The Importance of Unit Testing in Modern Programming
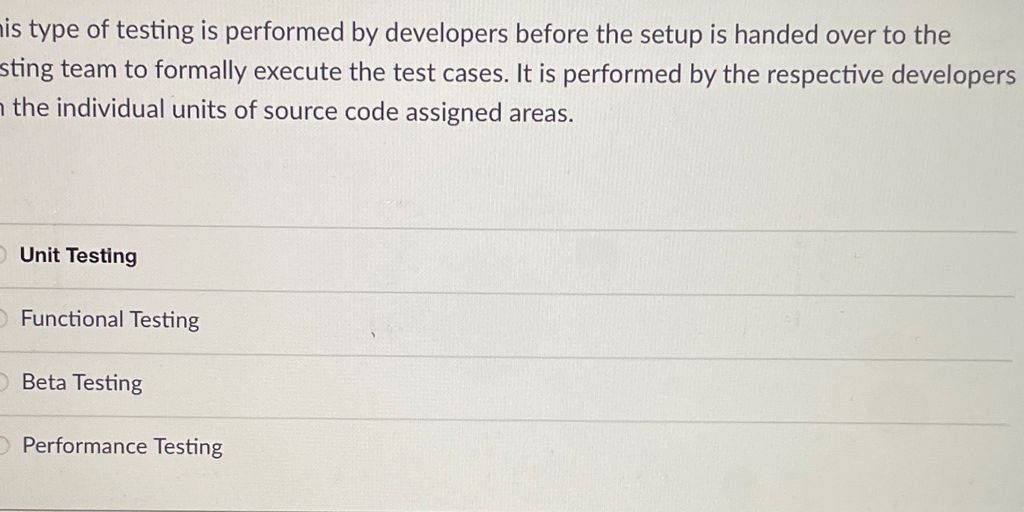
In today’s fast-paced world of software development, ensuring your code works correctly is more important than ever. Unit testing is a method that helps developers make sure each part of their code does what it’s supposed to do. This article will explain what unit testing is, why it’s important, and how you can use it to improve your programming skills.
Key Takeaways
- Unit testing helps catch bugs early, saving time and effort later.
- It makes code easier to change and improve without breaking things.
- Unit tests can make your code more reliable and easier to understand.
- Different programming languages have their own tools for unit testing.
- Good unit tests are simple, independent, and easy to read.
Understanding Unit Testing in Programming
Definition and Purpose
Unit testing is a key part of software development. It involves testing the smallest parts of an application, called units, to make sure they work correctly. These units are often individual functions or sections of code. The main goal is to catch bugs early and ensure each unit meets its design specifications.
Key Components of Unit Tests
A good unit test has several important parts:
- Setup: Prepare the unit for testing.
- Execution: Run the unit with test data.
- Verification: Check if the results are as expected.
- Teardown: Clean up after the test.
Common Misconceptions
There are some common myths about unit testing:
- Unit tests are time-consuming: While they take time to write, they save time by catching bugs early.
- Unit tests are only for large projects: Even small projects benefit from unit tests.
- Unit tests guarantee bug-free code: They help find bugs, but they can’t catch everything.
Benefits of Unit Testing for Developers
Early Bug Detection
Unit testing helps developers catch bugs early in the development process. By identifying issues before they become bigger problems, developers can save time and effort. This early detection leads to more reliable and stable software.
Facilitating Code Refactoring
Unit tests provide a safety net that allows developers to refactor code with confidence. If a change introduces a bug, the tests will fail, alerting the developer to the issue. This makes it easier to improve and optimize the codebase over time.
Improving Code Quality
With unit tests in place, developers can ensure that their code meets certain quality standards. The tests serve as a form of documentation, showing how the code is supposed to behave. This leads to cleaner, more maintainable code.
Best Practices for Writing Unit Tests
Writing Atomic Tests
When writing unit tests, it’s crucial to focus on small, specific pieces of functionality. Each test should be clear and concise, concentrating on a single concept or condition. This makes it easier to understand what is being tested and why a test might fail. Writing atomic tests ensures that each test is isolated and tests only one thing at a time.
Ensuring Test Independence
Tests should be independent of each other. This means that the outcome of one test should not affect the outcome of another. Independent tests are easier to debug and maintain. To achieve this, avoid sharing state between tests and use setup and teardown methods to prepare the test environment.
Maintaining Test Readability
Readable tests are easier to understand and maintain. Use descriptive names for your tests to clearly indicate what they are testing. Additionally, keep your tests simple and avoid complex logic within them. This helps other developers quickly grasp the purpose of the test and the behavior it is verifying.
Unit Testing in Different Programming Languages
Unit Testing in JavaScript
JavaScript is a popular language for web development, and unit testing plays a crucial role in ensuring code quality. Frameworks like Jest and Mocha are commonly used. They help developers test individual functions or methods in isolation, making it easier to catch bugs early.
