Exploring Unit Test Examples: A Step-by-Step Guide
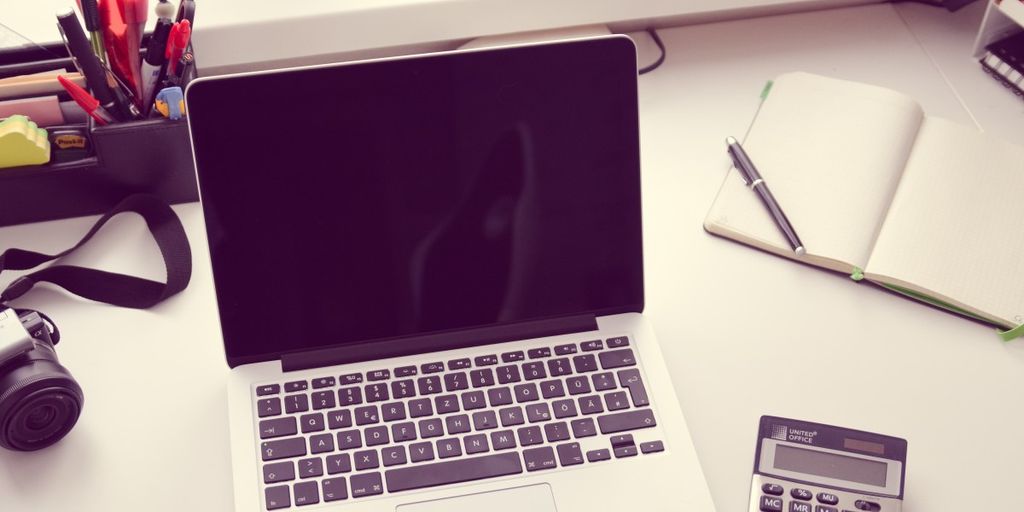
Unit testing is a crucial aspect of software development that ensures individual components of an application work as expected. This article delves into unit test examples, providing a comprehensive step-by-step guide to help developers understand, implement, and maintain effective unit tests across various programming environments.
Key Takeaways
- Understanding the fundamental concepts and components of unit testing is essential for effective implementation.
- Selecting appropriate tools and setting up a conducive development environment are critical steps in unit testing.
- Writing and running your first unit test can be simplified with a systematic approach to creating test cases and analyzing results.
- Advanced techniques such as mocking, stubbing, and dependency injection enhance the flexibility and robustness of unit tests.
- Maintaining unit tests involves regular updates and documentation to ensure they remain effective and relevant over time.
Understanding the Basics of Unit Testing
Definition and Importance
Unit testing is a software testing method where individual units or components of a software are tested. The purpose is to validate that each unit of the software performs as designed. Unit testing is crucial because it ensures early detection of errors, simplifies integration, and facilitates changes.
Key Components of a Unit Test
A unit test typically consists of three main components:
- Input: Data provided to the test.
- Execution: The process where the unit is invoked.
- Output: The result of the execution.
The Role of Assertions
Assertions are statements that check if a particular condition is true. They are essential in unit testing as they determine whether a test passes or fails. Effective use of assertions can significantly improve the quality of tests by ensuring that the software behaves as expected under various conditions.
Setting Up Your Environment for Unit Testing
Choosing the Right Tools and Frameworks
Selecting the appropriate tools and frameworks is crucial for effective unit testing. Research and compare different options based on the programming language and project requirements. Popular frameworks include JUnit for Java, PyTest for Python, and Jest for JavaScript.
Configuring Your Development Environment
Ensure your development environment is optimized for testing by integrating the chosen unit testing frameworks. Set up necessary plugins and ensure that your IDE supports easy test execution and debugging.
Best Practices for a Testable Codebase
To maintain a testable codebase, adhere to principles like SOLID and DRY. Structure your code to make it easy to isolate components for testing, and avoid tight coupling. Regular code reviews and refactoring play a significant role in keeping the codebase clean and testable.
Writing Your First Unit Test
Creating a Simple Test Case
Start by identifying a small, isolated functionality of your application to test. Write a clear, concise test that verifies this functionality behaves as expected under various conditions. Use assertions to validate the outcomes.
Running and Interpreting Results
Execute your test using your chosen framework’s tools. Review the test results carefully; successful tests confirm your code’s reliability, while failures highlight areas needing improvement.
Troubleshooting Common Issues
When tests fail, first check for common errors like typos or incorrect setup. If the issue persists, consider whether your test is designed correctly or if there’s an underlying bug in the code. Debug systematically to isolate and resolve the problem.
Advanced Unit Testing Techniques
Mocking and Stubbing
Mocking and stubbing are essential techniques in unit testing that allow you to simulate the behavior of complex dependencies. By using mocks and stubs, you can isolate the unit of code under test, ensuring that tests run quickly and reliably. These techniques are particularly useful when dealing with external services or databases.
