Exploring the Different Varieties of Program Testing Techniques
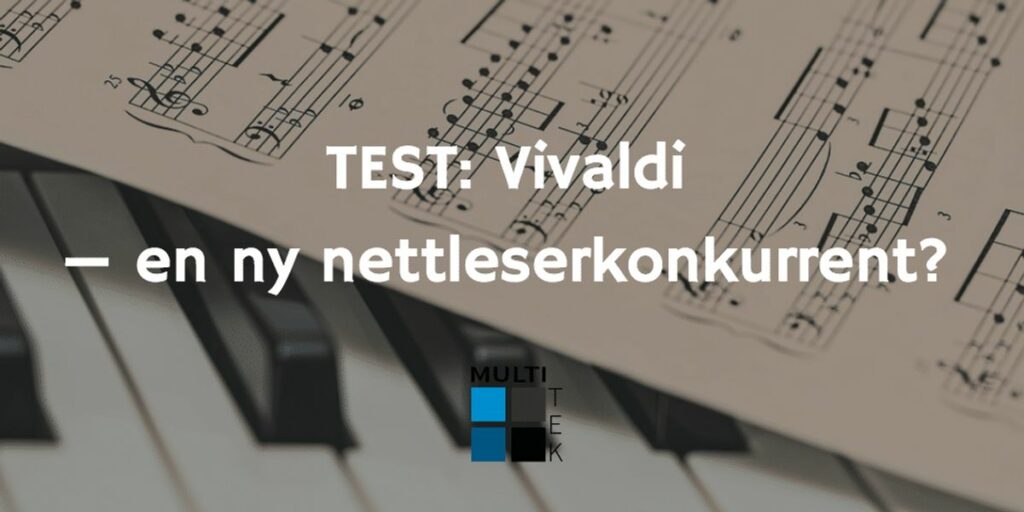
In the dynamic realm of software development, testing stands as a critical phase that ensures quality and reliability. The article ‘Exploring the Different Varieties of Program Testing Techniques’ dives into the multifaceted world of software testing, unraveling the myriad techniques employed to scrutinize software at various levels. From foundational strategies like unit testing to specialized practices like security testing, this article provides a comprehensive overview of the methodologies that fortify software against defects and malfunctions.
Key Takeaways
- Software testing is an essential component of software development, aiming to identify and resolve defects.
- Testing techniques range from basic unit and integration tests to advanced methods like equivalence partitioning and decision table testing.
- Non-functional testing, including performance, usability, and compatibility testing, is crucial for assessing software quality beyond functional correctness.
- The balance between manual and automated testing is necessary for effective quality assurance, leveraging the strengths of both approaches.
- Specialized testing practices such as security and user acceptance testing are indispensable for ensuring software robustness and user satisfaction.
Core Testing Strategies
Unit Testing – The Foundation of Code Stability
Unit testing is a critical practice in software development, aimed at ensuring the stability and reliability of individual code units. By isolating each part of the program and verifying its correctness, developers can catch issues early, minimizing the impact on the overall system.
- Unit tests serve as a form of documentation, clarifying the expected behavior of code segments.
- They provide immediate feedback to developers, allowing for quick and confident issue resolution.
- The practice encourages clean, modular code, as it often highlights design flaws.
Moreover, unit tests are instrumental in preventing regressions, ensuring that previously resolved defects do not reappear after subsequent changes. This continuous testing cycle is fundamental to maintaining code quality throughout the development process.
Integration Testing – Ensuring Module Interoperability
Integration testing is a critical phase in the software development lifecycle, focusing on the interactions between different software modules. It aims to detect interface and communication defects that can occur when individual units are combined to form a larger system. This type of testing is essential for verifying that combined components function together as intended.
The process typically follows unit testing and precedes system testing, acting as a bridge between the two. There are various approaches to integration testing, such as the top-down or bottom-up methods, each with its own merits. For instance:
- Top-down: Starts from the topmost modules and integrates downwards, using stubs for lower-level modules not yet integrated.
- Bottom-up: Begins with the lowest-level modules and integrates upwards, using drivers for higher-level modules not yet integrated.
Integration testing can uncover a range of issues, from data flow problems to failed interactions between modules. The table below summarizes common problems identified during integration testing:
Issue Type | Description |
---|---|
Data flow errors | Incorrect data passed between modules |
Interface mismatches | Incompatibilities in module connections |
Functionality gaps | Missing or incorrect functions in the integration |
By addressing these issues early, integration testing helps to ensure a smoother transition to system testing, where the entire application is evaluated.
System Testing – Validating the Complete System Functionality
System testing stands as a critical phase in the software development lifecycle. It is the process where the software is tested as a whole system to ensure that all components work together harmoniously. This type of testing is typically conducted after integration testing and before acceptance testing, serving as a final verification before the software reaches the end-user.
