The Programmer’s Guide to Effective Unit Testing Techniques
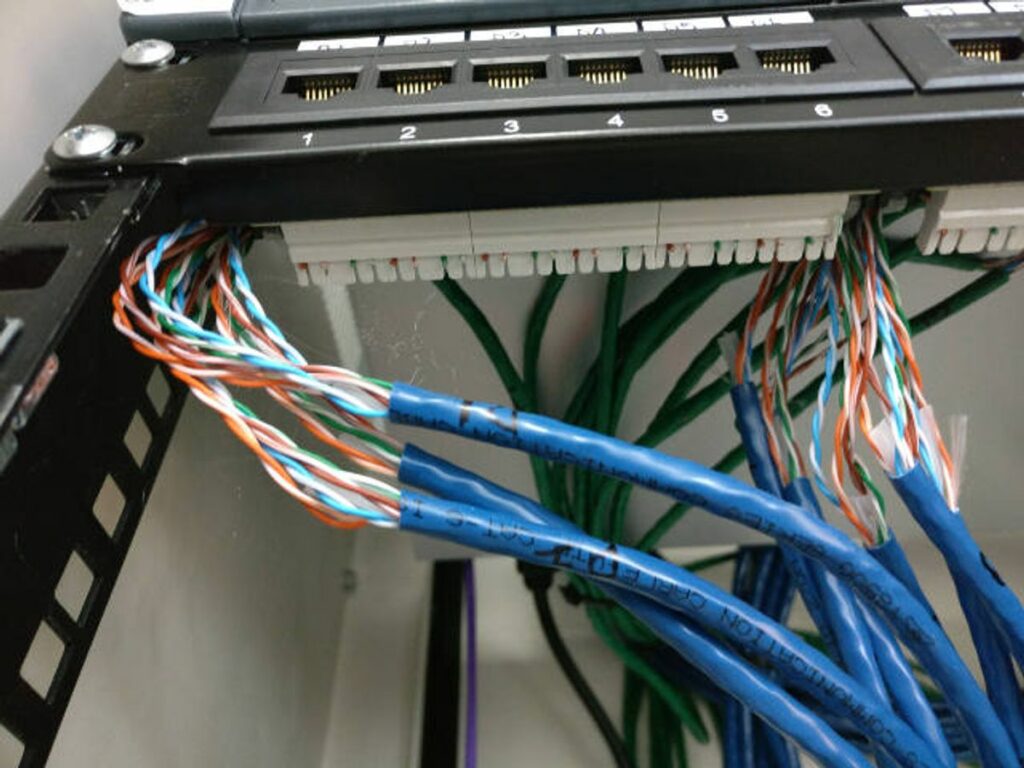
Unit testing is an indispensable software development practice that ensures the quality and reliability of code by testing individual units, such as functions or methods. This guide aims to provide developers with advanced insights and step-by-step techniques to enhance their unit testing practices using tools like Jest in Node.js and TypeScript environments. Whether you’re a beginner or an experienced developer seeking to deepen your knowledge, this guide offers practical advice and a structured approach to improving your testing practices.
Key Takeaways
- Understanding unit testing fundamentals is the cornerstone of writing reliable code, and balancing test types according to the testing pyramid is crucial.
- Setting up a robust testing environment with the right tools and configurations is essential for Node.js and TypeScript projects, integrating CI/CD for efficiency.
- Advanced testing techniques, including mocking dependencies and handling asynchronous code, are key for comprehensive and maintainable unit tests.
- Writing testable code and organizing test suites efficiently are best practices that foster confidence in unit testing within development teams.
- Continuous learning from community knowledge sharing and staying updated with the latest testing techniques are vital for maintaining testing excellence.
Understanding the Fundamentals of Unit Testing
Defining Unit Testing and Its Importance
Unit testing is a foundational practice in software development, focusing on the smallest parts of an application—individual units or components. Unit tests are designed to validate that each unit of the software performs as expected. By isolating each part and testing it rigorously, developers can ensure that the software application meets its functional requirements and operates correctly.
