How to Effectively Test a Unit: Best Practices
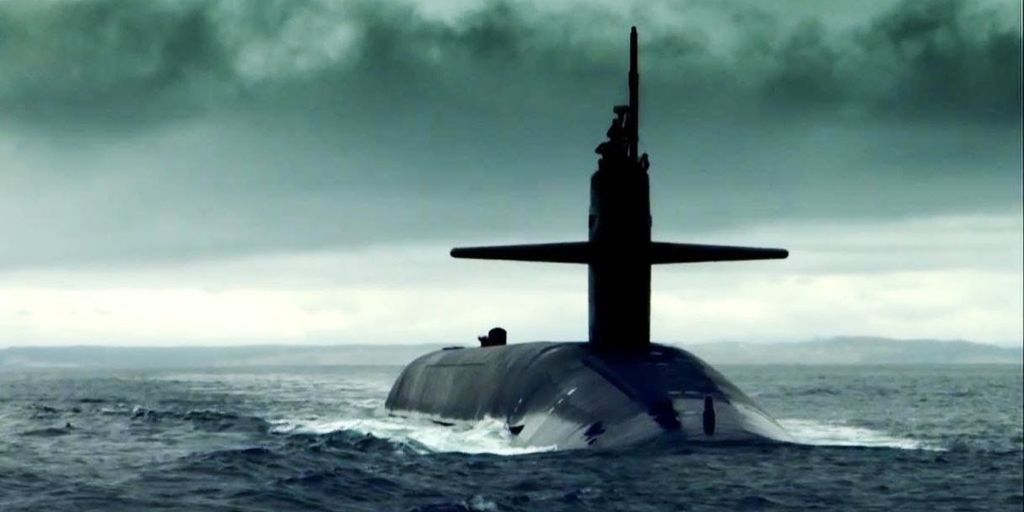
Unit testing is a fundamental aspect of software development, ensuring that individual units of source code function correctly. This article explores effective strategies for designing, implementing, and maintaining unit tests, providing insights into best practices that can enhance the quality and reliability of software projects.
Key Takeaways
- Understanding unit testing fundamentals is crucial before diving into best practices.
- Effective unit tests are characterized by clarity in naming, isolation, and specific assertions.
- Testing should cover various scenarios, including both expected and edge cases, to ensure robustness.
- Performance optimization and maintaining test isolation are essential for accurate and efficient testing outcomes.
- Regular maintenance and thoughtful refactoring of unit tests are necessary to keep them useful and relevant over time.
Understanding the Basics of Unit Testing
What is a Unit Test?
Unit testing ensures that the units within your program are working as expected. It is usually the developer’s responsibility to unit test their own code, as they have the greatest insight into its expected behavior. This form of testing is crucial for maintaining code quality from the early stages of development.
Key Components of Unit Testing
Unit testing involves several key components that ensure each unit operates correctly:
- Functionality: Testing if the unit performs as intended.
- Accuracy: Ensuring the unit produces the correct outputs.
- Efficiency: Assessing if the unit operates within acceptable resource limits.
Why Unit Testing is Essential
Unit testing is essential because it helps identify problems early in the development process, reducing the cost and time required for later corrections. It also supports code quality and reliability, making the overall software more robust against future changes or integrations.
Designing Effective Unit Tests
Arranging Test Conditions
To design effective unit tests, start by arranging test conditions meticulously. This involves setting up the necessary state of the object or method before invoking it. A well-arranged test ensures that the unit operates under controlled and predictable conditions, which is crucial for accurate testing.
Acting on the Test Unit
The next step is to act on the test unit by calling the method or triggering the event you want to test. This action should mimic the real-world usage as closely as possible to ensure the test is relevant and covers the expected functionality.
Asserting Test Outcomes
Finally, asserting test outcomes is essential. This step verifies that the output or behavior of the unit matches the expected results. Effective assertions are clear and directly related to the test conditions and actions, providing a straightforward way to check if the unit behaves as intended under various conditions.
Writing Clear and Descriptive Test Names
Importance of Descriptive Names
Descriptive test names are crucial for maintaining readable and maintainable code. They act as documentation, helping both current and future developers understand what each test covers without diving into the details of the test implementation.
Examples of Good Test Names
Good test names should clearly reflect the intent of the test and the specific scenario it addresses. Here’s a simple structure to follow:
- Method being tested
- Scenario under which it is tested
- Expected behavior when the scenario is invoked
This structure ensures that the test name communicates sufficient details about what is being tested and the conditions under which it is tested.
Common Mistakes in Naming Tests
One common mistake in naming tests is using vague or non-descriptive terms that do not convey the specific purpose of the test. Avoid generic names like test1
or test2
, and instead, opt for names that provide clear and actionable insights into the test’s intent and scope.
