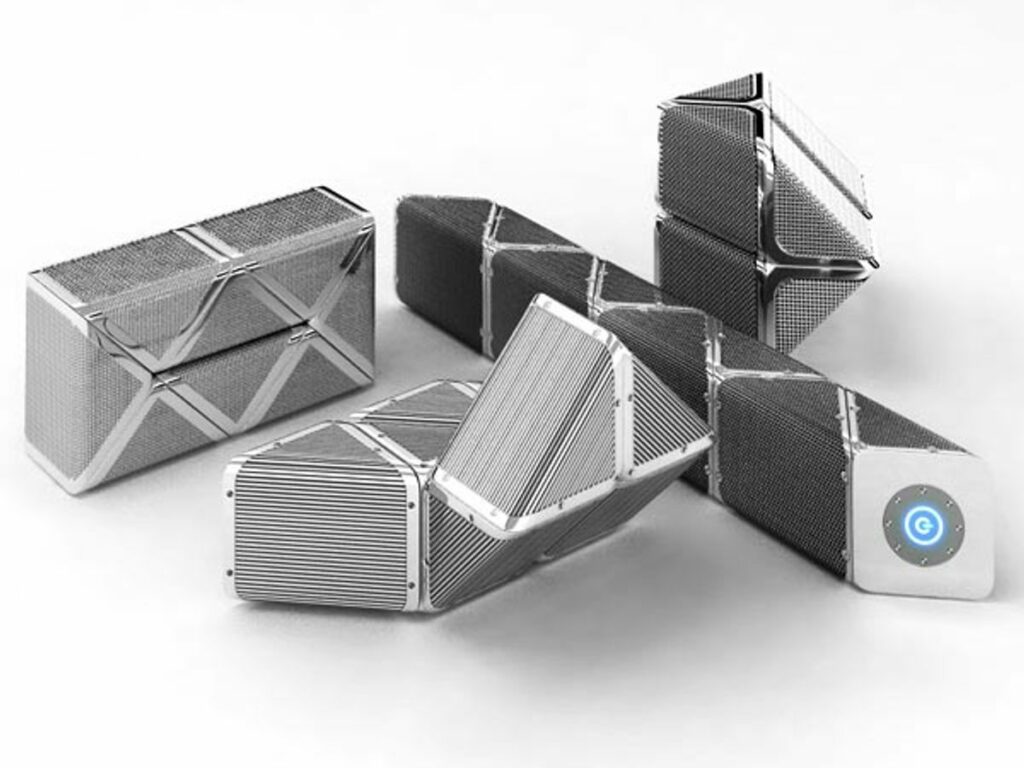
Unit testing is a fundamental aspect of software development that ensures the individual components of an application function correctly. This article serves as a comprehensive guide to unit testing, specifically focusing on test cases within the Laravel framework. It covers the basics of unit testing, effective test writing in Laravel, best practices, advanced techniques, and methods to enhance test case quality and reliability. By adhering to the principles and practices discussed, developers can build more stable and high-quality Laravel applications.
Key Takeaways
- Unit testing is an integral part of software development that enables early bug detection and contributes to the stability and reliability of applications.
- Laravel’s testing environment, complemented by PHPUnit, provides developers with the necessary tools to write meaningful and comprehensive unit tests.
- Adhering to best practices, such as ensuring test isolation and striving for meaningful code coverage, is crucial for maintaining high-quality software.
- Advanced unit testing techniques, including refactoring with confidence and leveraging mocks and stubs, play a significant role in the software development life cycle.
- Enhancing test case quality through methods like formal verification and fault injection can significantly improve the safety and reliability of the software.
Understanding the Fundamentals of Unit Testing
Defining Unit Testing in the Software Development Process
Unit testing is a fundamental practice in software development, focusing on the smallest parts of an application—individual components like classes, methods, and functions. Unit tests are designed to ensure that each code unit operates correctly in isolation, providing a safety net for developers as they build and maintain complex systems.