The importance of unit testing cannot be overstated. It serves as an early detection system for defects, which can be more easily and cost-effectively resolved at this level. Here are some key advantages of unit testing:
- Early bug detection before they escalate into larger issues
- Prevention of new bugs when changes are made to the code
- Enhancement of code modularity, making it more understandable and maintainable
- Improvement of overall software quality and reliability
Unit testing is often contrasted with integration testing, which examines the interactions between units. While integration tests are crucial for ensuring that different parts of the system work together seamlessly, unit tests are invaluable for verifying the functionality of individual components without the complexity of the entire system.
Exploring the Testing Pyramid: Balancing Test Types
The testing pyramid is a model that helps developers ensure a balanced distribution of test types within their suite. At the base are unit tests, which are numerous and focused on individual components. As we move up, integration tests verify the interactions between components, and at the top, end-to-end tests assess the system as a whole.
While the pyramid serves as a guide, it’s not the only perspective. Alternatives like the testing quadrants consider the audience of the test—whether it’s technology-facing or business-facing. This approach emphasizes who gains confidence from the test’s results.
Adhering to the testing pyramid can lead to a more efficient testing strategy, optimizing for speed, reliability, and cost. However, it’s important to remain flexible and adapt your approach based on the project’s needs, possibly integrating ideas from both the pyramid and quadrants to create a comprehensive testing strategy.
Unit Testing Principles: The Foundation of Reliable Code
Unit testing principles are the bedrock upon which reliable and maintainable code is built. By adhering to these principles, developers can ensure that their unit tests are not only effective at catching bugs but also contribute to the overall quality of the software. Unit testing is an essential part of software development that helps to ensure the quality and reliability of the software by identifying errors early in the development cycle.
When designing code, it’s crucial to keep testability in mind. Aim for modular and loosely coupled code that can be easily tested in isolation. This approach simplifies the creation of unit tests and makes them more robust. The SOLID principles provide a valuable framework for writing quality code that is easier to test and maintain. Each principle contributes to a codebase that is more flexible and amenable to testing:
- Single responsibility: Each module or class should have one reason to change.
- Open-closed: Software entities should be open for extension but closed for modification.
- Liskov substitution: Objects should be replaceable with instances of their subtypes without altering the correct functioning of the system.
- Interface segregation: Many client-specific interfaces are better than one general-purpose interface.
- Dependency inversion: Depend on abstractions, not on concretions.
It’s also important to start small, especially if you’re new to unit testing. Begin with simple functions or components and gradually build up your suite of tests. This incremental approach helps in understanding the tools and techniques while building confidence in unit testing practices. Remember, unit tests are inherently tied to the code’s implementation, and changes in the codebase will require corresponding updates to the tests. This interdependence underscores the importance of maintaining a clean and well-structured codebase for both development and testing.
Setting Up a Robust Testing Environment
Choosing the Right Tools: Jest and Others
Selecting the appropriate unit testing framework is crucial for the success of your testing strategy. Jest stands out as a popular choice among JavaScript developers due to its ease of use and comprehensive feature set. It is well-documented and supported, with a wealth of resources available to help you get started and troubleshoot any issues.
Jest’s simple API and out-of-the-box assertions make it a go-to option for many. However, it’s important to consider other frameworks that might suit your project’s specific needs. Here’s a comparison of some widely-used JavaScript unit testing frameworks as of 2024:
- Jest: Known for its rich set of matchers and seamless integration with JS/TS ecosystems.
- Mocha: Flexible and modular, with a focus on simplicity.
- Storybook: Ideal for UI component testing and visualization.
- Cypress: Excellent for end-to-end testing with a real browser environment.
- Jasmine: Behavior-driven development framework with a clean syntax.
- Puppeteer: Provides a high-level API to control Chrome or Chromium over the DevTools Protocol.
- Testing Library (react): Encourages good testing practices for React components.
