Demystifying Unit Testing: Uncovering Its Purpose and Importance
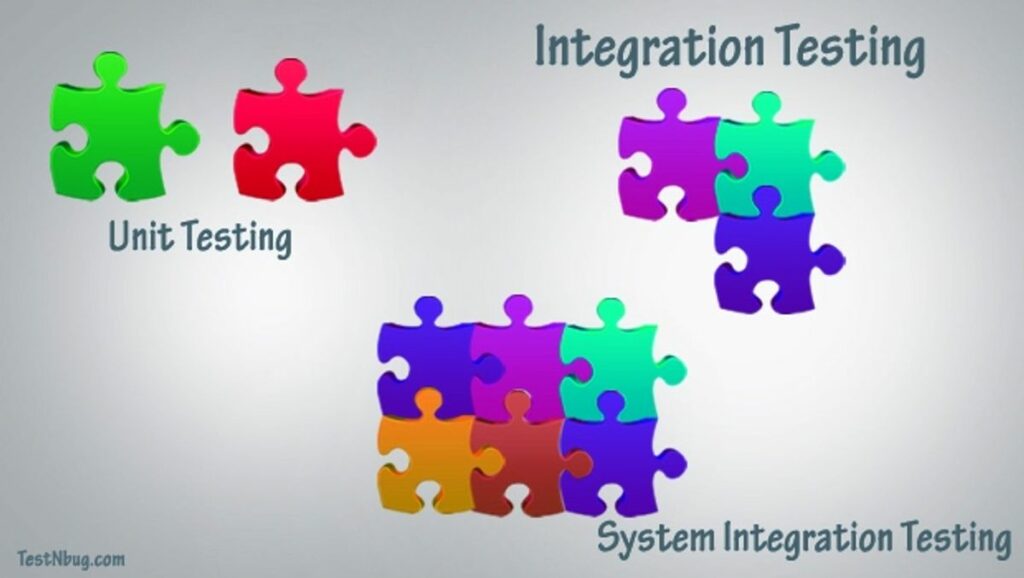
Unit testing is an essential practice in software development, enabling developers to verify the functionality of individual code units in isolation. By understanding and applying unit testing effectively, teams can ensure their applications are reliable and maintainable. This article dives into the fundamentals of unit testing, explores the role of mock testing, discusses strategies for implementation, assesses test quality, and examines advanced techniques and tools to demystify the process and highlight its significance.
Key Takeaways
- Unit testing breaks down complex applications into testable pieces, ensuring each part functions correctly before integrating into the larger system.
- Mock testing allows developers to simulate dependencies and isolate code for testing, enabling a focus on the unit’s behavior rather than external services.
- Incorporating unit testing into development processes like TDD and CI improves code quality and helps catch issues early in the development cycle.
- Quality assessment through assert and verify statements, along with code coverage metrics, is crucial for maintaining effective and reliable unit tests.
- Advanced techniques such as automated testing methodologies, mutation testing, and fuzzing, along with the right tools, can enhance the robustness of test suites.
Understanding the Fundamentals of Unit Testing
Defining Unit Testing and Its Scope
Unit testing is a fundamental practice in software development, where individual components of the application are tested to ensure they work as intended. Unit tests are designed to validate that each unit of the software performs correctly. Typically, a unit refers to the smallest testable part of an application, such as a function or a method.
The scope of unit testing is confined to the boundaries of a single component, without considering its interactions with other parts of the system. This isolation helps developers to identify and fix defects at an early stage, making it a crucial part of the coding phase. By focusing on small, manageable chunks, unit tests can be automated and executed quickly, providing immediate feedback to developers.
Here’s a brief comparison of different types of automated testing:
- Unit tests: Test individual units of code independently.
- Mock tests: Simulate internal or external services to test code in isolation.
- Integration tests: Verify the interfaces between components.
- System tests: Assess the system as a whole.
Unit testing is not only about verifying correctness but also about designing robust and maintainable code. By writing tests for small units, developers are encouraged to create modular and decoupled code, which is easier to manage and evolve over time.