Parameterized Testing
Parameterized testing helps to improve test coverage by running the same test logic with different input values. This approach reduces redundancy in test code and makes it easier to identify edge cases. Parameterized tests are designed to validate the smallest possible unit of code in various scenarios, enhancing the robustness of your software.
Using Dependency Injection
Dependency injection is a technique used to manage code dependencies, making unit tests more maintainable and flexible. By injecting dependencies, you can easily swap out real implementations with mock objects during testing. This method supports a clean separation of concerns and simplifies the testing process.
Unit Testing in Different Programming Languages
Java
Java, known for its robustness and object-oriented capabilities, offers several frameworks for unit testing. JUnit is the most popular, providing a simple yet powerful testing platform. Developers can leverage annotations like @Test
to denote test methods, ensuring a structured approach to testing.
Python
Python’s dynamic nature and readability make it an excellent choice for unit testing. pytest and unittest
are widely used frameworks that support various testing styles. Python’s simplicity allows for writing concise and readable tests, enhancing maintainability.
JavaScript
JavaScript’s asynchronous behavior and event-driven programming require a unique approach to unit testing. Frameworks like Mocha and Jest provide comprehensive testing solutions that cater to JavaScript’s specific needs. These tools help manage asynchronous code and offer features like real-time feedback and automated browser testing.
Integrating Unit Tests with Build Tools
Integrating unit tests into build tools is a crucial step for automating and streamlining the testing process. This integration helps in ensuring that tests are not just a checkbox in the development process but a core part of the software development lifecycle.
Automating Tests with CI/CD Pipelines
Automating unit tests within CI/CD pipelines ensures that every code commit is verified, leading to higher code quality and early detection of potential issues. This automation can be configured to run on various triggers such as push, pull requests, or scheduled events.
Configuring Build Tools for Test Automation
Proper configuration of build tools is essential for effective test automation. This involves setting up the environment, selecting the right plugins, and configuring the build scripts to execute unit tests automatically.
Handling Test Failures and Errors
Effective handling of test failures and errors is critical for maintaining the integrity of the build process. Strategies include setting up notifications for failed tests, using retry mechanisms, and isolating flaky tests to prevent them from blocking the build pipeline.
Best Practices for Maintaining Unit Tests
Maintaining unit tests is crucial for ensuring the long-term effectiveness of testing strategies. Proper maintenance helps in adapting to new requirements and avoiding obsolete or redundant tests.
Keeping Tests Up-to-Date
Regular updates to unit tests are essential as the codebase evolves. This involves revising tests when new features are added or existing features are modified. It’s important to remove or refactor outdated tests to reflect the current state of the application.
Balancing Unit and Integration Tests
Achieving a balance between unit tests and integration tests ensures comprehensive coverage and efficient testing. Unit tests should focus on individual components, while integration tests should verify how these components interact.
Documenting Test Cases
Well-documented test cases improve understandability and maintainability. Documentation should include the purpose of the test, the methodology used, and any special considerations. This makes it easier for new developers to understand the testing framework and for existing developers to modify tests as needed.
Conclusion
In this guide, we’ve explored various unit test examples, providing a comprehensive step-by-step approach to understanding and implementing effective testing strategies. By dissecting different scenarios and techniques, we aim to enhance your ability to write robust unit tests that ensure your code functions as intended. Remember, consistent practice and learning from each test case will significantly improve your testing skills and software quality. We encourage you to apply these insights and continue exploring more complex testing environments to become proficient in unit testing.
Frequently Asked Questions
What is unit testing and why is it important?
Unit testing involves testing individual components of software to ensure they work as expected. It’s important because it helps catch bugs early, simplifies debugging, and improves code quality.
How do I choose the right tools for unit testing?
Select tools that integrate well with your development environment and support the programming languages you use. Popular choices include JUnit for Java, pytest for Python, and Jest for JavaScript.
What are the key components of a unit test?
A unit test typically includes a setup phase to prepare the test environment, the invocation of the method to be tested, and assertions to verify the output against expected results.
How can I ensure my codebase is testable?
Design your code with testability in mind by using principles like modularity, loose coupling, and high cohesion. Also, make use of dependency injection to make components easier to test.
What are some advanced unit testing techniques?
Advanced techniques include mocking and stubbing to simulate complex dependencies, parameterized testing to cover a range of input scenarios, and using dependency injection to manage external dependencies.
How do I handle test failures and errors in automated test environments?
Investigate and fix failing tests promptly to maintain the integrity of the codebase. Use detailed logs to aid troubleshooting, and configure your CI/CD pipeline to alert you to failures immediately.