- Webdriver io: A custom implementation for Selenium’s WebDriver API.
- Playwright: Enables reliable cross-browser end-to-end testing.
- Ava: Minimalistic with a focus on parallel test execution.
- Vitest: A Vite-native test runner with a Jest-compatible API.
While Jest is a strong contender, evaluating each framework’s features and community support will help you make an informed decision tailored to your project’s requirements.
Configuring Your Testing Framework for Node.js and TypeScript
Setting up a robust testing environment for Node.js and TypeScript applications can be a daunting task, especially when integrating a testing framework like Jest. Understanding the prerequisites is crucial before diving into the configuration process. Ensure that Node.js, npm (or Yarn), and TypeScript are installed on your machine.
The configuration involves a series of steps that are essential for a smooth testing experience. Here’s a quick rundown:
- Initialize a new Node.js project with
npm init
oryarn init
. - Install Jest using
npm install --save-dev jest
or the equivalent Yarn command. - Configure TypeScript for Jest by adding a
jest.config.js
file to your project. - Set up Babel (if needed) to transpile TypeScript code by installing the appropriate Babel presets.
- Write a simple test to verify that Jest is working correctly with your TypeScript setup.
By following these steps, you’ll be well on your way to writing and running unit tests that help ensure the reliability and quality of your code.
Integrating Continuous Integration and Deployment (CI/CD) with Unit Tests
Integrating unit tests into the CI/CD pipeline is a strategic move that ensures code quality and stability throughout the development lifecycle. Automated unit tests are executed as part of the continuous integration process, providing immediate feedback on the impact of code changes.
The integration of unit testing with CI/CD can be broken down into several key steps:
- Setup: Configure the CI server to run unit tests automatically upon each commit.
- Execution: Ensure tests are run in an environment that closely mirrors production.
- Reporting: Generate and review test reports to identify failures quickly.
- Action: Implement a process for addressing test failures, which may include halting the deployment pipeline.
By following these steps, developers can catch and resolve issues early, leading to a more efficient development process and a higher quality product. The role of unit testing in this context is to serve as a first line of defense against bugs and regressions, making it an indispensable part of the CI/CD strategy.
Advanced Testing Techniques
Mocking Dependencies for Isolated Testing
In the realm of unit testing, mocking dependencies is essential for achieving test isolation, which is critical for reliable and deterministic test outcomes. By using mocks, you can simulate the behavior of external systems, ensuring that your tests focus solely on the code being tested. This technique is particularly useful when dealing with unpredictable or uncontrollable external systems like databases or APIs.
When deciding whether to mock a dependency, consider the potential side effects and the stability of the dependency itself. Not all dependencies need to be mocked; sometimes, using the real implementation is acceptable and can reduce the overhead of testing. However, be mindful that each unmocked dependency could introduce variability into your tests, potentially becoming a source of technical debt.
Here are some guidelines to help you determine when to mock a dependency:
- Mock external systems that are beyond your control.
- Avoid mocking if the dependency is stable and has no side effects.
- Use mocks to prevent flakiness caused by network issues or external service downtime.
- Consider the cost of maintenance for mocks versus the risk of not mocking.
Remember, the goal is to create true unit tests that are not influenced by external factors. As highlighted in the Semaphore Tutorial, learning to effectively mock dependencies is a key skill for any developer aiming to write robust unit tests.
Handling Asynchronous Code in Tests
Testing asynchronous code is a critical skill for developers working with JavaScript and its frameworks. Jest offers a variety of methods to handle asynchronous operations, ensuring tests run correctly and efficiently. One common approach is using the async/await
syntax, which simplifies writing tests by allowing you to handle promises in a way that resembles synchronous code.
For instance, when testing an asynchronous function, you can declare your test function with async
and then await
the promise. This makes the test easier to read and reason about. However, there are situations where the done()
callback is more appropriate. In such cases, Jest will wait for the done
callback to be called before considering the test complete. This is particularly useful when testing code that does not return a promise or when you need to perform multiple asynchronous operations in a single test.
