Creating the Optimal Test Environment for Reliable Results
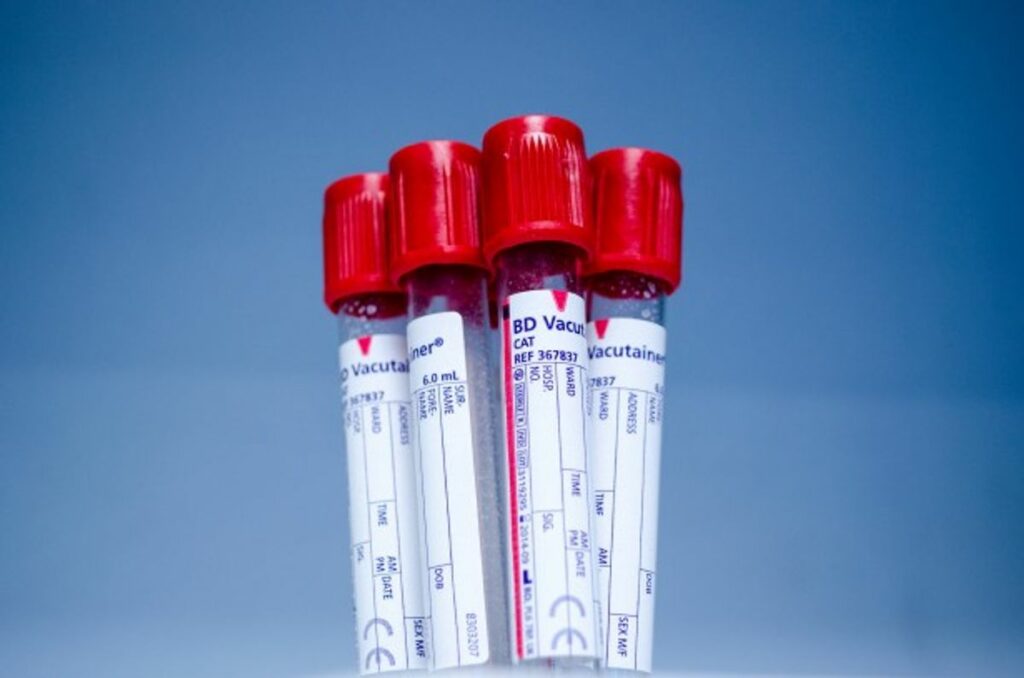
In the fast-paced world of software development, creating an optimal test environment is crucial for ensuring reliable results. This article explores the various strategies and practices that can be implemented to identify and mitigate flaky tests, write robust and dependable tests, and maintain a controlled and optimized testing process. By applying these methodologies, developers can enhance the stability and reliability of their test suites, which is essential for quality assurance throughout the software development lifecycle.
Key Takeaways
- Isolating flaky tests requires understanding their behavior under different conditions, employing strategies such as altering test order, mocking dependencies, and controlling timing with Jest’s timers.
- Writing reliable tests involves deterministic inputs and outputs, proper handling of asynchronous operations, and avoiding timing-based logic to ensure consistency.
- Process control and optimization are achieved through regular monitoring, applying Statistical Process Control (SPC), and ensuring the accuracy and consistency of testing equipment.
- Long-term reliability testing is essential, involving routine inspections, equipment calibration, stress testing, performance prediction, and effective data analysis and troubleshooting.
- Integration of testing into CI/CD pipelines and solving common problems like race conditions are key to creating realistic and reliable testing environments.
Understanding and Isolating Flaky Tests
Identifying the Causes of Flakiness
Flaky tests are a notorious issue in software development, often leading to a lack of confidence in the testing suite. These tests yield inconsistent outcomes, sometimes passing and other times failing, without any code changes. The unpredictability of flaky tests can result in overlooked bugs and wasted developer time, especially problematic in CI/CD pipelines where they can introduce delays and compromise software quality.
The causes of flakiness are varied, but common culprits include reliance on external services, timing issues, and improper asynchronous operations handling. For instance, in Jest testing environments, flakiness can arise from non-deterministic behaviors such as mocking the wrong selector, which can affect component rendering unpredictably.
