Unit Test: What to Test and How to Ensure Quality
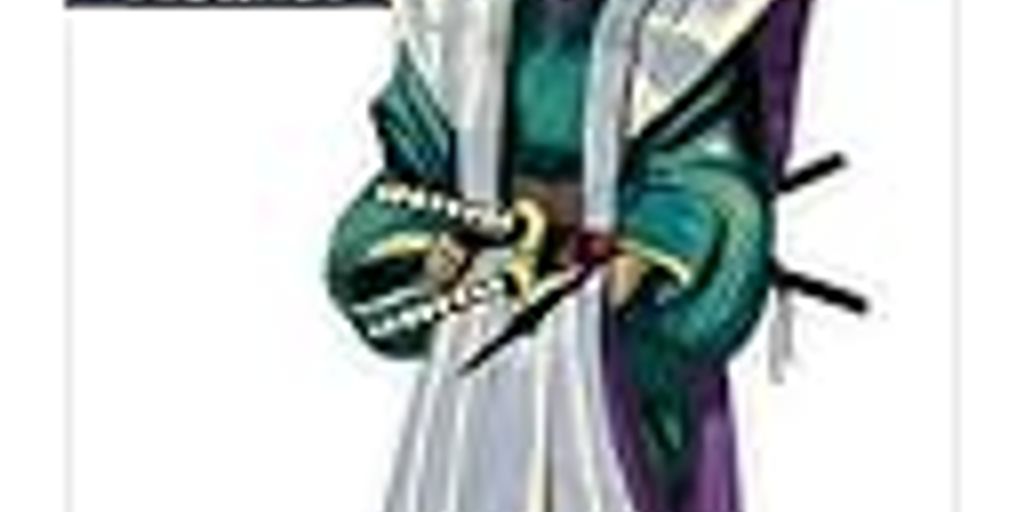
Unit testing is a key step in making sure your code works well and is reliable. It helps developers check that each part of their code does what it’s supposed to do. But it’s not just about writing tests; it’s about writing good tests that are easy to keep up with and understand. This article will walk you through the basics of unit testing, what makes a good test, and how to make sure your tests are top-notch.
Key Takeaways
- Unit testing helps ensure each part of your code works correctly.
- Good unit tests are easy to read, understand, and maintain.
- Testing should cover all possible cases, including edge cases and errors.
- Using tools and frameworks can make unit testing easier and more efficient.
- Regular code reviews and continuous integration can improve the quality of your unit tests.
Understanding the Basics of Unit Testing
Defining a Unit
When we talk about unit testing, the first question to ask is, "What is a unit?" A unit is often defined as the smallest piece of code that makes sense to test. In object-oriented programming, this could be a whole class or an interface. In functional programming, it might be a single function or method. The goal is to test these units in isolation to ensure they work as expected.
Importance of Unit Testing
Unit testing is a crucial part of the software development process. It is the first level of testing and is done before integration testing. Think of it as a safety net that catches defects early, ensuring that individual components function correctly. This early detection paves the way for smoother subsequent testing stages. By catching issues early, unit testing helps maintain code quality and reduces the cost and effort of fixing bugs later in the development cycle.
Characteristics of High-Quality Unit Tests
Readability and Understandability
A good unit test should be easy to read and understand. Clear and descriptive names for tests help others know what the test is checking. This makes it easier to maintain and update tests over time.
Isolation of Dependencies
Unit tests should test only one piece of code at a time. This means isolating the code from other parts of the system. Using techniques like mocking and stubbing can help achieve this isolation.
Speed and Efficiency
High-quality unit tests run quickly. They should provide fast feedback so developers can catch issues early. Efficient tests help keep the development process smooth and productive.
What to Test in Unit Tests
Functional Verification
When writing unit tests, the first thing to check is if the code does what it’s supposed to do. This means making sure the function or method works correctly with the given inputs. Unit tests are typically automated and written by developers using various frameworks such as JUnit, NUnit, or PyTest. These tests validate the correctness of the code by comparing the actual output with the expected output.
Edge Cases and Boundary Conditions
It’s important to test how your code handles unusual or extreme inputs. These are called edge cases and boundary conditions. For example, if you’re testing a function that adds two numbers, you should also test what happens when you add very large numbers or very small numbers. This helps ensure that your code doesn’t break when it encounters unexpected inputs.
Error Handling
Another key aspect to test is how your code deals with errors. This includes checking if the code properly handles invalid inputs or unexpected situations. By writing tests for error handling, you can make sure that your code fails gracefully and provides useful error messages when something goes wrong.
Best Practices for Writing Unit Tests
Test-Driven Development
Test-Driven Development (TDD) is a practice where you write tests before writing the actual code. This approach ensures that your code meets the requirements from the start. TDD helps in creating a clear and concise design and makes it easier to identify issues early in the development process.
Mocking and Stubbing
Mocking and stubbing are techniques used to isolate the unit being tested by replacing dependencies with controlled substitutes. This ensures that tests are not affected by external factors and can focus solely on the functionality of the unit. Use mocking frameworks to simplify this process and make your tests more reliable.