Here’s a quick comparison of the two approaches:
- Async/Await: Write tests in a cleaner, more synchronous style.
- Done Callback: Useful for non-promise returning code or multiple async operations.
Remember, choosing the right approach depends on the specific needs of the code you’re testing. It’s essential to understand both methods to apply them effectively in different scenarios.
Utilizing Setup and Teardown for Maintainable Tests
In the realm of unit testing, maintaining a clean test environment is crucial for the reliability and readability of your tests. Jest, a popular testing framework, offers beforeEach()
and afterEach()
functions that serve this very purpose. These functions are called before and after each test respectively, allowing developers to prepare the necessary environment and clean up afterwards without redundancy.
For instance, you might use beforeEach()
to create a fresh instance of a database connection, and afterEach()
to close that connection. This ensures that each test runs in an isolated environment, preventing tests from affecting each other and leading to more predictable outcomes.
beforeEach()
– Prepares the environment before each test.afterEach()
– Cleans up the environment after each test.
By leveraging these methods, you can avoid common pitfalls such as flaky tests and resource leaks, which can compromise the integrity of your test suite.
Snapshot Testing with Jest: A Practical Guide
Snapshot testing is a powerful feature of Jest that allows you to compare the current output of your code against a previously saved snapshot. This method is particularly useful for testing UI components or any output that should not change unexpectedly. It’s an efficient way to ensure that your application’s user interface remains consistent after code changes or refactoring.
To implement snapshot testing in Jest, follow these simple steps:
- Write a test case that renders the component or generates the output you want to snapshot.
- Use Jest’s
toMatchSnapshot()
matcher to create a snapshot of the output. - Review the generated snapshot file to ensure it accurately represents the desired state of your output.
- Commit the snapshot file to your version control system.
- On subsequent test runs, Jest will compare the new output with the stored snapshot and report any differences.
Snapshot testing is not a silver bullet and should be used judiciously. It’s best suited for components with stable and mature UIs where changes are infrequent and need to be closely monitored. For more dynamic components, consider combining snapshot testing with other testing strategies to achieve comprehensive coverage.
Best Practices for Writing Unit Tests
Writing Testable Code: A Developer’s Responsibility
Writing testable code is not just a practice but a developer’s responsibility. It is the foundation upon which reliable, maintainable, and robust software is built. By designing code with testability in mind, developers can ensure that each unit can be tested in isolation, which is a cornerstone of unit testing.
Adhering to the SOLID principles is a strategic approach to writing testable code. These principles guide developers in creating code that is easier to understand, modify, and test. Here’s a brief overview of what each principle stands for:
- Single Responsibility: A class should have one, and only one, reason to change.
- Open/Closed: Software entities should be open for extension, but closed for modification.
- Liskov Substitution: Objects of a superclass should be replaceable with objects of a subclass without affecting the correctness of the program.
- Interface Segregation: Many client-specific interfaces are better than one general-purpose interface.
- Dependency Inversion: Depend on abstractions, not on concretions.
Embracing these principles not only aids in writing testable code but also enhances teamwork. In the absence of any team member, others can easily understand and work on the code, facilitating knowledge sharing and making the team more effective. Moreover, test-driven development (TDD) encourages writing cleaner code and reduces the time spent on debugging, ultimately benefiting the developers themselves.
Organizing Your Test Suite for Maximum Efficiency
Efficient organization of your test suite is crucial for quick feedback and maintainability. Grouping related tests using Jest’s describe
blocks can greatly enhance readability and structure. Within these blocks, individual test cases can be defined with test
or it
functions, allowing for a clear hierarchy and easy navigation.
To ensure consistency and a clean state for each test, leverage beforeEach
and afterEach
hooks to set up and tear down your test environment. This practice prevents side effects between tests and contributes to a more reliable test suite.