To effectively address flaky tests, it’s crucial to understand their root causes. By isolating these tests and applying best practices, developers can improve the stability and reliability of their test suites, ensuring that automated testing continues to be a robust tool for quality assurance throughout the software development lifecycle.
Techniques for Isolating Unpredictable Tests
Isolating flaky tests is a critical step in ensuring the reliability of your test suite. By running tests under varied conditions, you can gain insights into their behavior and identify the root causes of unpredictability. One effective technique is altering the execution order of tests, which can reveal hidden dependencies or state contamination between tests.
Another approach involves mocking external dependencies to create a controlled test environment. This method helps to eliminate variability from external systems and allows for more consistent test results. Additionally, using tools like Jest’s timers can simulate different timing scenarios, helping to identify and resolve timing-based logic issues.
Here are some best practices to consider when isolating unpredictable tests:
- Run tests in a different order to detect inter-test dependencies.
- Mock external systems and dependencies to ensure consistent inputs.
- Utilize Jest’s fake timers to control and test timing-based logic.
- Implement custom scripts or use third-party tools to automate the detection of flakiness by running tests multiple times.
Implementing Jest’s Timers for Controlled Test Logic
In the quest to eliminate flakiness in tests, Jest’s fake timers stand out as a powerful tool. They allow developers to simulate the passage of time, making tests more predictable and less dependent on real-time delays. For instance, instead of waiting for setTimeout
to complete, which can slow down tests and introduce variability, Jest can simulate the timer’s completion instantly.
To effectively use Jest’s timers, it’s crucial to reset the state before each test. This practice ensures that tests are isolated and do not affect one another, maintaining a clean test environment. Additionally, addressing timing issues and race conditions is essential. By controlling JavaScript timers, we can prevent the flakiness caused by these asynchronous operations.
Here’s a simple example of how to implement Jest’s fake timers in a test:
test("reliable test with fake timers", () => {
jest.useFakeTimers();
setTimeout(() => {
expect(true).toBeTruthy();
}, 1000);
jest.runAllTimers(); // Simulates all timers running
});
By following these practices, developers can create more reliable and efficient tests, ensuring that the outcomes are consistent and independent of the environment’s timing.
Best Practices for Writing Reliable Tests
Deterministic Inputs and Outputs
Ensuring deterministic inputs and outputs is crucial for writing reliable tests. Flakiness often stems from tests that depend on variables which can change unpredictably between runs. For instance, a function like getRandomNumber
that yields a random number between 1 and 10 can introduce non-determinism into your tests due to its inherently unpredictable output.
To combat this, it’s essential to use techniques such as mocking or seeding random values to maintain consistency across test runs. Mocking external dependencies allows you to create a controlled test environment where inputs are stable and outputs are predictable. Here’s a good practice to follow:
- Mock or seed random functions to ensure output consistency
- Isolate tests from external data sources
- Use stubbing to simulate complex interactions
By adhering to these practices, you can significantly reduce the probabilistic nature of your test outcomes, leading to more reliable and maintainable test suites.
Proper Asynchronous Handling
Asynchronous operations in testing can introduce flakiness if not handled correctly. Proper asynchronous handling ensures that tests execute in a predictable manner, waiting for operations to complete before making assertions. A common pitfall is making assertions immediately after initiating an asynchronous operation, which can lead to false positives or unpredictable outcomes.
To write reliable asynchronous tests, follow these guidelines:
- Use
async
andawait
to ensure that the test waits for the asynchronous operation to complete. - Avoid fixed timers and instead use testing frameworks’ built-in mechanisms, like
waitFor
from the React Testing Library. - Structure your tests to reflect the actual execution order of asynchronous code.
By adhering to these practices, tests become more deterministic, reducing the likelihood of encountering flaky behavior due to improper handling of asynchronous operations.
Avoiding Timing-Based Logic
To achieve reliable test results, it’s crucial to avoid timing-based logic in your tests. Tests that depend on specific timing can be unpredictable, leading to flaky results. Instead of using real-time delays or system clocks, which can vary across different environments, consider using Jest’s fake timers to simulate time-based behavior.