The importance of unit testing cannot be overstated. It allows developers to verify the correctness of their code early in the development process, which is crucial for identifying and resolving errors before they escalate. According to Guru99, unit testing is done during the development phase by the developers to isolate a section of code and verify its correctness. This practice aligns with the principles of Test-Driven Development (TDD), where tests are written before the code itself, following a red-green-refactor cycle.
By integrating unit testing into the development workflow, teams can achieve several benefits:
- Early bug detection, reducing the time and cost of later fixes
- Improved software design through modular and loosely coupled code
- Increased confidence in refactoring, as tests quickly identify regressions
Adopting unit testing is a step towards building better, more reliable applications. As Jeremy Holcombe notes, it’s a crucial component in ensuring that your application’s components work as expected in isolation.
The Role of Unit Testing in Early Bug Detection
Unit testing is a cornerstone of modern software development, serving as the first line of defense against bugs. By focusing on the smallest parts of an application, such as classes, methods, and functions, developers can ensure that each unit operates correctly in isolation. Early detection of bugs through unit testing is not just about finding faults; it’s about improving the overall design and maintainability of the code.
The benefits of unit testing in bug detection are manifold. Here are a few key points:
- Early bug detection reduces the cost and time associated with fixing issues later in the development cycle.
- It promotes better software design by encouraging developers to think about the interface and component interactions before diving into implementation.
- Unit testing provides a safety net that allows for confident refactoring, knowing that regressions will be quickly caught by existing tests.
Incorporating unit testing into the development workflow ensures that each piece of the codebase is scrutinized before it becomes part of the larger system. This practice not only catches bugs early but also contributes to a more robust and reliable application, as highlighted by automateNow’s insight into unit testing’s pivotal role in transitioning from bugs to brilliance.
Red-Green-Refactor: The TDD Approach
Test-driven development (TDD) is a methodical approach that reverses the traditional order of coding and testing. The core of TDD lies in the red-green-refactor cycle, a three-step process that ensures code quality and functionality from the outset. Initially, developers write a test for a new feature or improvement, which naturally fails (red) because the feature isn’t implemented yet. This is followed by writing the minimum amount of code required to pass the test (green). Finally, the new code is refactored to improve its structure and efficiency while keeping the test passing.
The benefits of TDD are multifaceted. It not only aids in early bug detection but also promotes better software design through modular and loosely coupled code. Moreover, TDD serves as a safety net for refactoring, allowing developers to enhance code without fear of breaking existing functionality. Here’s a brief rundown of the TDD cycle:
- Red phase: Write a failing test to define the desired functionality.
- Green phase: Implement just enough code to make the test pass.
- Refactor phase: Clean up the code while ensuring the test still passes.
As a result, test cases become a form of living documentation that is always synchronized with the codebase, providing clear examples of the code’s intended behavior.
Writing Effective Unit Tests in Laravel
Setting Up the Laravel Testing Environment
Once you’ve grasped the fundamentals of unit testing, setting up a Laravel testing environment is your next step. Laravel simplifies this process by supporting testing with PHPUnit and providing a suite of convenient helper methods. The framework comes with a preconfigured phpunit.xml file and a dedicated tests directory, ensuring you can dive straight into writing tests without the hassle of extensive configuration.
To prepare your Laravel application for unit testing, you’ll need to configure the testing database environment. Laravel’s approach to testing databases is both efficient and transient, using an in-memory SQLite database. Here’s how to set it up:
- Open the phpunit.xml file in your project’s root directory.
- Uncomment the lines that configure the database connection to SQLite and set the database to use memory.
Laravel’s default tests layout, with Feature and Unit subdirectories, provides a clear structure for organizing your tests. This organization is crucial for maintaining a clean testing environment and adhering to TDD principles. By writing tests before the actual code, you can ensure that each component, such as controllers and models, behaves as expected right from the start.
Crafting Meaningful Test Cases
Crafting meaningful test cases is a pivotal step in ensuring the quality and reliability of your Laravel application. A well-constructed test case not only verifies the correctness of a specific piece of functionality but also serves as a form of documentation for future reference. To achieve this, each test case should be clear, concise, and focused on a single aspect of the application.
When writing test cases, consider the following points:
- Define clear objectives for each test to understand what success looks like.
