The Ultimate Guide to Unit Testing: Techniques and Strategies
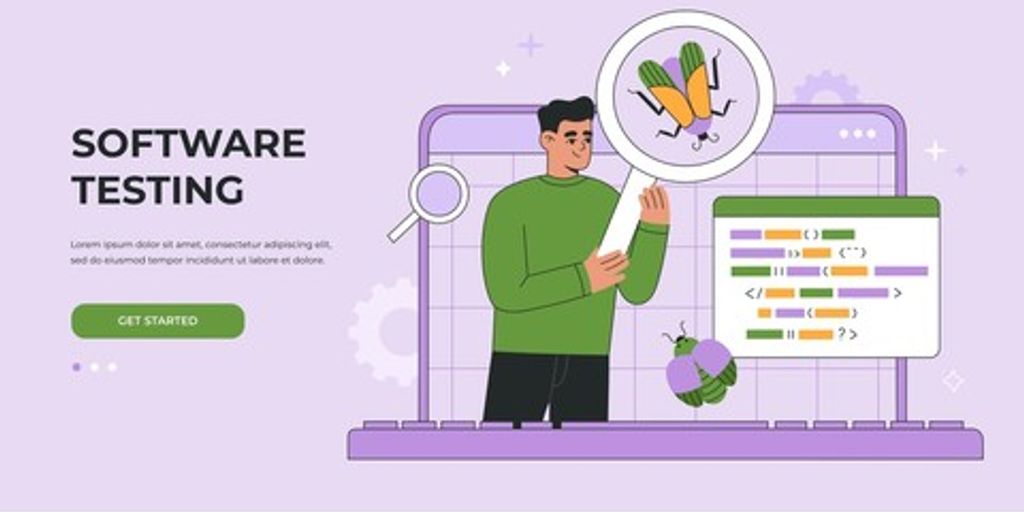
Unit testing is a key part of making sure software works right. It means checking small parts of a program to see if they do what they are supposed to do. This guide will help you understand unit testing, learn different ways to do it, and find the best tools and practices.
Key Takeaways
- Unit testing helps find problems early in the software development process.
- There are different ways to do unit testing, like black box, white box, and gray box testing.
- Writing code that is easy to test makes unit testing more effective.
- Using the right tools and frameworks can make unit testing easier.
- Unit testing is important for keeping software working well over time.
Understanding Unit Testing
Definition and Importance
Unit testing is a fundamental aspect of software testing where individual components or functions of a software application are tested in isolation. This practice ensures that each part of the software works correctly on its own. By catching bugs early, unit testing can save a lot of time and costs before integration testing.
Key Concepts and Terminology
Understanding unit testing involves knowing several key concepts:
- Test Case: A set of conditions or variables used to determine if a unit of code works as expected.
- Assertions: Statements that check if the test results match the expected outcomes.
- Test Suite: A collection of test cases that are intended to be used together.
- Mocking: Creating fake objects or functions to simulate the behavior of real ones.
Common Misconceptions
There are several common misconceptions about unit testing:
- Unit testing is time-consuming: While it may seem like it takes extra time, it actually saves time by catching bugs early.
- Only testers should write unit tests: Developers should also write unit tests to ensure their code works correctly.
- Unit tests are only for new code: Even legacy code can benefit from unit tests to ensure it continues to work as expected.
Unit Testing Techniques
Black Box Testing
Black Box Testing is a method where the tester evaluates the functionality of the software without looking at the internal code structure. This technique focuses on input and output. The tester provides input and checks if the output matches the expected result. This method is useful for validating user requirements and ensuring the software behaves as expected.
White Box Testing
White Box Testing, also known as structural testing, involves testing the internal structures or workings of an application. The tester needs to have knowledge of the code to design test cases. This technique helps in identifying hidden errors and optimizing code. It ensures that all paths through the code are tested, making it a thorough approach.
Gray Box Testing
Gray Box Testing is a combination of Black Box and White Box Testing. The tester has partial knowledge of the internal workings of the application. This technique allows for a more comprehensive testing approach, as it combines the benefits of both Black Box and White Box Testing. It helps in identifying issues that might not be visible through either technique alone.
Best Practices for Effective Unit Testing
Writing Testable Code
To write effective unit tests, you need to write code that is easy to test. This means keeping your functions small and focused on a single task. Avoid complex dependencies and tightly coupled code. Use interfaces and dependency injection to make your code more modular and easier to test.
Maintaining Test Suites
A well-maintained test suite is crucial for long-term success. Regularly review and update your tests to ensure they remain relevant as your codebase evolves. Remove outdated tests and add new ones as needed. Consistency in naming conventions and test structure can make your test suite easier to manage.
Mocking and Stubbing
Mocking and stubbing are techniques used to isolate the unit of code being tested. Mocks simulate the behavior of real objects, while stubs provide predefined responses. Use these techniques to test your code in isolation, without relying on external systems or dependencies. This helps you focus on the specific behavior you want to verify.