For instance, rather than hard coding wait timers that introduce non-determinism, use Jest’s fake timers to control the flow of time in your tests. This ensures that your tests are not affected by the actual passage of time and can run quickly and consistently.
Here are some strategies to avoid timing-based logic in your tests:
- Utilize Jest’s fake timers to simulate delays and timeouts.
- Mock external services and APIs to prevent reliance on real-time responses.
- Reset the state before each test to ensure a consistent starting point.
- Synchronize asynchronous operations properly to avoid race conditions.
By following these strategies, you can create a test environment that is less susceptible to the variances of real-world timing, leading to more reliable and predictable test outcomes.
Process Control and Optimization in Test Environments
Regular Monitoring and Fine-Tuning
In the realm of software testing, regular monitoring is a pivotal activity that ensures the ongoing health and performance of applications. It encompasses the scrutiny of server health, application performance, user behavior, and error logs. This vigilant oversight allows teams to proactively detect and address issues, maintaining the software’s stability and responsiveness.
Optimization is the complementary process that enhances the software’s performance, efficiency, and user experience. It can range from refining code to optimizing database queries and improving UI/UX design. To illustrate the importance of these activities, consider the following points:
- Continuous monitoring identifies potential bottlenecks and performance issues.
- Optimization efforts lead to a more efficient and enjoyable user experience.
- Regular audits of the test suite can prevent the accumulation of flaky tests.
By integrating these practices into the development workflow, teams can sustain a high-quality software product, exceed user expectations, and remain competitive in a fast-paced industry.
Applying Statistical Process Control (SPC)
Statistical Process Control (SPC) is a methodical approach to monitoring and controlling a process to ensure that it operates at its full potential. By using SPC, teams can detect unwanted variability in the test environment and take corrective actions before defects occur. SPC is pivotal in maintaining consistent quality and efficiency in testing processes.
To effectively apply SPC, it is essential to understand the key metrics that indicate the health of the test environment. These metrics often include Process Capability Index (CPK), Yield, and Overall Equipment Efficiency (OEE). Below is a table summarizing how these metrics can be used to monitor process control:
Metric | Description | Relevance to SPC |
---|---|---|
CPK | Measures how closely a process is running to its specification limits | Indicates process capability |
Yield | The percentage of products that meet quality standards | Reflects the effectiveness of the process |
OEE | The ratio of fully productive time to planned production time | Assesses equipment productivity |
Regular application of SPC techniques, such as control charts and process capability analysis, can lead to significant improvements in test reliability. It allows for the early detection of variations and provides a framework for continuous process improvement. By integrating SPC into the test environment, organizations can achieve a more predictable and stable process, which is crucial for delivering reliable test results.
Ensuring Equipment Accuracy and Consistency
To achieve reliable results in testing environments, it is crucial to ensure that equipment operates with both accuracy and consistency. Regular calibration and maintenance are essential practices that underpin the precision of tools such as wafer probers and probe stations, which are used to evaluate the performance of each wafer.
The process of data collection and precision measurement is a critical component of quality control. It involves not only the accurate assessment of equipment performance but also the secure recording and reporting of data. This is where the principles of IQ (Installation Qualification), OQ (Operational Qualification), and PQ (Performance Qualification) come into play, particularly in FDA-regulated industries. These qualifications help define different user roles and ensure that the equipment adheres to the required standards.
Effective troubleshooting and data analysis are also vital for identifying and resolving any issues that may arise. This ensures that the equipment remains reliable over time, contributing to the overall integrity of the testing process. Below is a list of tools and software that support these efforts:
- Metrology and Defect Data Management
- Inspect Image Management
- Semiconductor Optical Memory Mapping
- Semiconductor Equipment Efficiency Monitoring Software
- Wafer Prober Control Module
- Semiconductor Failure Analysis Software
- Semiconductor Testing Software
- Semiconductor Yield Analysis Software
By integrating these tools and adhering to rigorous maintenance schedules, testing environments can maintain the high level of equipment accuracy and consistency required for dependable test outcomes.