The primary goal of system testing is to validate that the software meets both functional and non-functional requirements. It encompasses a variety of test types, including but not limited to performance, reliability, security, and usability testing. Each of these areas is crucial for delivering a robust software product that performs well under various conditions and meets user expectations.
Benefits of system testing include:
- A comprehensive assessment of the software’s end-to-end functionality.
- Identification and resolution of usability issues, glitches, and inconsistencies.
- Assurance that the software behaves correctly in the user’s working environment and fulfills user requirements.
Advanced Testing Techniques
Equivalence Partitioning – Dividing Input Data
Equivalence Partitioning is a method used in Black Box Testing to reduce the number of test cases by dividing input data into classes that are expected to exhibit the same behavior. This technique assumes that all values from a particular class will yield identical results, thus identifying redundant test cases that do not contribute to the discovery of new defects.
The process involves creating valid and invalid equivalence classes for input data. For example, if an application accepts whole numbers between 10 and 50 that are multiples of 10, the equivalence classes could be defined as follows:
- Valid Equivalence Class: {10, 20, 30, 40, 50}
- Invalid Equivalence Class (not a multiple of 10): {11, 12, …, 49}
- Invalid Equivalence Class (outside range): {1, 2, …, 9, 51, 52, …, 100}
By selecting just one representative from each class, testers can efficiently cover all scenarios without executing excessive and repetitive test cases. This strategic approach not only streamlines the testing process but also ensures comprehensive coverage of the input domain.
Boundary Value Analysis – Testing at the Edge
Boundary Value Analysis (BVA) is a testing technique that targets the edges of input ranges where defects are more likely to occur. It is a type of Black Box testing, which means it is based on the requirements and functionality without considering the internal system design. BVA is particularly effective because programmers often make off-by-one errors, such as using ‘<‘ instead of ‘<=’ in conditions.
When applying BVA, testers focus on the values at the extreme ends of input ranges. For example, if a function is expected to handle numbers from 1 to 500, BVA would suggest testing at least the values 0, 1, 2, 499, 500, and 501. This approach helps to uncover defects that occur at the boundary values which might not be detected by other testing methods.
Here is a simplified example of how BVA can be structured:
S.no | Test Data | Expected Result | Classes Covered |
---|---|---|---|
1 | 30 | True | 1, 3, 6 |
2 | 5 | False | 4, 7 |
3 | 15.5 | False | 2 |
4 | 56 | False | 5 |
The guidelines for BVA suggest testing at the minimum and maximum values, as well as just above and below these thresholds. This methodical approach ensures a thorough examination of potential weak points in the software’s input handling.
Decision Table Testing – Evaluating Complex Business Rules
Decision Table Testing, also known as Cause-effect graphing, is a structured approach to identify and evaluate the outcomes of different combinations of input conditions. This technique is particularly useful when dealing with complex business rules that have multiple permutations and combinations of inputs and outputs. It provides a clear and comprehensive representation of system behavior under various scenarios, making it easier to understand and test the logic of the system.
The process of creating decision tables involves several steps. Initially, the causes (input conditions) and effects (actions) are identified for a given module. A cause-effect graph is then developed, which is subsequently converted into a decision table. This table serves as the basis for generating test cases that cover all possible scenarios. For example, consider a rule where an employee’s salary must be within a certain range. The decision table would outline the boundaries and expected outcomes for test cases around these salary limits.
Here’s a simplified example of a decision table used to test the salary validation logic:
S.No | Test Data | Expected Result |
---|---|---|
1 | 9999 | False |
2 | 10000 | True |
3 | 20000 | True |
4 | 20001 | False |
By systematically testing each condition outlined in the decision table, testers can ensure that the system behaves as expected across all defined rules, thereby validating the complex business logic embedded within the software.
Non-Functional Testing Types
Performance Testing – Assessing Speed and Efficiency
Performance testing is a critical component of software quality assurance, focusing on evaluating how well an application performs under stress. This type of testing is essential for determining the speed, scalability, stability, and responsiveness of software when subjected to various load and stress conditions. It is typically conducted during or after system testing to ensure that the application can handle expected and peak user traffic without degradation or crashes.
Key aspects of performance testing include assessing load time, throughput, latency, and resource utilization. Tools such as Loader.IO, JMeter, and LoadRunner are commonly used to facilitate this process. The benefits of performance testing are numerous:
- Identification of performance bottlenecks and issues
- Assessment of application’s workload handling capabilities
- Optimization of hardware and software resource utilization
- Measurement of response times for user interactions
By addressing performance bottlenecks before deployment, organizations can avoid potential system failures and ensure a smooth user experience.
