The Ultimate Guide to Unit Testing Software: Best Practices and Tools
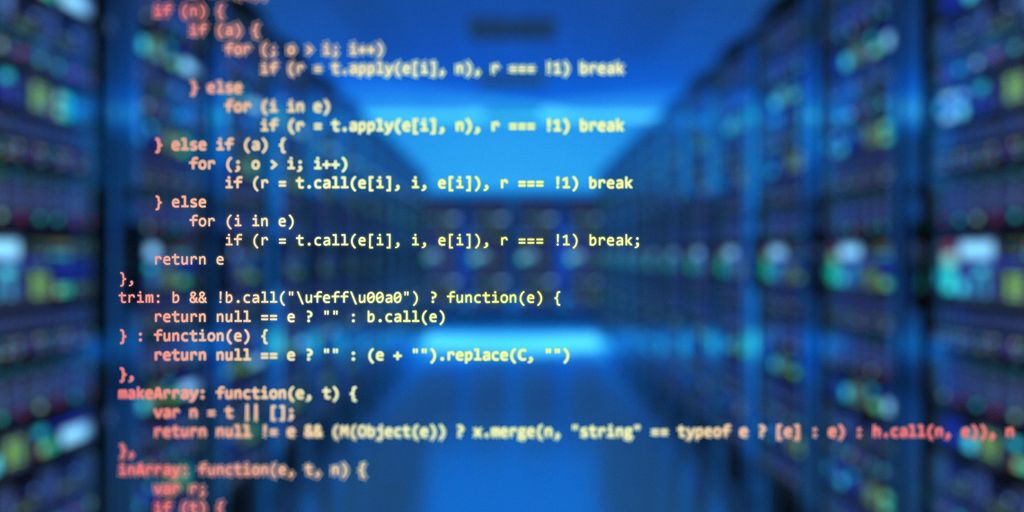
Unit testing is an indispensable technique in software development, ensuring that individual components of an application function correctly in isolation. This guide will delve deep into the essentials of unit testing, covering everything from the basics and best practices to the tools necessary for effective implementation. By understanding and applying these practices, developers can significantly enhance the quality and reliability of their software.
Key Takeaways
- Understand the fundamental concepts and importance of unit testing in software development.
- Select and configure the appropriate tools to create an efficient unit testing environment.
- Learn to write effective unit tests that cover various scenarios, including edge cases and exceptions.
- Adopt best practices to improve the readability, maintainability, and effectiveness of your unit tests.
- Explore advanced unit testing techniques and tools to further enhance your testing strategy.
Understanding Unit Testing Software
Definition and Fundamentals
Unit testing is a form of software testing where individual components, or units, of a program are tested in isolation to ensure they function correctly. This method is crucial for verifying the behavior of the smallest parts of an application, such as functions or methods.
Importance in Software Development
Unit testing plays a pivotal role in software development, enhancing code quality and reliability. It allows developers to identify and fix bugs early in the development process, leading to more stable and maintainable software.
Common Misconceptions
Many believe that unit testing is too time-consuming or only suitable for large-scale applications. However, it is essential for any software development process, regardless of the size of the project, and can significantly reduce debugging and maintenance time.
Setting Up Your Unit Testing Environment
Choosing the Right Tools
Selecting the appropriate tools is crucial for effective unit testing. Identify the tools that integrate seamlessly with your development environment and support the languages you use. Popular frameworks include JUnit for Java, NUnit for .NET, and Jest for JavaScript.
Configuring Your Development Environment
To configure your development environment for unit testing, ensure all necessary dependencies and plugins are installed. Set up a dedicated test directory and maintain a clean separation between production and test code. This setup helps in managing test cases and streamlines the testing process.
Integrating with Continuous Integration Systems
Integration of unit tests with CI systems like Jenkins, Travis CI, or GitHub Actions automates the testing process, ensuring that tests are run automatically with every code commit. This practice helps in identifying issues early and maintaining software quality consistently.
Writing Effective Unit Tests
Principles of Good Unit Tests
Good unit tests are the backbone of reliable software. They should be clear, concise, and focus on one functionality at a time. Here are key principles to follow:
- Independence: Tests should not rely on external conditions or the results of other tests.
- Repeatability: Ensure tests produce the same results regardless of the environment they are run in.
- Fast execution: Tests should run quickly to not slow down development cycles.
Structuring Your Tests for Maximum Coverage
To achieve comprehensive test coverage, structure your tests methodically. Start by covering the basic functionalities and gradually move to more complex scenarios. Use a table to track coverage areas:
Functionality | Basic Test | Complex Test |
---|---|---|
Function A | Done | Done |
Function B | Done | In Progress |
Handling Edge Cases and Exceptions
Handling edge cases and exceptions is crucial for robust unit tests. Ensure your tests anticipate and properly handle unusual or extreme conditions. This includes testing for null inputs, unexpected data types, and boundary conditions. List steps to handle exceptions effectively:
- Identify potential edge cases in your application.