- Use descriptive test method names that convey the purpose of the test.
- Structure tests to reflect the application’s domain logic, making them easier to comprehend and maintain.
Remember, the goal is not to inflate the number of tests but to cover the critical and complex parts of your application thoroughly. As highlighted by Kinsta, don’t skip unit testing; it’s crucial in building better Laravel apps. By adhering to these principles, you can create a suite of tests that not only validate your application but also facilitate a smoother development process.
Utilizing PHPUnit for Robust Testing
PHPUnit is the backbone of testing in the PHP world, and Laravel’s integration with this framework is seamless. Laravel’s support for PHPUnit includes a set of convenient helper methods, simplifying the process of writing and running tests. With minimal configuration, developers can leverage a preconfigured phpunit.xml file and a dedicated tests directory, streamlining the setup process.
To effectively utilize PHPUnit in Laravel, it’s essential to understand the phpunit.xml file’s role. This configuration file orchestrates the testing process, ensuring consistency and allowing for customization to meet project-specific needs. It defines test suites, specifies the test environment, and sets up database connections, among other settings.
Here are some basic steps to get started with PHPUnit in Laravel:
- Install PHPUnit via Composer if it’s not already included in your Laravel project.
- Configure the phpunit.xml file to suit your application’s requirements.
- Write tests in the tests directory, using Laravel’s built-in methods and assertions.
- Run your tests using the
php artisan test
command or directly with PHPUnit.
By following these steps and embracing best practices, such as the red-green-refactor cycle, developers can create robust and high-quality applications. The integration of PHPUnit within Laravel’s ecosystem is a testament to the framework’s commitment to quality and reliability in software development.
Best Practices for Unit Test Cases
Ensuring Test Isolation and Independence
Unit testing hinges on the principle that each piece of code should be verified independently to ensure that individual units function as intended. This isolation is crucial for pinpointing the exact location of defects within the codebase.
To achieve test isolation, developers must structure their tests so that the outcome of one test does not affect another. This often involves creating separate test environments or using mock objects to simulate dependencies. Here are some key strategies to ensure test isolation:
- Utilize mocks and stubs to replace external dependencies.
- Reset the state before each test to prevent cross-contamination.
- Run tests in parallel to detect hidden dependencies.
By adhering to these practices, developers can maintain a suite of unit tests that accurately reflect the health of the code, free from interference caused by overlapping test scopes.
Achieving Meaningful Code Coverage
Code coverage is a metric that quantifies the extent to which our unit tests exercise the codebase. It’s a crucial aspect of ensuring that our tests are comprehensive and that they touch upon the various paths and conditions within our application. Higher code coverage is often associated with more thorough testing, but it’s essential to understand that it doesn’t inherently guarantee the effectiveness or completeness of tests.
When reviewing code coverage reports, it’s important to look beyond the percentages. For instance, a model with 100% coverage indicates that all methods and lines of code have been tested. However, the total coverage for the entire codebase might be significantly lower, revealing areas that may need more attention. Tools like PHPUnit and Laravel’s built-in features can generate these insightful reports, helping to identify parts of the codebase that are well-tested and those that might require additional test cases.
While striving for high code coverage, such as the often-cited 85% threshold, is commendable, it’s more critical to focus on the application’s most complex and crucial parts. This targeted approach ensures that the most important aspects of your application are robustly tested, rather than merely aiming for a high percentage across the board.
Maintaining Living Documentation Through Tests
Unit tests are not just a tool for checking the correctness of code; they also serve a crucial role as living documentation. This documentation is inherently up-to-date, as any changes in the code that lead to a discrepancy with the test cases will result in failing tests, prompting immediate updates. This ensures that the documentation evolves alongside the codebase, providing accurate guidance on how the code is intended to function.
Maintaining living documentation through tests offers several advantages. It enhances the confidence in refactoring, as developers can rely on the test suite to catch regressions. Moreover, it improves the overall readability and maintainability of the code, as the intent behind code changes is clearly communicated through the tests. Here’s a brief overview of the benefits:
- Confidence in refactoring: Tests identify regressions during code changes.