Usability Testing – Focusing on User Experience
Usability testing is essential in assessing how intuitive and user-friendly a software application is. It involves evaluating the software from the perspective of the end user, focusing on aspects such as ease of use, learnability, and overall satisfaction. This type of testing is crucial for ensuring that the system is not only functional but also accessible and efficient for its intended audience.
Key elements of usability testing include the examination of the software’s navigation, layout, and design, as well as the effectiveness of any feedback mechanisms. It is typically conducted during or after system testing to identify any areas that may hinder the user experience. For instance, in a stock trading mobile app, testers would assess whether the app provides a clear overview of the market upon launch and if it can be easily operated with one hand.
The goal of usability testing is to create a seamless and engaging user experience, which is vital for the success of any software application. Testers often rely on their domain knowledge and previous experience to uncover defects and suggest improvements that can make the software more user-centric.
Compatibility Testing – Ensuring Cross-Platform Functionality
In the realm of software development, compatibility testing is a critical step to verify that applications perform consistently across various environments. This type of testing assesses the software’s interoperability with different hardware, software, operating systems, browsers, and devices. It is a task typically undertaken during or after system testing to ensure a seamless user experience regardless of the platform.
One specific area within compatibility testing is browser compatibility testing. This ensures that web applications operate effectively across a combination of different browsers and operating systems. It’s not just about functionality; it’s also about maintaining the look and feel of the application. The goal is to provide a positive user experience, whether the user accesses the software on the latest version of Chrome, an older edition of Internet Explorer, or any other browser.
Cross-browser testing is essential because users have diverse preferences for operating systems, browsers, and devices. Companies aim to deliver a good user experience across all these variables. Here’s a brief overview of why cross-browser testing is indispensable:
- Diverse User Base: Different users have different system configurations.
- Consistent Performance: Ensuring the application works well on all platforms.
- Uniform Look and Feel: The application should look and function the same across browsers.
By mastering cross-platform compatibility testing, developers can ensure a consistent gaming experience or any other software interaction across all platforms, as highlighted in iXie’s Guide.
Manual vs Automated Testing
Manual Testing – The Human Touch in Quality Assurance
Manual testing stands as a critical component in the software development lifecycle, where human expertise and intuition play a pivotal role. It involves testers acting as end-users to identify bugs and unexpected behaviors, ensuring that the software functions correctly across various scenarios. This type of testing is not only about following scripted test cases but also about exploratory sessions where testers delve into the software without predefined steps, uncovering issues from a user’s perspective.
The value of manual testing is evident in its flexibility and adaptability. Testers can swiftly respond to new insights and evolving requirements, which is particularly beneficial during the early stages of development. While automated testing can execute predefined scenarios rapidly, it lacks the ability to interpret and react to unscripted events. Manual testing fills this gap, providing a safety net that captures the nuances automation may miss.
Here are some key aspects of manual testing:
- Human skills and domain knowledge are essential.
- Suitable for exploratory testing and early development phases.
- Complements automated testing by exploring edge cases.
- Involves various stages such as unit, system, user acceptance, and integration testing.
Automation Testing – Speed and Repeatability
Automation testing is a powerful tool in the QA arsenal, offering significant advantages in terms of speed and efficiency. By automating repetitive and regression testing tasks, teams can execute test scenarios quickly and repeatedly, which is especially beneficial for large-scale projects with numerous test cases. This approach not only saves time but also enhances the precision of the testing process.
The repeatability and consistency of automated tests are unmatched. Once written, these tests can be run any number of times, ensuring that the same test cases are executed in the same manner, thereby minimizing the risk of human error. This is particularly useful for load testing and stress testing, where consistent execution is crucial.
Here are some key benefits of automation testing:
- Enhanced testing efficiency
- Improved testing accuracy
- Increased overall test coverage
- Time and cost savings
While automation excels in handling repetitive tasks and ensuring test consistency, it is important to remember that it complements rather than replaces manual testing. The latter continues to play a vital role, particularly when a focus on usability and exploratory testing is required.
Comparing Manual and Automated Approaches
The debate between manual and automated testing is a pivotal one in the realm of software quality assurance. Manual testing is characterized by its flexibility and the unique human insight it provides, allowing testers to perform checks on the fly without the need for advance planning. On the other hand, automated testing utilizes scripts, code, and tools to execute tests, offering speed and repeatability that manual methods cannot match.
Choosing between manual and automated testing often depends on the specific requirements and context of the project. Manual testing is indispensable when tests require human observation for nuances that a script cannot detect. Conversely, automated testing excels in repetitive, data-intensive scenarios where consistency and efficiency are paramount. The best outcomes are usually achieved through a combination of both, leveraging the strengths of each to cover the software’s functionality comprehensively.
Here are some considerations when deciding between manual and automated testing:
- Manual testing is ideal for exploratory, ad-hoc, and usability testing.
- Automated testing is preferred for regression, load, and performance testing.