The Anatomy of a Unit Test
At the core of unit testing lies the principle of breaking down the code into small, discrete parts, or units. This segmentation allows for each unit to be tested in isolation, ensuring that individual functions perform as expected without interference from other components.
A typical unit test comprises several key elements:
- Test case: The fundamental component that contains the code to execute and validate a single unit of functionality.
- Test suite: A collection of test cases, often related by the functionality they test or by the system component they belong to.
- Assertions: Statements that check if the code under test behaves as intended, comparing expected outcomes with actual results.
- Test fixtures: Any necessary setup or cleanup operations required before or after test execution, such as initializing test data or resources.
Executing these tests frequently during development can catch errors early and facilitate a smoother coding process. By automating these tests, developers can run them quickly and continuously, even while other code is being worked on. The goal is to parse unit tests into the smallest pieces possible, enhancing the precision and effectiveness of the testing process.
Best Practices for Writing Effective Unit Tests
Unit testing is a critical component of software development, ensuring that individual units of code function as intended. Adhering to best practices can significantly enhance the quality and reliability of these tests. One fundamental practice is to name your tests clearly and descriptively, which aids in identifying the purpose of the test and the functionality it covers. Tests should be autonomous, meaning they do not rely on external states or the outcomes of other tests. This independence is crucial for pinpointing issues quickly when a test fails.
Writing readable unit tests is another cornerstone of effective testing. Readability not only makes tests easier to understand but also facilitates maintenance and updates by other team members. It’s also important to embrace automated unit testing; automation speeds up the testing process and allows for more frequent test execution, which is especially beneficial when integrated into a continuous integration pipeline. Writing tests during the development process, rather than as an afterthought, ensures that testing is a priority and not overlooked.
Here are some additional best practices to consider:
- Use Assert and Verify in appropriate scenarios to check conditions within your tests.
- Avoid code duplication by creating reusable components or wrapping common functionality.
- Leverage parallel testing to run multiple tests simultaneously, reducing overall test execution time.
- Implement a uniform directory structure to keep your tests organized and manageable.
By incorporating these practices, developers can create a robust unit testing framework that contributes to the overall stability and performance of the software.
The Role of Mock Testing in Unit Testing
Simulating Dependencies with Mocks
Mock testing is an essential technique in unit testing that allows developers to simulate dependencies and isolate the unit of code under test. By creating mock objects that mimic the behavior of real services or components, developers can ensure that tests run in a controlled environment. This isolation is crucial for pinpointing issues and verifying that the unit behaves as expected without interference from external systems.
Mock tests are not just about creating a fake version of a service; they are about gaining certainty in the behavior of the unit being tested. Any variables external to the mock, such as values from an SDK, should be firmly controlled to prevent inconsistencies. While mock testing focuses on the individual unit, it’s important to understand its distinction from integration and end-to-end tests, which assess the interaction between multiple units and are inherently more complex.
To effectively implement mock testing, developers often rely on frameworks like Mockito and JUnit. These tools provide the necessary functionalities to stub and mock dependencies, allowing for comprehensive and reliable unit tests. For instance, using a mock with the Jest test runner can simplify testing with the React Web SDK, as seen in the Jest mock GitHub repo. However, it’s important to note that not all SDKs support mock testing, and developers may need to configure test data sources to achieve predictable behavior when evaluating flags.
Balancing Mock Tests with Real-World Scenarios
While mock tests offer a controlled environment to focus on individual units of code, it’s crucial to balance them with tests that reflect real-world scenarios. Mock tests should not become a crutch that prevents the code from being validated against actual behaviors and integrations.
To ensure a well-rounded testing strategy, consider the following points:
- Use mock tests to isolate and test specific functionalities without external dependencies.
- Gradually introduce integration tests to evaluate how units work together with real components.
- Perform end-to-end tests to assess the system as a whole, ensuring that all parts interact correctly in a production-like environment.
Remember, the goal of unit testing is not only to verify the correctness of code but also to ensure that it behaves as expected in the real world. Striking the right balance between mock tests and tests involving real components is essential for a robust testing strategy.