Ensuring Test Isolation for Accurate Results
What is Test Isolation?
Test isolation refers to the practice of running each unit test in a controlled environment where it does not share any state with other tests. This approach ensures that the outcome of a test is solely dependent on the unit under test and not on external factors or prior test results.
Benefits of Isolated Tests
Isolated tests are crucial because they:
- Ensure reproducibility of results
- Minimize flakiness
- Enhance test reliability
- Speed up the testing process by eliminating interdependencies
Strategies for Isolating Tests
To achieve test isolation, consider the following strategies:
- Use mock objects to simulate external dependencies.
- Ensure each test cleans up its environment during teardown.
- Run tests in isolation from one another to prevent cross-contamination.
- Utilize containers or virtual environments to mimic production environments closely.
Optimizing Test Performance
Making Tests Run Faster
To ensure that unit tests are efficient and do not hinder the development process, it is crucial to make your tests run as fast as possible. Slow tests discourage frequent execution, which is essential for maintaining code quality. Consider strategies such as minimizing test dependencies and avoiding complex logic in tests to speed up execution times.
Balancing Test Coverage and Performance
Achieving a balance between thorough test coverage and optimal performance is key. While it’s important to cover a wide range of scenarios, it’s equally important to ensure that tests remain fast and maintainable. Use techniques like prioritizing critical paths and employing test automation to achieve this balance.
Tools for Measuring Test Performance
To effectively optimize test performance, utilizing tools to measure and monitor test speeds is essential. Tools like benchmarking suites and performance profiling can provide valuable insights into how tests perform under different conditions, allowing for targeted improvements.
Testing Across Multiple Scenarios
Covering Happy and Edge Cases
Testing both expected and unexpected outcomes is crucial for robust software. Test positive and negative scenarios to ensure that your application can handle both success and failure gracefully. Consider testing boundary conditions, such as the minimum and maximum values of input variables, to cover a comprehensive range of scenarios.
Error Handling in Tests
Effective error handling in tests is essential for identifying and mitigating potential issues before they reach production. Ensure that your tests are designed to catch and properly handle errors, making your code more reliable and easier to maintain.
Using Mocks and Stubs
Mocks and stubs are powerful tools for testing across multiple scenarios without relying on external systems. They allow you to simulate various inputs and behaviors, making your tests both faster and more isolated. Use mocks and stubs to test how your application behaves under different conditions, ensuring that all functionalities are tested thoroughly.
Maintaining and Refactoring Unit Tests
When to Refactor Tests
Refactoring tests is crucial when you notice that they are becoming too coupled to the internals of the code they’re testing. This ensures they remain resilient and provide a safety net during code changes. Refactor tests to improve clarity, reduce redundancy, and enhance test performance.
Keeping Tests Up-to-Date
It’s essential to regularly update your unit tests to reflect changes in the application’s codebase. This practice prevents outdated tests from misleading developers by failing incorrectly or passing despite bugs.
Best Practices for Test Maintenance
To maintain the integrity and effectiveness of your unit tests, follow these best practices:
- Adopt Test-Driven Development (TDD) to ensure every piece of code is tested before it is written.
- Use a suitable testing framework that fits the project’s needs.
- Write clear and isolated tests to minimize the risk of interference from other tests.
- Regularly review and update tests to cover new code paths and edge cases.
Conclusion
Throughout this article, we’ve explored various best practices for unit testing that can significantly enhance the quality and reliability of your software development process. From understanding the importance of naming conventions and test isolation to recognizing the need for tests to be fast, simple, and timely, these guidelines serve as a cornerstone for effective unit testing. As you implement these practices, remember that the ultimate goal is to create a robust testing environment that supports continuous improvement and helps catch issues early, ultimately leading to a more stable and maintainable codebase.
Frequently Asked Questions
What is a Unit Test?
A unit test is an automated piece of code that invokes a unit of work in the system and then checks a single assumption about the behavior of that unit of work.
Why is Unit Testing essential?
Unit testing ensures that all code meets quality standards before it’s deployed. This helps to catch and fix bugs early in the development cycle, saving time and money.
What does a good unit test name entail?
A good unit test name should clearly describe what the test intends to achieve, allowing for better understandability and easier maintenance.
How can tests be made to run faster?
Optimizing test data, reducing external dependencies, and running tests in parallel are some strategies to make tests run faster.
What is test isolation?
Test isolation means that no test case should depend on the state of another. Each test should setup its own data and not rely on external data.
Why should tests cover multiple scenarios?
Covering multiple scenarios, including edge cases and error conditions, ensures that the application behaves correctly under various conditions, thereby increasing the robustness of the software.