- Manual testing allows for more spontaneous and flexible test execution.
- Automation ensures tests are performed identically every time, reducing the risk of human error.
Specialized Testing Practices
Security Testing – Safeguarding Against Threats
Security testing is a critical phase in the software development life cycle, aimed at uncovering vulnerabilities, weaknesses, and potential threats. It ensures that the software can withstand security breaches and protects sensitive data from unauthorized access. This type of testing is not only about fending off malicious programs and viruses but also about verifying the robustness of authentication and authorization mechanisms.
The benefits of conducting thorough security testing are manifold. It enhances the software’s resilience against cyber threats and maintains the organization’s reputation by preventing data breaches. Moreover, it ensures compliance with industry-specific regulations and standards, which is crucial in today’s regulatory environment.
Here are some key benefits of security testing:
- Enhances the software’s resilience against cyber threats.
- Protects sensitive data, customer information, and intellectual property.
- Maintains the organization’s reputation by preventing data breaches.
- Ensures compliance with industry-specific regulations and standards.
Vulnerability testing, a subset of security testing, focuses on identifying weaknesses in software, hardware, and networks. It is essential to conduct vulnerability testing before production to identify critical defects and flaws in security that could be exploited by hackers.
User Acceptance Testing – Meeting End-User Expectations
User Acceptance Testing (UAT) is the final verification phase before a software product is released into production. It involves real-world end-users or stakeholders who assess the software to ensure it aligns with their needs and expectations. UAT is crucial for confirming that the software delivers the expected business value and supports organizational objectives.
The benefits of UAT are manifold:
- It provides a safety net by uncovering defects or deviations from the specified requirements that might have been missed during previous testing stages.
- Engaging end-users in the testing process enhances user satisfaction as it ensures the software is tailored to meet their expectations.
- Early detection and resolution of issues during UAT can lead to significant cost savings, as fixing problems post-release is often more expensive and challenging.
In summary, UAT serves as a critical checkpoint for quality assurance, providing a platform for feedback and ensuring that the software is ready for successful deployment.
Exploratory Testing – Unscripted and Insightful
Exploratory testing stands out as a dynamic and intuitive approach to quality assurance. Testers act as detectives, using their creativity and experience to navigate through the application without the constraints of predefined test cases. This method is particularly effective when documentation is scarce or when an additional layer of testing is desired after traditional scripted methods have been applied.
The essence of exploratory testing lies in its freedom and adaptability. Testers are encouraged to follow their instincts, which often leads to the discovery of defects that structured testing might miss. It’s a thinking process that requires a tester’s intellect and smart work, making it a valuable tool for uncovering critical issues early in the development cycle.
Exploratory testing is not just about random actions; it’s a sophisticated technique that includes various agile methodologies such as User Journey tests and User Story testing. These practices are increasingly sought after for their ability to provide rapid and insightful feedback during the agile development process.
Conclusion
Throughout this article, we have explored an array of software testing techniques, each with its unique approach to ensuring the quality and reliability of software products. From the precision of Equivalence Partitioning to the comprehensive coverage of Path Testing, and the practical insights of User Story Testing, we’ve seen how diverse methods cater to different testing needs. It’s clear that the selection of appropriate testing techniques is crucial for identifying defects, validating functionality, and verifying the performance of software systems. As the software development landscape continues to evolve, so too will the techniques for testing, underscoring the importance of staying informed and adaptable in our testing strategies. Whether you’re a seasoned tester or new to the field, the knowledge of these techniques will be instrumental in your quest to deliver robust and user-friendly software.
Frequently Asked Questions
What is the primary goal of Unit Testing?
The primary goal of Unit Testing is to validate that each individual unit of the software performs as designed, ensuring code stability and helping to catch errors early in the development process.
How does Integration Testing differ from System Testing?
Integration Testing focuses on ensuring that different modules or components of a software system work together properly, while System Testing validates the complete and fully integrated software product to ensure it meets all specified requirements.
What is Equivalence Partitioning in software testing?
Equivalence Partitioning is a testing technique that divides input data into equivalent partitions where test cases can be designed to cover each partition, reducing the number of test cases required while maintaining coverage.
Why is Performance Testing important in software development?
Performance Testing is crucial for assessing the speed, scalability, and efficiency of a software application, ensuring that it can handle expected loads and providing a good user experience under various conditions.
What are the benefits of Automated Testing over Manual Testing?
Automated Testing offers benefits such as faster execution of tests, repeatability, reliability, and the ability to run tests frequently without additional cost, which can be especially useful for regression testing and continuous integration.
Can you explain what Security Testing involves?
Security Testing involves evaluating a software system to discover vulnerabilities, threats, and risks that could lead to a security breach, with the aim of safeguarding data and maintaining integrity, confidentiality, and availability.