Reliability Testing for Long-Term Performance
Routine Inspections and Equipment Calibration
Ensuring quality control through routine inspections and regular equipment calibration is fundamental for achieving reliable test results. These practices maintain the testing equipment’s accuracy and consistency, which are crucial for the integrity of the data collected. Tools such as wafer probers and probe stations are essential for accurately assessing semiconductor performance, and their precision must be upheld through meticulous calibration.
The integration of advanced testing methods, like the use of LabVIEW parametric test routines and Nikon Metrology’s optical technology, has led to significant improvements in testing efficiency. Automated, unattended wafer tests at various stages of the process flow highlight the importance of advanced equipment in research and development.
To ensure the effectiveness of routine inspections and equipment calibration, a structured approach is necessary. Below is a list of key components that should be regularly monitored and calibrated:
- Metrology and Defect Data Management
- Inspect Image Management
- Semiconductor Optical Memory Mapping
- Wafer Prober Control Module
- Semiconductor Failure Analysis Software
- Semiconductor Testing Software
- Semiconductor Yield Analysis Software
Regular calibration and inspection not only improve the reliability of test results but also contribute to the optimization of the manufacturing process, ensuring high standards of quality and efficiency.
Stress Testing and Performance Prediction
Stress testing is a critical component of ensuring long-term performance and reliability. By simulating high load or stress conditions, developers can identify potential bottlenecks and areas of improvement in the system. Performance prediction then takes these insights to forecast how the system will behave under similar conditions in the future, allowing for preemptive optimizations.
Key aspects of stress testing include:
- Assessing system behavior under peak load conditions
- Identifying the thresholds at which performance degrades
- Evaluating the system’s recovery process after failure
Performance prediction models leverage historical data to estimate future outcomes. These models are particularly useful in evolving digital landscapes where predictive analytics can identify bugs and performance issues more efficiently. The table below summarizes the relationship between stress testing metrics and performance predictions:
Stress Test Metric | Impact on Performance Prediction |
---|---|
Response Time | Directly correlates to user experience and system efficiency |
Throughput | Indicates the maximum load the system can handle |
Error Rate | Helps predict system reliability and maintenance needs |
By integrating stress testing and performance prediction into the development cycle, teams can ensure that their software is not only robust at launch but also primed for longevity and scalability.
Data Analysis and Troubleshooting Techniques
In the realm of semiconductor manufacturing, data analysis plays a pivotal role in ensuring the reliability of the production process. Advanced data interpretation methods are crucial for transforming extensive and complex wafer test data into actionable intelligence. Tools like yieldWerx offer powerful capabilities for data analysis, reporting, and interpretation, which are indispensable for identifying and rectifying yield issues.
The process of data analysis often begins with the collection of accurate and comprehensive data from each wafer test. This data includes electrical measurements, defect analysis, and more. Precision in these measurements is fundamental, as they form the basis for further analysis and decision-making. Automated systems like yieldWerxTM can streamline the collection and organization of data, enabling automated anomaly and trend detection that simplifies the analysis process.
Once data is collected, Commonality Analysis can be employed to identify patterns and correlations in failure data. This technique involves examining test results from various wafers to spot common failure trends or recurrent issues. By pinpointing these commonalities, engineers can trace back to the potential root causes of failures, which may stem from specific steps in the manufacturing process or particular batches of materials.
Integration and Problem-Solving in Testing Workflows
Incorporating Testing into CI/CD Pipelines
Incorporating testing into CI/CD pipelines is a critical step in ensuring that software changes are not only built and deployed efficiently but also meet quality standards. Automated testing within CI/CD workflows allows for immediate feedback on code changes, catching bugs early and reducing the risk of integration issues. This practice supports a culture of continuous improvement and rapid delivery, which is essential in today’s fast-paced software development environment.
To effectively integrate testing into CI/CD pipelines, consider the following steps:
- Define clear testing stages within the pipeline, such as unit testing, integration testing, and acceptance testing.