Adhering to the testing pyramid ensures a balanced test suite. Here’s a simple breakdown:
- Unit Tests: Verify individual units of code (most numerous)
- Integration Tests: Verify interactions between units (fewer in number)
- End-to-end Tests: Verify the entire application flow (least numerous)
By following these guidelines, you can create a test suite that is not only thorough but also optimized for speed and efficiency.
Incorporating CI/CD in Your Testing Strategy
Incorporating Continuous Integration (CI) and Continuous Deployment (CD) into your unit testing strategy is essential for maintaining a high-quality codebase. Automating the testing process using a test runner not only streamlines workflows but also provides valuable feedback on code functionality and performance.
The advantages of integrating CI/CD with unit testing are numerous. It fosters a culture of frequent code integration, where tests and code quality checks are run automatically. This practice ensures that code changes are reliable and function as expected across different environments. Moreover, continuous deployment allows for automatic code releases to production after successful test validations, leading to faster and more consistent delivery.
To effectively implement CI/CD in your testing strategy, consider the following steps:
- Utilize a CI/CD service like GitHub Actions, Travis CI, or Jenkins to automate integration and deployment processes.
- Employ a code coverage tool to measure the extent of your test coverage, ensuring that no part of the code is left untested.
- Develop an effective testing strategy with a detailed understanding of all CI/CD processes, and categorize your test cases accordingly.
By embracing these practices, you can build confidence in your unit testing and ensure that your projects remain robust and error-free.
Building Confidence in Unit Testing Within Your Team
Building confidence in unit testing is crucial for a team’s success in maintaining high-quality code. Unit tests serve as a safety net, allowing developers to refactor code with the assurance that any regressions will be caught promptly. This safety net is especially important when implementing new features or addressing technical debt.
To foster this confidence, it’s essential to understand the unit testing principles and how they contribute to a robust codebase. By focusing on unit tests that use mocks, teams can ensure that each component behaves as expected in isolation, which is a cornerstone of reliable software.
Here are some key steps to build confidence in unit testing within your team:
- Encourage learning and understanding of unit testing basics.
- Promote the practice of Test-Driven Development (TDD) for a more reliable and maintainable codebase.
- Regularly review and refactor the existing test suite to keep it efficient and relevant.
- Celebrate successes and learn from failures in unit testing to foster a positive team culture.
By integrating these practices, teams can achieve a level of confidence that enables fearless refactoring and a more collaborative approach to tackling complex coding challenges.
Continuous Learning and Improvement in Unit Testing
Learning from Open Source Projects and Peer Code Reviews
Open source projects offer a treasure trove of learning opportunities for developers at all levels. Engaging in code reviews within these projects can be particularly beneficial for junior developers. It’s a chance to see a variety of coding styles and approaches, as well as to understand the application of best practices in a real-world setting.
Participating in peer code reviews is equally instructive. It allows developers to exchange knowledge and insights, which can lead to improved coding skills and better unit tests. The process of reviewing others’ code also helps in identifying common pitfalls and learning how to avoid them.
Here are some key benefits of learning from open source projects and code reviews:
- Exposure to diverse technologies and coding techniques
- Understanding the importance of coding standards and conventions
- Gaining insights into effective problem-solving strategies
- Building a network within the developer community
Staying Updated with Latest Testing Techniques and Tools
In the ever-evolving landscape of software development, staying abreast of the latest testing techniques and tools is crucial for maintaining the effectiveness of your unit tests. As new technologies and methodologies emerge, they bring with them innovative ways to enhance testing quality and efficiency.
To ensure you’re not falling behind, consider the following strategies:
- Regularly review and assess your current testing tools against emerging options.
- Subscribe to industry newsletters, blogs, and forums that focus on software testing advancements.
- Participate in webinars, workshops, and conferences dedicated to software testing.
- Engage with open-source communities to learn from collective experiences and contributions.
By actively seeking out new information and continuously integrating it into your testing practices, you can keep your skills sharp and your tests relevant. Remember, the goal is not just to adopt new tools for the sake of novelty, but to thoughtfully evaluate and embrace those that genuinely improve your testing process.