Integrating Mock Testing with Test Frameworks
Integrating mock testing within test frameworks streamlines the process of simulating dependencies, allowing developers to focus on the code under test. Frameworks like Jest provide specialized mocks for various services, which can be used with their respective SDKs or adapted for use with other JavaScript frameworks. This flexibility is crucial for maintaining the isolation of unit tests while ensuring that external variables are tightly controlled.
Incorporating mock testing into a test framework often involves a few key steps:
- Identifying the external dependencies that need to be mocked.
- Creating mock implementations or using existing ones from libraries or repositories.
- Configuring the test framework to use the mocks during the test execution.
This integration is essential for achieving a balance between unit testing and real-world scenarios, as it allows for the testing of code in isolation without the overhead of setting up actual services. Moreover, it supports automated testing methodologies, which are vital for continuous integration and delivery pipelines.
Strategies for Implementing Unit Testing in Development
Test-Driven Development (TDD) vs. Behavior-Driven Development (BDD)
When exploring the methodologies of Test-Driven Development (TDD) and Behavior-Driven Development (BDD), it’s crucial to understand their distinct approaches to software development. TDD focuses on the developer’s perspective, where tests are written before the actual code, ensuring that each piece of code is tested as soon as it’s written. BDD, on the other hand, is an agile-based development process that emphasizes collaboration between developers, QA, and non-technical stakeholders. This approach encourages the creation of tests that describe an application’s expected behavior from the users’ perspective.
BDD’s emphasis on business and feature specifications leads to tests with an improved shelf-life, as changes in business requirements often require minimal updates to the tests. This contrasts with TDD, where tests are closely tied to the structure of the code itself. BDD frameworks like Cucumber, Behave, and SpecFlow utilize a language called Gherkin, allowing tests to be written in plain English, which is accessible to all team members, regardless of technical expertise.
The choice between TDD and BDD can significantly impact the development process and product quality. Below is a comparison of key aspects:
- TDD:
- Developer-centric
- Tests guide code design
- Immediate feedback on code functionality
- BDD:
- Collaboration between technical and non-technical stakeholders
- Tests based on user behavior and business outcomes
- More adaptable to changes in business specifications
Incorporating Unit Testing into Continuous Integration
Incorporating unit testing into continuous integration (CI) systems is a critical step in ensuring that code changes are verified automatically and consistently. CI pipelines are designed to detect problems early, triggering a suite of unit tests with every code commit. This practice helps in maintaining code quality and stability throughout the development lifecycle.
To effectively integrate unit testing into CI, developers should follow these steps:
- Configure the CI server to run unit tests automatically upon every commit or pull request.
- Ensure that the unit test environment on the CI server matches the local development environment to avoid discrepancies.
- Manage test data and dependencies to ensure that unit tests are repeatable and reliable.
- Set up notifications for test results to quickly address any failures.
By adhering to these steps, teams can create a robust development process where unit tests serve as a first line of defense against bugs and regressions. It’s also important to maintain a balance between the speed of the CI process and the thoroughness of the testing to avoid bottlenecks while still catching critical issues.
Managing Unit Test Cases and Test Suites
Effective management of unit test cases and test suites is crucial for maintaining a robust testing framework. Naming conventions play a vital role in ensuring that tests are easily identifiable and understandable by all team members. It’s important to adopt a clear and descriptive naming strategy for both test cases and test suites to facilitate collaboration and future maintenance.
Running tests is a straightforward process, often initiated with a simple click in the development environment. For instance, in JetBrains Rider, you can run all tests in a class by clicking the unit test icon and selecting ‘Run All’. The results are then displayed, allowing you to quickly identify and focus on any failed tests.
Autonomy in test design is essential to avoid inter-dependencies that can lead to unreliable results. Each test should be self-contained and not rely on the outcome of another. This ensures that the test suite’s reliability is not compromised over successive test cycles. Additionally, performance testing should be integrated into the suite to assess the impact of the code under test on application performance.
Assessing the Quality of Your Unit Tests
Utilizing Assert and Verify in Tests
In the realm of unit testing, the use of assert and verify constructs is pivotal to validating the behavior of individual units of code. Asserts are typically employed when a test must be halted upon encountering a failure that would render subsequent tests invalid. For instance, if a login function fails to operate as expected, an assert would prevent any further tests that depend on a successful login from running, thus avoiding a cascade of failures.
