How to Write Effective Unit Test Cases
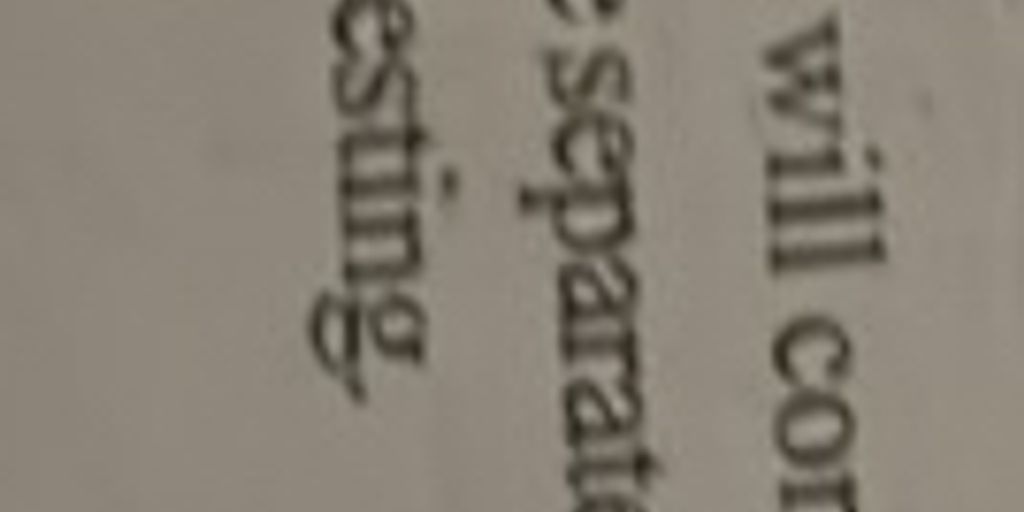
Unit testing is a key part of making sure your code works well. It helps catch bugs early, making your code more reliable and easier to maintain. This article will guide you on how to write effective unit test cases, covering characteristics, best practices, common pitfalls, tools, advanced techniques, maintenance, and the role of unit testing in agile development.
Key Takeaways
- Keep your tests simple and readable to make them easier to understand and maintain.
- Use descriptive names for your tests so you can easily tell what each test is checking.
- Follow the Arrange, Act, Assert pattern to structure your tests clearly.
- Avoid making your tests too complex or dependent on each other.
- Regularly review and update your tests to keep them in sync with your code changes.
Characteristics of Effective Unit Test Cases
Readability and Simplicity
A good unit test should be easy to read and understand. Simple tests are easier to write, maintain, and refactor. When a test is straightforward, it helps developers quickly grasp its purpose and logic. This clarity is crucial for identifying why a test might fail and for ensuring that anyone on the team can work with the test cases.
Isolation and Independence
Effective unit tests must be isolated from each other. This means that each test should run independently, without relying on the outcome of other tests. Isolation ensures that tests do not interfere with one another, making it easier to pinpoint issues when they arise. This characteristic is vital for maintaining the reliability of your test suite.
Speed and Performance
Unit tests should execute quickly. Fast tests provide immediate feedback, which is essential for a smooth development workflow. Quick execution allows developers to run tests frequently, catching issues early in the development process. This speed is a key factor in making unit testing a practical and efficient practice.
Best Practices for Writing Unit Test Cases
Use Descriptive Names
When naming your unit tests, make sure the names clearly describe what the test is checking. A good name helps you understand the test’s purpose without looking at the code. This makes it easier to identify which scenarios are failing when a test doesn’t pass.
Follow Arrange, Act, Assert
A common structure for writing unit tests is the Arrange, Act, Assert pattern. This means you first set up your test data and environment (Arrange), then execute the code being tested (Act), and finally check the results (Assert). This pattern helps keep your tests organized and easy to read.
Keep Tests Short and Focused
Each unit test should focus on a single behavior or scenario. Keeping tests short and focused makes them easier to understand and maintain. It also helps ensure that when a test fails, you can quickly pinpoint the issue.
By following these best practices, you can write unit tests that are more effective and easier to manage.
Common Pitfalls to Avoid in Unit Testing
Overly Complex Tests
Tests can sometimes become too complicated. This can make them hard to understand and maintain. Keep your tests simple. If you need custom functions to check results, make sure they are also tested.
Ignoring Edge Cases
It’s easy to test the usual scenarios, but don’t forget the unusual ones. Edge cases can reveal hidden bugs. Always include tests for these special situations.
Dependent Tests
Each test should stand alone. If one test depends on another, it can cause problems. Make sure your tests are independent so they can run in any order.