- Up-to-date documentation: Test cases reflect the current state of the code.
- Improved readability and maintainability: Tests clarify the expected behavior of the code.
By integrating test cases as part of the documentation, teams can ensure that both new and existing members can quickly understand and work with the code. This practice also facilitates better communication about the code’s functionality and expected behavior, making it easier for developers to collaborate and for new team members to get up to speed.
Advanced Techniques in Unit Testing
Refactoring with Confidence
Refactoring is a critical aspect of software development, aimed at improving the non-functional attributes of the codebase, such as its structure, readability, and performance. Unit tests play a pivotal role in this process, providing a safety net that allows developers to make changes with the assurance that existing functionality will remain intact. The presence of a comprehensive test suite means that any unintended side effects of refactoring are quickly caught, preventing regressions from slipping into production.
The Red-Green-Refactor cycle, central to Test-Driven Development (TDD), emphasizes the importance of having tests in place before refactoring. Initially, you write a failing unit test that captures the desired behavior. Once the test passes with the simplest code implementation, the refactor phase begins. Here, you can enhance the code’s quality, knowing that your tests will alert you to any issues. This cycle not only ensures early bug detection but also promotes an improved software design, encouraging developers to think about interfaces and component interactions upfront.
To illustrate the benefits of unit tests in the refactoring process, consider the following points:
- Early bug detection — TDD helps catch bugs early in the development process, reducing the cost and time of fixing problems later.
- Improved design — Encourages modular and loosely coupled code for better software design.
- Confidence in refactoring — Existing tests quickly identify any regressions introduced during refactoring.
In summary, unit tests are indispensable for refactoring with confidence. They serve as living documentation that is always up to date, guiding developers through code improvements while ensuring the system’s integrity is maintained.
Leveraging Mocks and Stubs
In the realm of unit testing, mocks and stubs play a crucial role in simulating the behavior of complex dependencies. These tools allow developers to create a controlled testing environment where the focus is on the unit under test, rather than the surrounding infrastructure. By using mocks and stubs, testers can ensure that the unit tests are not affected by external factors such as databases, network calls, or third-party services.
When integrating with APIs, mocking and stubbing become indispensable. They enable developers to test the interaction with an API without making actual network calls. This not only speeds up the testing process but also ensures that tests are not reliant on the availability or performance of external services. For instance, in API Integration Testing, mocking and stubbing can be used to replicate the responses from an API, allowing for thorough testing of the application’s handling of various scenarios.
Here are some benefits of using mocks and stubs in unit testing:
- Isolation from external dependencies
- Control over test scenarios
- Ability to test edge cases and error conditions
- Simplified testing of code that interacts with third-party services
It’s important to note that while mocks and stubs are powerful, they should be used judiciously. Over-reliance on these tools can lead to a false sense of security if they do not accurately represent the real-world behavior of dependencies.
Implementing Feature Testing Alongside Unit Tests
While unit tests are crucial for verifying the functionality of individual components, feature testing plays a complementary role by evaluating how these components interact within the larger application context. Feature testing is essential for ensuring that different parts of your application work together seamlessly, providing a more realistic assessment of the system’s behavior.
To implement feature testing effectively, follow these steps:
- Use the
php artisan make:test
command to create a new feature test case. - Write tests that simulate HTTP requests and responses to validate routes, controllers, and the integration of various components.
- Employ the
RefreshDatabase
trait to ensure a clean state for each test, preventing data pollution across tests. - Mimic user interactions to verify the user experience and functionality of the application as a whole.
Feature tests are particularly adept at uncovering issues that unit tests might miss, such as those affecting user journeys and complex scenarios. They also serve as a safeguard against regressions, helping to detect any code-breaking changes that may arise during development. By integrating feature testing into your testing strategy, you can achieve a more robust and reliable software delivery.
Enhancing Test Case Quality and Reliability
Researching Methods for Test Case Generation
In the quest for crafting quality software, researching methods for test case generation is pivotal. It involves exploring various strategies and algorithms to enhance the quality and coverage of the test cases produced. By incorporating domain knowledge and expert experience, developers can generate test cases that align more closely with real-world requirements.