- Write tests that specifically address these cases.
- Ensure exceptions are caught and handled gracefully, providing useful error messages.
Unit Testing Best Practices
Naming Conventions
Consistent naming conventions are crucial for maintaining the clarity and navigability of unit tests. It helps developers quickly understand what each test aims to verify. For example, using a pattern like test[Method]_[Scenario]_[ExpectedBehavior]
can be very effective.
Test Isolation Techniques
To ensure that each unit test is independent and does not share state with others, employ test isolation techniques. This practice enhances test reliability and prevents the cascading failure of tests due to shared states or data.
Ensuring Test Readability and Maintainability
Readable and maintainable tests are essential for long-term project health. Use clear, descriptive test names and keep the logic simple and focused. Refactoring tests to remain clean and straightforward as the codebase evolves is also vital for ongoing test effectiveness.
Advanced Techniques in Unit Testing
Mocking and Stubbing
Mocking and stubbing are essential techniques in unit testing that allow developers to simulate the behavior of complex components. By using mocks and stubs, testers can focus on the unit under test without relying on external dependencies. This isolation helps in achieving more accurate and reliable test results.
Parameterized Testing
Parameterized testing enables the execution of tests across various input sets, enhancing the test coverage significantly. This approach allows developers to verify the behavior of a unit for multiple scenarios using a single test method, making the tests more efficient and comprehensive.
Using Code Coverage Tools
Code coverage tools measure the percentage of code executed during tests, providing insights into untested parts of the codebase. These tools are crucial for ensuring that all critical paths are tested, which can lead to higher quality software and reduced bugs.
Choosing the Right Unit Testing Tools
Selecting the right tools for unit testing is crucial for enhancing the efficiency and effectiveness of your testing process. The choice of tools can significantly impact the productivity of developers and the quality of the final software product.
Overview of Popular Tools
There is a wide array of automated unit test software available, each with its own strengths and weaknesses. JTest and JUnit are among the most popular, providing robust frameworks and plugins that integrate seamlessly with development environments.
Comparative Analysis
When comparing unit testing tools, consider factors such as integration capabilities, ease of use, and the support for different programming languages. Tools like Parasoft Jtest offer comprehensive features that automate many aspects of unit testing, allowing developers to focus more on business logic.
Tool Selection Criteria
To choose the right unit testing tool, it’s essential to evaluate your specific needs and the features offered by each tool. Factors to consider include the tool’s compatibility with your development environment, the quality of documentation and community support, and the ability to scale with your project’s needs.
Troubleshooting Common Unit Testing Issues
Debugging Failing Tests
When unit tests fail, it’s crucial to quickly identify the root cause. Start by isolating the test to see if the issue is with the test itself or the code it covers. Use debuggers or detailed logs to trace the execution flow and pinpoint where things go wrong. Ensure that each test case covers a specific aspect of the unit’s functionality to make debugging more manageable.
Optimizing Test Performance
Unit tests should be fast to maintain productivity. If tests are slow, consider optimizing the test environment or the tests themselves. Techniques include minimizing database interactions, mocking external services, and running tests in parallel. Remember, the goal is to make tests both thorough and efficient.
Dealing with Flaky Tests
Flaky tests that pass and fail intermittently can undermine confidence in the testing process. To address flakiness, ensure tests are isolated and do not depend on external states. Use retries only as a last resort, and focus on making each test deterministic. This approach helps in maintaining clarity of scope and objectives.
Conclusion
In this ultimate guide to unit testing, we have navigated through the essential practices and tools that enhance the effectiveness and reliability of software development. By embracing these best practices, developers can ensure that each unit of software performs as expected, leading to more robust and maintainable codebases. The tools discussed provide the necessary support to automate and streamline the testing process, making it an integral part of the development lifecycle. As unit testing continues to evolve, staying informed and adaptable will be key to leveraging its full potential for improving software quality.
Frequently Asked Questions
What is unit testing?
Unit testing is a software testing method where individual units or components of a software are tested to ensure they are fit for use. It primarily focuses on verifying the correctness of each unit of the code.
Why is unit testing important in software development?
Unit testing is crucial because it helps catch bugs early in the development cycle, enhances code quality, and improves maintainability. It also supports agile practices by allowing for changes without breaking functionality.
What are some common misconceptions about unit testing?
Common misconceptions include the belief that unit testing is too time-consuming, it’s only for large projects, or that it can completely eliminate the need for other types of testing.
How do I choose the right unit testing tools?
Choosing the right unit testing tool depends on the project’s language, the complexity of the tests, integration capabilities with other tools, and the specific needs of the development team.
What are the best practices for writing effective unit tests?
Best practices include writing clear and concise tests, ensuring tests are independent and repeatable, covering both positive and negative scenarios, and maintaining tests alongside the codebase.
How can I handle edge cases and exceptions in unit tests?
Handling edge cases and exceptions involves writing additional tests that specifically target these scenarios. It’s important to anticipate and simulate various unusual or extreme conditions to ensure robustness.