Tools and Frameworks for Unit Testing
Popular Unit Testing Frameworks
Unit testing has come a long way from being a manual and tedious task. Today, test automation has revolutionized the process, making it faster and more efficient. Here are some of the most widely used frameworks for unit testing:
- JUnit: A popular framework for Java applications.
- NUnit: Commonly used for .NET applications.
- TestNG: Inspired by JUnit but with more features.
- PHPUnit: Designed for PHP applications.
- Mockito: A framework for creating mock objects in Java.
- Karma: A test runner for JavaScript.
Choosing the Right Tool
Selecting the right unit testing tool depends on various factors such as the programming language, the complexity of the project, and the specific needs of your team. Here are some points to consider:
- Language Compatibility: Ensure the tool supports the programming language you’re using.
- Ease of Use: The tool should be easy to set up and use.
- Community Support: A tool with a large community can offer better support and more resources.
- Integration: Check if the tool integrates well with other tools in your development pipeline.
Integration with CI/CD Pipelines
Integrating unit testing tools with Continuous Integration and Continuous Deployment (CI/CD) pipelines is crucial for maintaining code quality. This integration allows for automatic testing whenever code changes are made, ensuring that new code does not break existing functionality. Popular CI/CD tools like Jenkins, Travis CI, and CircleCI support integration with most unit testing frameworks, making it easier to automate the testing process.
By leveraging these tools and frameworks, you can significantly improve the efficiency and effectiveness of your unit testing efforts.
Challenges and Solutions in Unit Testing
Dealing with Legacy Code
Working with legacy code can be tough. Often, this code lacks tests and is hard to change. Effective strategies for successful unit test adoption include starting with small, incremental changes and writing tests for new code first. This way, you can gradually improve the test coverage without overwhelming the team.
Handling Dependencies
Dependencies can make unit testing tricky. Mocking and stubbing are common techniques to handle this. By creating fake versions of dependencies, you can test your code in isolation. This ensures that your tests are reliable and not affected by external factors.
Ensuring Test Coverage
Ensuring that your tests cover all parts of the code is crucial. Tools like code coverage analyzers can help you see which parts of your code are tested and which are not. Aim for high coverage, but remember that 100% coverage is not always necessary. Focus on critical parts of the code first.
To sum up, unit testing comes with its own set of challenges, but with the right strategies, you can overcome them and ensure smoother framework updates.
Advanced Unit Testing Strategies
Test-Driven Development (TDD)
Test-Driven Development, or TDD, is a method where you write tests before writing the actual code. This approach helps ensure that your code meets the requirements from the start. By writing tests first, you can catch errors early and make your code more reliable. TDD follows a simple cycle: write a test, make it pass, and then refactor the code. This cycle helps keep the code clean and maintainable.
Behavior-Driven Development (BDD)
Behavior-Driven Development, or BDD, extends TDD by focusing on the behavior of the application. In BDD, tests are written in a way that describes the expected behavior of the system. This makes it easier for non-developers to understand what the tests are doing. BDD encourages collaboration between developers, testers, and business stakeholders to ensure that everyone is on the same page.
Continuous Testing
Continuous Testing is the practice of running tests automatically as part of the software delivery pipeline. This helps catch issues as soon as they are introduced, making it easier to fix them. Continuous Testing is essential for maintaining high-quality software in fast-paced development environments. It integrates with Continuous Integration and Continuous Deployment (CI/CD) pipelines to provide quick feedback on the health of the codebase.
Conclusion
Unit testing is a key part of making sure software works well. By using the right techniques and strategies, you can catch bugs early and save time in the long run. Remember to follow best practices, like writing clear and simple tests, and always keep your code clean. With these tips, you’ll be on your way to becoming a unit testing pro. Happy testing!
Frequently Asked Questions
What is unit testing?
Unit testing is a way to check if small parts of a program, called units, work correctly. It’s like testing each Lego piece to make sure it fits before building a big structure.
Why is unit testing important?
Unit testing helps catch bugs early, saves time in the long run, and makes the code easier to understand and maintain. It’s like checking your homework for mistakes before turning it in.
What are the common techniques in unit testing?
Some common techniques include Black Box Testing, White Box Testing, and Gray Box Testing. Each method has its own way of checking if the code works as expected.
How do I write testable code?
To write testable code, keep your functions small and focused on one task. Avoid using global variables and write code that is easy to read and understand.
What tools can I use for unit testing?
There are many tools available like JUnit for Java, NUnit for .NET, and Mocha for JavaScript. Choose a tool that fits well with the programming language you’re using.
What is Test-Driven Development (TDD)?
Test-Driven Development is a way of writing code where you write tests first and then the code to pass those tests. It’s like setting a goal and then working to achieve it.