- Configure automated test execution triggered by code commits or pull requests.
- Utilize code review tools to ensure that tests are reviewed alongside the code.
- Set up notifications for test results to quickly address any failures.
- Regularly review and update test cases to align with new features and code changes.
By following these steps, teams can create a robust testing strategy that complements the CI/CD process, leading to more reliable and maintainable software.
Addressing and Resolving Race Conditions
Race conditions are a notorious source of flakiness in tests, often leading to unpredictable and unreliable results. Addressing and resolving race conditions is crucial for creating a stable test environment. The main hurdle is ensuring that operations, which are meant to run in parallel, do not interfere with each other, leading to inconsistent outcomes.
To combat this, a series of steps can be taken:
- Utilize Jest’s fake timers to control JavaScript timers, thus eliminating flakiness caused by timing issues.
- Mock external services and APIs to ensure tests do not rely on external factors.
- Reset the state before each test to prevent tests from affecting each other.
- Ensure proper synchronization of asynchronous operations to avoid the pitfalls of race conditions.
By systematically applying these techniques, developers can significantly reduce the occurrence of race conditions, leading to more reliable and consistent test results.
Creating Realistic and Reliable Testing Environments
To achieve real-world success, it’s essential to create testing environments that closely mimic production settings. This involves configuring the test environment to replicate the complexities and nuances of the actual user environment. Doing so ensures that tests are not only passing in an idealized setting but are also robust enough to handle the unpredictable nature of real-world operations.
Key components of a realistic testing environment include:
- Accurate simulation of network conditions
- Use of production-like data sets
- Integration of third-party services and APIs
- Implementation of security protocols
By addressing these areas, development teams and testers can interact more proficiently, leading to more reliable outcomes. Clear communication among all stakeholders is crucial to maintain the integrity of the testing process and to ensure that everyone is up to speed with the latest developments.
Conclusion
In conclusion, creating an optimal test environment is a multifaceted endeavor that requires attention to detail, a deep understanding of the tools at hand, and a commitment to best practices. From isolating flaky tests and controlling process parameters to ensuring equipment accuracy and handling asynchronous operations correctly, each aspect plays a critical role in achieving reliable results. By applying strategies such as mocking external dependencies, using Jest’s timers, and integrating with the manufacturing workflow, developers and engineers can enhance the stability and reliability of their test suites. This not only improves the quality assurance process but also ensures that products perform reliably in real-world conditions. Ultimately, the insights and techniques discussed in this article serve as a guide to fostering a testing environment where quality and efficiency are paramount, paving the way for successful software development and manufacturing outcomes.
Frequently Asked Questions
What are flaky tests and how can they be isolated?
Flaky tests are tests that exhibit inconsistent results, passing and failing across different runs without changes to code. Isolating flaky tests involves running them under varied conditions, altering the execution order, mocking external dependencies, and using Jest’s timers to control timing-based logic.
What are the best practices for writing reliable tests?
Best practices include ensuring deterministic inputs and outputs, proper handling of asynchronous operations using Jest’s async features, and avoiding timing-based logic by using Jest’s fake timers to simulate delays.
How does process control and optimization improve test environments?
Process control and optimization involve regular monitoring and fine-tuning of process parameters to maintain high quality and efficiency. Techniques like Statistical Process Control (SPC) provide a systematic approach to process monitoring, crucial for reliable test results.
Why are routine inspections and equipment calibration important in reliability testing?
Routine inspections and regular equipment calibration ensure the testing equipment’s accuracy and consistency. These practices are fundamental for obtaining reliable test results, as they help maintain quality control throughout the testing process.
How can integration and problem-solving in testing workflows be achieved?
Integration and problem-solving can be achieved by incorporating testing into CI/CD pipelines, addressing and resolving race conditions, and creating realistic and reliable testing environments that closely mimic production settings.
What are race conditions and how can they be resolved?
Race conditions occur when the outcome of a test depends on the sequence or timing of uncontrollable events, such as API calls or database operations. They can be resolved by ensuring proper handling of asynchronous operations and avoiding dependencies on timing or sequence in test logic.