Unit Testing in Python
Python’s simplicity and readability make it a favorite among developers. The unittest
module, built into Python, provides a solid foundation for writing unit tests. Other popular frameworks include PyTest and Nose, which offer more advanced features and flexibility.
Unit Testing in Java
Java, being a statically-typed language, benefits greatly from unit testing. JUnit is the most widely used framework for Java unit tests. It allows developers to write repeatable tests and is well-integrated with many development tools and continuous integration systems.
Challenges and Solutions in Unit Testing
Dealing with Legacy Code
Working with legacy code can be tough. Often, this code lacks tests, making changes risky. To tackle this, start by writing tests for the most critical parts. Gradually, expand your test coverage as you refactor the code.
Managing Test Flakiness
Flaky tests are tests that sometimes pass and sometimes fail without any changes to the code. They can be frustrating and reduce trust in the test suite. To manage this, identify the root cause, which is often due to timing issues or dependencies on external systems. Use mocks and stubs to isolate the unit under test.
Balancing Test Coverage and Development Time
Achieving high test coverage is important, but it can be time-consuming. Focus on writing tests for the most critical and complex parts of your code first. This way, you ensure that the most important functionalities are well-tested without spending too much time on less critical areas.
Tools and Frameworks for Unit Testing
Several frameworks and tools can assist in the unit testing process. Here are a few commonly used ones:
Popular Unit Testing Frameworks
JUnit is one of the most popular unit testing frameworks for Java. It is easy to use and provides annotations to identify test methods, setup and teardown procedures, and test cases. JUnit also integrates well with popular development environments and build tools.
NUnit is a widely-used unit testing framework for .NET languages. It offers a rich set of assertions and supports data-driven tests, making it a versatile choice for developers.
TestNG is another powerful testing framework for Java. It is designed to cover a wider range of test categories, from unit to integration tests, and provides advanced features like parallel test execution.
Choosing the Right Tool
Selecting the right unit testing tool depends on several factors, including the programming language, development environment, and specific project needs. Here are some steps to help you choose:
- Identify the programming language and platform you are working on.
- Consider the integration capabilities with your development environment and CI/CD pipelines.
- Evaluate the features and flexibility of the tool to ensure it meets your testing requirements.
- Look for community support and documentation to help you get started and troubleshoot issues.
Integrating Unit Tests into CI/CD Pipelines
Integrating unit tests into Continuous Integration/Continuous Deployment (CI/CD) pipelines is crucial for maintaining code quality and ensuring that new changes do not break existing functionality. Here are some benefits:
- Automated testing helps in early bug detection and reduces manual effort.
- Continuous feedback allows developers to address issues promptly.
- Ensures that the codebase remains stable and reliable throughout the development lifecycle.
The Role of Unit Testing in Agile Development
Supporting Continuous Integration
Unit testing is a key part of continuous integration (CI) in Agile development. By running unit tests frequently, developers can catch bugs early and ensure that new code changes do not break existing functionality. This practice helps maintain a stable codebase and speeds up the development process.
Enhancing Collaboration
Unit tests serve as a form of documentation that helps team members understand the code better. When everyone on the team writes and runs unit tests, it fosters a culture of quality and collaboration. This shared responsibility for code quality leads to better communication and more efficient problem-solving.
Adapting to Changing Requirements
Agile development is all about being flexible and responsive to change. Unit tests make it easier to adapt to new requirements because they provide a safety net that ensures changes do not introduce new bugs. This allows teams to iterate quickly and confidently, knowing that their code remains reliable.
Conclusion
In summary, unit testing is a vital part of modern programming. It helps developers catch bugs early, making the code more reliable and easier to maintain. By including unit tests in your workflow, you can create cleaner, more efficient software. Embracing unit testing not only speeds up debugging but also boosts your confidence in the code you write. So, make unit testing a regular practice and enjoy the benefits of better, more dependable applications.
Frequently Asked Questions
What is unit testing in programming?
Unit testing is a way to check small parts of your code to make sure they work correctly. Each small part, or unit, is tested by itself.
Why is unit testing important?
Unit testing helps find bugs early, makes code easier to change, and improves overall quality. It saves time and reduces the risk of larger problems later.
How do unit tests help with code refactoring?
When you change code, unit tests make sure that the changes don’t break anything. This makes it easier to improve and clean up code without worrying about new bugs.
What are some common misconceptions about unit testing?
Some people think unit testing is too time-consuming or only for big projects. In reality, it saves time in the long run and is useful for projects of all sizes.
What are the best practices for writing unit tests?
Good unit tests are small and focused on one thing. They should be independent of each other and easy to read and understand.
Can unit testing be used with any programming language?
Yes, unit testing can be done in almost any programming language. There are special tools and frameworks to help with unit testing in languages like JavaScript, Python, and Java.