Participating in Developer Communities for Knowledge Sharing
Engaging with developer communities is a vital aspect of continuous learning in unit testing. Developer forums and online groups provide a platform for sharing experiences, seeking advice, and discussing challenges related to unit testing. These interactions can lead to a deeper understanding of best practices and common pitfalls.
Participation in communities can take various forms, from asking questions on Stack Overflow to joining discussions on Slack, Discord, or the DEV Community. Here’s a list of popular platforms where developers can connect:
- Stack Overflow: A Q&A site for professional and enthusiast programmers.
- GitHub: Offers collaboration features such as bug tracking, feature requests, task management, and wikis for every project.
- Slack & Discord: Messaging platforms that host numerous tech-related communities.
- DEV Community: An inclusive network for software developers to share and learn.
By actively contributing to these communities, developers not only receive valuable feedback but also contribute to the collective knowledge base, helping others overcome similar obstacles.
Seeking Feedback and Mentoring for Testing Excellence
The journey towards testing excellence is continuous and often benefits greatly from the insights of others. Seeking feedback and mentoring is a crucial step in refining your unit testing skills. Engage with your peers and mentors to gain fresh perspectives on your testing strategies. Rapid feedback, ideally within 24 hours, can significantly enhance the learning process and the quality of your tests.
To further your understanding and stay current with best practices, immerse yourself in educational resources. Explore a variety of materials such as articles, tutorials, and books. Analyze open source projects and the tests written by other developers to discover new approaches and solutions to common testing challenges. Here’s a simple list to guide your learning journey:
- Read and analyze code from open source projects or your team
- Participate in online forums and communities for discussion
- Attend workshops or take courses to deepen your knowledge
- Ask open-ended questions to leaders and managers for broader insights
Remember, the goal is to not only improve your own unit testing capabilities but also to contribute to the collective knowledge of your team, fostering a culture of quality and excellence in your projects.
Conclusion
As we wrap up this Programmer’s Guide to Effective Unit Testing Techniques, it’s clear that unit testing is an indispensable part of modern software development. By embracing the practices and strategies discussed, from starting small and simple to mastering advanced techniques, you can significantly enhance the quality and reliability of your code. Remember, the journey to effective unit testing is continuous and requires dedication to learning and improvement. Whether you’re a novice or an experienced developer, there’s always room to refine your approach and integrate new methods into your workflow. Keep exploring, asking questions, and sharing knowledge within the community, and you’ll find that your unit testing skills—and your code—will only get better with time.
Frequently Asked Questions
What is unit testing and why is it important?
Unit testing is a software development practice that involves testing individual units of code, such as functions or methods, to ensure their correctness. It is important because it helps identify and fix bugs early in the development process, ensuring the reliability and quality of the code.
How can I get started with unit testing if I’m new to it?
Start small and simple by testing basic functions or components. Get familiar with your testing tools and techniques, and build your confidence and skills gradually. Following the testing pyramid concept can also help optimize the distribution of different test types.
What are some advanced unit testing techniques?
Advanced techniques include mocking dependencies for isolated testing, handling asynchronous code, using setup and teardown methods for cleaner tests, and leveraging snapshot testing features like those offered by Jest.
How do I foster confidence in unit testing within my team?
Fostering confidence involves practicing writing testable code, organizing test suites efficiently, incorporating CI/CD in your testing strategy, and ensuring that the team understands the importance and contribution of each test to the overall code quality.
What are the best practices for writing unit tests?
Best practices include writing testable code, organizing your test suite for maximum efficiency, integrating continuous integration and deployment (CI/CD) with your tests, and building a culture that values unit testing within your team.
How can I continue learning and improving my unit testing skills?
To improve your unit testing skills, engage in continuous learning by reading articles, watching tutorials, participating in workshops, reviewing open source projects, seeking peer feedback, and joining developer communities for knowledge sharing.