Tools and Frameworks for Unit Testing
When it comes to unit testing, having the right tools can make a world of difference. Fortunately, you can do better by using frameworks designed to simplify the process. Let’s explore some of the most popular options available.
Popular Unit Testing Frameworks
There are several well-known frameworks that developers rely on for unit testing. Here are a few:
- JUnit
- NUnit
- TestNG
- PHPUnit
- Mockito
- Cantata
- Karma
These tools help streamline the testing process, making it easier to write, run, and manage tests.
Choosing the Right Tool
Selecting the right tool depends on your specific needs and the programming language you’re using. For instance, JUnit is widely used for Java applications, while NUnit is popular for .NET projects. Consider the following factors when choosing a tool:
- Compatibility with your programming language
- Ease of integration with your development environment
- Community support and documentation
Integrating with CI/CD Pipelines
Integrating unit tests with Continuous Integration and Continuous Deployment (CI/CD) pipelines ensures that your code is tested automatically with every change. This helps catch issues early and maintain code quality. Most modern CI/CD tools support integration with popular unit testing frameworks, making it easier to automate the testing process.
Advanced Techniques for Unit Testing
Mocking and Stubbing
Mocking and stubbing are essential for isolating the code under test. By mastering these advanced techniques, developers can create more robust and reliable tests. Mocks simulate the behavior of real objects, while stubs provide predefined responses. This helps in testing components independently without relying on external systems.
Testing Asynchronous Code
Testing asynchronous code can be tricky, but it’s crucial for modern applications. Using proper tools and frameworks can simplify this process. Ensure your tests wait for promises to resolve or use async/await syntax to handle asynchronous operations effectively. This ensures that your tests are accurate and reflect real-world scenarios.
Parameterization in Tests
Parameterization allows you to run the same test with different inputs, making your tests more comprehensive. This technique helps in covering a wide range of scenarios without writing separate test cases for each input. It not only saves time but also ensures that your code is robust and handles various inputs gracefully.
Maintaining and Refactoring Unit Test Cases
Regularly Review and Update Tests
It’s important to regularly review and update tests to ensure they remain relevant and effective. As your code evolves, your tests should too. This practice helps catch any issues early and keeps your codebase healthy.
Refactor Tests Alongside Code
When you refactor your code, don’t forget to refactor your tests as well. This ensures that your tests are always aligned with your code. Better unit testing practice: each test should test one thing. This makes it easier to identify and fix issues.
Ensure Tests Remain Deterministic
Tests should always produce the same results given the same inputs. Non-deterministic tests can lead to flaky builds and unreliable results. Once both levels are green, we can do any extra refactoring or work on edge cases.
The Role of Unit Testing in Agile Development
Supporting Continuous Integration
Unit testing is a fundamental aspect of software testing where individual components or functions of a software application are tested in isolation. In Agile development, unit tests are crucial for supporting continuous integration (CI). They act as a safety net, ensuring that new changes do not break existing functionality. This allows developers to merge code frequently, reducing integration issues and enabling faster feedback.
Facilitating Test-Driven Development
Unit tests are essential for facilitating Test-Driven Development (TDD). In TDD, tests are written before the code itself. This approach helps developers focus on the requirements and design of the software, leading to cleaner and more reliable code. Unit tests provide immediate feedback, making it easier to identify and fix issues early in the development process.
Enhancing Collaboration Among Teams
Unit testing also enhances collaboration among teams. By having a suite of unit tests, team members can understand the expected behavior of the code, making it easier to work on shared codebases. This transparency fosters better communication and collaboration, as everyone is on the same page regarding the software’s functionality.
Conclusion
Writing effective unit test cases is a crucial skill for any developer. By following best practices such as keeping tests simple, readable, and isolated, you can ensure that your code is reliable and maintainable. Remember, good unit tests not only help catch bugs early but also make your code easier to understand and refactor. As you gain more experience, writing these tests will become second nature, and you’ll find that they are an invaluable part of your development process. Keep practicing and refining your approach, and you’ll see the benefits in the quality of your software.
Frequently Asked Questions
What is a unit test?
A unit test checks a small part of your code to make sure it works right. It’s like testing one piece of a puzzle to see if it fits.
Why are unit tests important?
Unit tests help catch bugs early, make code easier to change, and ensure everything works as expected.
How do I write a simple unit test?
To write a simple unit test, make sure it’s easy to read, focuses on one thing, and runs fast. Use clear names for your tests.
What are common mistakes in unit testing?
Some common mistakes include writing tests that are too complex, ignoring edge cases, and making tests that depend on each other.
Which tools can I use for unit testing?
There are many tools like JUnit, NUnit, and pytest. Choose one that fits your programming language and needs.
How do unit tests fit into Agile development?
In Agile, unit tests support continuous integration, help with test-driven development, and make teamwork easier.