Ensuring Complete Coverage
To ensure that your unit tests are effective, aim for complete coverage of your code. This means testing all possible paths, including edge cases and error conditions. Use code coverage tools to identify untested parts of your code and write additional tests to cover them. Complete coverage helps in catching hidden bugs and ensures that your code is robust and reliable.
Common Pitfalls and How to Avoid Them
Over-Coupling to Code Internals
One common pitfall in unit testing is over-coupling tests to implementation details. When tests are too closely tied to the internal workings of the code, even minor changes can cause them to fail. This makes maintaining the tests a burden. To avoid this, focus on testing the behavior and outcomes rather than the specific implementation.
Ignoring Edge Cases
Another frequent mistake is ignoring edge cases. It’s crucial to test for all possible scenarios, including those that are less likely to occur. By doing so, you can identify problem areas in your code before they become an issue. This goes a long way towards promoting better development practices.
Neglecting Test Maintenance
Tests, like production code, require regular maintenance. Neglecting this can lead to brittle tests that break easily. To prevent this, regularly review and update your tests to ensure they remain relevant and effective. Recognize test setup pain as a smell; if setting up tests becomes cumbersome, it might be a sign that your design needs re-evaluation.
Tools and Frameworks for Unit Testing
Popular Unit Testing Frameworks
Several frameworks and tools can assist in the unit testing process. Here are a few commonly used ones:
- JUnit: One of the most popular unit testing frameworks for Java. It is easy to use and provides annotations to identify test methods, setup and teardown procedures, and test cases. JUnit also integrates well with popular development environments and build tools.
- NUnit: A unit-testing framework for all .Net languages. Initially ported from JUnit, NUnit has grown and evolved into a powerful tool in its own right, with features such as custom attributes to support complex testing scenarios, and strong support for data-driven tests.
- unittest: A built-in unit testing framework for Python. It supports test automation, sharing of setup and shutdown code for tests, aggregation of tests into collections, and independence of the tests from the reporting framework.
Choosing the Right Tools
Selecting the right unit testing tools depends on several factors, including the programming language, the development environment, and specific project needs. Consider the following criteria when choosing a tool:
- Language Compatibility: Ensure the tool supports the programming language used in your project.
- Integration: Check if the tool integrates well with your development environment and build tools.
- Community and Support: A tool with a strong community and good support can be very helpful.
- Features: Look for features that match your testing requirements, such as support for data-driven tests or custom attributes.
Integrating with CI/CD Pipelines
Integrating unit testing tools with Continuous Integration/Continuous Deployment (CI/CD) pipelines is crucial for maintaining code quality. This integration allows for automatic running of tests whenever code changes are made, ensuring that new code does not break existing functionality. Key steps to integrate unit testing with CI/CD pipelines include:
- Set Up the CI/CD Pipeline: Configure your CI/CD tool (like Jenkins, GitLab CI, or GitHub Actions) to run unit tests as part of the build process.
- Automate Test Execution: Ensure that tests are automatically executed on code commits or pull requests.
- Monitor Test Results: Regularly check test results and address any failures promptly.
- Maintain Test Scripts: Keep your test scripts up to date with code changes to ensure they remain effective.
Ensuring Quality in Unit Testing
Code Reviews and Pair Programming
Code reviews and pair programming are essential practices for maintaining high-quality unit tests. Code reviews help catch mistakes and ensure that tests are thorough and accurate. Pair programming, where two developers work together on the same code, can also improve the quality of unit tests by combining different perspectives and expertise.
Continuous Integration
Continuous Integration (CI) is a practice where code changes are automatically tested and integrated into the main codebase. This ensures that unit tests are run frequently, catching issues early. CI helps maintain a stable codebase and reduces the risk of bugs slipping through.
Metrics and Code Coverage
Metrics and code coverage tools provide insights into how much of the code is being tested. High code coverage indicates that a large portion of the codebase is covered by tests, reducing the risk of untested code causing issues. However, it’s important to focus on the quality of tests, not just the quantity. Aim for meaningful tests that cover critical paths and edge cases.
Conclusion
Unit testing is a cornerstone of creating reliable and maintainable software. By focusing on writing high-quality tests, developers can ensure their code works as intended and is easier to improve over time. Remember, good unit tests act as a safety net, catching bugs early and preventing future issues. They also serve as living documentation, helping others understand how the code is supposed to work. As you continue to write and refine your unit tests, keep these principles in mind to maintain a robust and efficient codebase.
Frequently Asked Questions
What is unit testing?
Unit testing is when you check small parts of your code to make sure they work right. It’s like making sure each Lego piece fits before building the whole set.
Why is unit testing important?
Unit testing helps catch bugs early, makes your code easier to understand, and helps keep everything working when you make changes.
What makes a good unit test?
A good unit test is easy to read, runs quickly, and tests just one thing at a time. It should also work the same way every time you run it.
How do I know what to test in my unit tests?
You should test the main functions of your code, check for edge cases, and make sure your code handles errors well.
What are some best practices for writing unit tests?
Some best practices include writing tests before you write the code, using mocks and stubs to isolate parts of your code, and making sure you test everything important.
What are common mistakes to avoid in unit testing?
Avoid making tests that are too tied to the way your code is written, forgetting to test edge cases, and not keeping your tests up to date.