The process of test case generation can be broken down into several key areas, each with its own set of methodologies and evaluation criteria. For instance, comprehensive test case generation methods are scrutinized based on specific datasets, metrics, and evaluation criteria to ensure they meet the desired standards of safety and reliability.
To further improve the generation quality, it’s essential to consider the following points:
- Research advanced generation strategies.
- Integrate domain knowledge into the generation model.
- Employ formal verification methods to ensure compliance with specifications.
- Utilize fault injection techniques to assess test case effectiveness.
Improving Safety with Formal Verification
Formal verification is a critical step in enhancing the safety and reliability of software systems. It involves the application of formal methods to prove the correctness of algorithms against their specifications. This rigorous process helps in identifying subtle bugs that traditional testing methods might miss.
The use of formal verification is particularly important in systems where failure can lead to catastrophic consequences, such as in automotive or aerospace software. Techniques like model checking, theorem proving, and abstract interpretation are employed to analyze and validate the software’s behavior. For instance, model checking can exhaustively explore all possible states of a system to ensure that certain properties hold.
While formal verification can significantly improve software safety, it is not without its challenges. It requires a deep understanding of mathematical logic and can be time-consuming. However, the benefits in terms of increased confidence in software correctness make it a worthwhile investment for critical systems.
Evaluating Test Effectiveness with Fault Injection
Fault injection is a powerful technique for assessing the robustness of unit tests. By deliberately introducing faults into the system, developers can simulate errors and observe how well their tests detect these issues. This method not only increases confidence in the software’s reliability but also highlights areas where test coverage may be lacking.
When implementing fault injection, it’s essential to measure the Fault Detection Rate (FDR). This metric indicates the success rate of tests in uncovering defects. Although a high FDR is desirable, it’s important to remember that it doesn’t guarantee all potential problems will be found. To complement this, Path Coverage should also be considered, ensuring that all execution paths are tested, thereby enhancing the thoroughness of the test suite.
Here’s a brief overview of key metrics to consider when evaluating test effectiveness:
- Fault Detection Rate (FDR): Measures the success of test cases in identifying defects.
- Path Coverage: Ensures all possible execution paths are covered by test cases.
By combining these metrics with fault injection techniques, developers can create more effective and reliable test cases, ultimately leading to safer and more robust software systems.
Conclusion
In conclusion, unit testing is an indispensable practice in crafting quality software, particularly in Laravel applications. By adhering to the principles of TDD and integrating PHPUnit into your development workflow, you can ensure that each component of your application functions as intended. This guide has highlighted the importance of writing meaningful test cases, the benefits of early bug detection, and the confidence that comes with the ability to refactor code without fear. Remember, while striving for high code coverage is commendable, the focus should always be on the thorough testing of critical and complex parts of your application. As you continue to build better Laravel apps, let unit testing be the cornerstone that upholds the integrity, reliability, and maintainability of your software.
Frequently Asked Questions
What is unit testing in software development?
Unit testing involves testing individual components of software, such as functions, methods, or classes, in isolation to ensure they work as expected. It’s a fundamental practice that helps detect bugs early in the development process.
How does Test-Driven Development (TDD) enhance software quality?
TDD involves writing tests before writing the code that makes the tests pass. This approach leads to better software design, early bug detection, and provides confidence when refactoring code.
Why is test isolation important in unit testing?
Test isolation ensures that each test case runs independently without side effects from other tests. This helps in identifying the cause of test failures and ensures the reliability of test results.
What is feature testing and how does it differ from unit testing?
Feature testing evaluates larger portions of the application, such as interactions between multiple components, while unit testing focuses on individual components in isolation.
How can you ensure high-quality test cases in Laravel?
In Laravel, you can ensure high-quality test cases by adhering to TDD principles, using PHPUnit for robust testing, and leveraging Laravel’s testing features like convenient methods and assertions.
What are some advanced techniques to improve unit testing?
Advanced techniques include refactoring with confidence due to existing tests, using mocks and stubs to simulate dependencies, and implementing feature testing alongside unit tests to cover more complex scenarios.