On the other hand, verify is used in scenarios where test execution should continue despite failures. This approach is beneficial when the failure is not critical to the overall test suite, allowing for a broader assessment of the system’s behavior. By utilizing verify, we can assert that specific method calls have occurred, verify the order of method invocations, and even validate the arguments passed to them.
It’s essential to understand when to use each construct to ensure tests are both robust and informative. Below is a list of scenarios where assert and verify are appropriately applied:
- Use assert to halt execution on critical failures.
- Employ verify when test execution should continue after non-critical failures.
- Assert is ideal for validating mandatory conditions, while verify is suited for optional checks.
- Verify allows for a more comprehensive test run, capturing all failures for later analysis.
Measuring Code Coverage and Test Effectiveness
Measuring code coverage is essential in understanding how much of the source code is exercised by the unit tests. Code coverage metrics provide a quantitative basis for assessing the thoroughness of the testing process. However, high coverage does not necessarily equate to effective testing; it is possible to achieve high coverage with superficial tests that do not assert meaningful behavior or catch potential bugs.
To evaluate test effectiveness, developers often look beyond coverage percentages to the quality of the assertions within the tests. Effective unit tests are characterized by their ability to detect defects and to validate the correctness of the code under test. This involves a careful examination of the test cases to ensure they are comprehensive and sensitive to code changes. The following table illustrates common code coverage criteria and their descriptions:
Coverage Type | Description |
---|---|
Statement Coverage | Ensures every statement in the code has been executed. |
Branch Coverage | Verifies that each branch of conditional logic has been taken. |
Path Coverage | Checks that all possible paths through the code have been followed. |
Function Coverage | Confirms that all functions or methods have been called. |
Ultimately, the goal is to balance high code coverage with high-quality test cases that provide confidence in the software’s reliability and robustness.
Refactoring and Maintaining Unit Tests
Refactoring code is a critical aspect of maintaining a healthy codebase, and it’s equally important to ensure that unit tests are updated to reflect these changes. When a refactored code is being tested, all these scenarios need to be validated, along with regression testing of the impacted areas. Be proactive while refactoring to avoid introducing new bugs.
Naming conventions play a significant role in the maintainability of unit tests. It’s advisable to name the test cases and test suites appropriately to provide clarity on their purpose. This practice is especially beneficial when revisiting tests after an extended period or when collaborating with other team members.
Unit tests should be as granular as possible, targeting individual functions or methods. This approach not only facilitates easier testing and debugging but also ensures that feature flags or other changes in the application’s functionality do not interfere with the tests. Here’s a simple breakdown of the types of tests:
- Unit tests: Test individual units of code independently.
- Mock tests: Simulate internal or external dependencies to test code in isolation.
Advanced Unit Testing Techniques and Tools
Leveraging Automated Testing Methodologies
Automated testing methodologies are pivotal in enhancing the efficiency and effectiveness of unit testing. These methodologies streamline the testing process, making it faster and more reliable. They include a variety of techniques, such as mutation testing and fuzzing, which are instrumental in evaluating the robustness of test suites.
Incorporating automated testing into the development workflow can significantly improve the stability and scalability of test cases. For instance, using design patterns like the Page Object Model (POM) in Selenium test automation can lead to more maintainable and flexible tests. Moreover, automated test reports provide valuable insights into feature analysis and test coverage, ensuring that no aspect of the application goes unchecked.
To maximize the benefits of automated testing, it is essential to adhere to best practices. These include using a Behavior-Driven Development (BDD) framework, maintaining a uniform directory structure, and employing data-driven testing for parameterization. Avoiding code duplication and leveraging parallel testing are also key strategies that contribute to the success of automated testing efforts.
Exploring Mutation Testing and Fuzzing
Mutation testing is a rigorous approach to evaluating the quality of software tests by introducing deliberate faults, or ‘mutations,’ into the codebase. The goal is to ensure that the existing test suite is capable of catching these induced errors, thereby confirming the suite’s effectiveness. Fuzzing, on the other hand, is an automated technique that involves providing invalid or unexpected inputs to a program. The purpose of fuzzing is to uncover potential vulnerabilities and bugs that might not be detected through conventional testing methods.
Both mutation testing and fuzzing are critical for achieving high-quality software. By simulating faults and unexpected scenarios, developers can identify weaknesses in their code before it reaches production. This proactive approach to testing can lead to more robust and secure applications. Moreover, the integration of Large Language Models (LLMs) has enhanced these methodologies, allowing for more sophisticated test case generation and improved test coverage.
When selecting between mutation testing and fuzzing, it’s important to consider the specific needs of the project. Mutation testing is particularly useful for assessing the thoroughness of test cases, while fuzzing excels at discovering edge cases and security flaws. Combining both methods can provide a comprehensive testing strategy that maximizes the detection of potential issues.
Selecting the Right Tools for Different Testing Scenarios
Selecting the appropriate tools for unit testing is crucial to the success of the testing process. Different testing scenarios require different tools, each with its own strengths and ideal use cases. For instance, Selenium and Appium are widely used for web and mobile testing respectively, while JUnit and TestNG are popular choices for Java unit tests.
When choosing a tool, consider factors such as the programming language in use, the type of application under test, and the specific requirements of the testing scenario. Below is a list of common tools categorized by their primary use:
- Web Testing: Selenium, QTP/UFT
- Mobile Testing: Appium, Robot Framework
- API Testing: Postman, SoapUI
- Load Testing: JMeter, LoadRunner
- Unit Testing Frameworks: JUnit, TestNG
It’s also important to integrate the selected tools with other systems like continuous integration pipelines and test management software. Tools like Jenkins and JIRA can help streamline the testing process, ensuring that tests are run automatically and results are tracked efficiently. Ultimately, the right tool not only provides the necessary functionality but also fits seamlessly into the development workflow.
Conclusion
Throughout this article, we’ve explored the intricacies of unit testing, from its fundamental concepts to its critical role in software development. Unit testing serves as the backbone of a robust testing strategy, ensuring that each component of an application functions as intended. By implementing unit tests, developers can catch bugs early, facilitate code refactoring, and enhance code reliability. Moreover, the integration of mock tests and the use of automated testing methodologies like Selenium further augment the testing process, allowing for more comprehensive and efficient quality assurance. As we’ve seen, the choice between manual and automated testing, as well as the selection of specific testing types, should be tailored to the project’s needs. Ultimately, unit testing is not just a technical necessity but a practice that aligns business and technical objectives, leading to improved product quality and a more agile development cycle. Embracing unit testing is, therefore, an investment in the maintainability, scalability, and success of software projects.
Frequently Asked Questions
What is unit testing and why is it important?
Unit testing involves testing individual units of code to ensure they work correctly. It is important because it helps identify bugs early in the development process, making them easier and less costly to fix. It also supports code quality, maintainability, and can contribute to a more reliable software product.
How do mock tests fit into unit testing?
Mock tests are a type of unit test that simulate internal or external services to isolate the behavior of the code being tested. This allows developers to focus on the correctness of the code without the interference of external dependencies.
What’s the difference between Test-Driven Development (TDD) and Behavior-Driven Development (BDD)?
TDD focuses on writing tests before code to define the desired functionality, while BDD emphasizes collaboration between technical and non-technical stakeholders to create tests based on business and feature specifications. BDD tests are often more understandable and maintainable due to their business-centric approach.
What role do assert and verify play in unit testing?
Assert and verify are used in unit testing to check if the code behaves as expected. Assert will halt the test execution if the condition fails, while verify allows the test to continue running and collects all the failures to report at the end.
How can code coverage and test effectiveness be measured?
Code coverage measures the percentage of code executed by tests, helping to identify untested parts of the codebase. Test effectiveness can be assessed by the ability of tests to detect defects, which can be evaluated through techniques like mutation testing and the thoroughness of test cases.
What are some advanced unit testing techniques and tools?
Advanced unit testing techniques include mutation testing, which introduces faults to assess test suite effectiveness, and fuzzing, which tests applications by inputting unexpected or random data. Tools for these techniques range from automated testing frameworks to specialized software designed for rigorous testing scenarios.