Unit Testing Testing: Ensuring Code Quality and Reliability
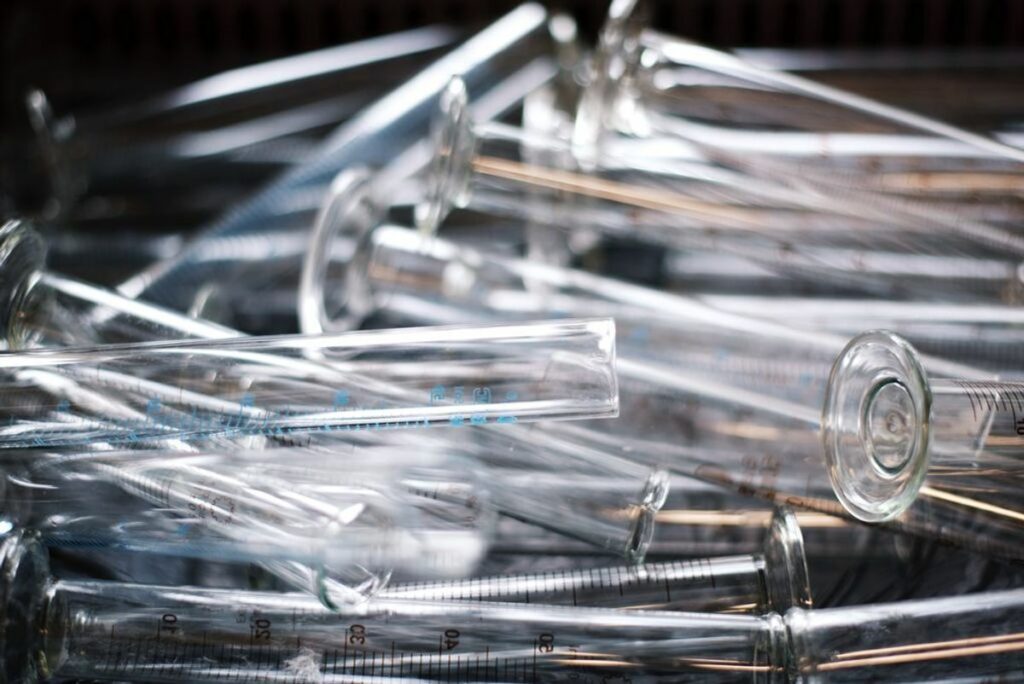
Unit Testing is an indispensable aspect of software development, aimed at validating the smallest components of an application—its units. It’s a practice that not only catches bugs early but also underpins the overall code quality and maintainability. By dissecting the development process into testable chunks, Unit Testing ensures that each part of the code performs as intended, which is crucial for the reliability of the entire system. This article delves into the intricacies of Unit Testing, exploring its fundamental principles, its role in enhancing code quality, its interplay with Test-Driven Development (TDD), best practices for implementation, and advanced techniques to further refine the testing process.
Key Takeaways
- Unit Testing is a core practice for verifying the functionality of code at the unit level, enhancing reliability and maintainability.
- Adherence to Unit Testing promotes writing modular, loosely coupled code and functions with single responsibilities, leading to improved code quality.
- Integrating Unit Testing within Test-Driven Development (TDD) facilitates quick feedback, iterative improvement, and code adaptability.
- Effective Unit Testing involves creating a comprehensive test suite, automating tests, and ensuring thorough coverage with continuous integration.
- Advanced Unit Testing techniques, such as mocking and leveraging code analysis tools, complement traditional testing and contribute to a robust software product.
The Fundamentals of Unit Testing
Defining Unit Testing and Its Purpose
Unit testing is a foundational practice in software development, focusing on the verification of individual components, or ‘units’, of code. The primary goal of unit testing is to validate that each unit performs as expected in isolation. This process involves creating and running test cases that check the functionality of functions, methods, or classes against a set of predefined conditions.
The benefits of unit testing are multifold, contributing to the overall quality and reliability of software. By isolating each part of the code and testing it thoroughly, developers can catch and rectify errors early in the development cycle. This proactive approach to error detection is crucial for maintaining a robust codebase. Below is a list of key purposes served by unit testing:
- Ensures individual code units function correctly.
- Facilitates the identification of defects at an early stage.
- Promotes better design and architecture of the code.
- Enhances code maintainability and readability.
- Simplifies the integration process by ensuring unit-level quality.
Unit testing is not just a debugging tool; it is a vital component of a disciplined software development process that leads to high-quality, reliable, and maintainable code.
The Role of Unit Tests in Software Development
Unit testing is integral to the software development lifecycle, serving as a first line of defense against bugs and errors. By focusing on the smallest testable parts of an application, unit tests ensure that each component functions as intended. This practice not only aids in bug detection but also promotes a more maintainable and robust codebase.
Developers are typically responsible for writing and executing unit tests, often using specialized testing frameworks. These tests are a form of white-box testing, where the internal structure of the unit is known to the tester, usually the developer. The process of unit testing provides quick feedback, allowing for immediate corrections and adjustments to the code.
The benefits of unit testing are numerous and can be summarized as follows:
- Early bug detection
- Facilitation of code refactoring
- Documentation of code behavior
- Simplification of the integration process
In conclusion, unit testing is a fundamental practice that enhances the quality and reliability of software. It allows developers to work with confidence, knowing that each change is verified, reducing the risk of introducing new defects into the system.
Unit Testing vs. Functional Testing: Understanding the Differences
While both unit testing and functional testing are crucial for ensuring software quality, they serve different purposes and operate at different levels of the application. Unit testing is the practice of testing individual components or units of code, such as functions, methods, or classes, in isolation. This ensures that each element performs as expected on its own. Functional testing, in contrast, evaluates the application as a whole, verifying that it behaves correctly from the user’s perspective and meets the business requirements.
The scope of testing is a key differentiator between the two. Unit tests are narrow in focus, targeting small, isolated sections of the codebase. Functional tests, however, have a broader scope, covering entire features or the system as a whole. This distinction is crucial when deciding which testing approach to use for different testing needs.
Here’s a quick comparison of the two testing methods:
- Focus: Unit testing looks at individual code elements in isolation, while functional testing examines the overall functionality from the user’s perspective.
- Concern: Unit testing deals with the internal aspects of the code, whereas functional testing addresses external aspects of the application.
- Scope: Unit testing covers a smaller portion of the codebase, whereas functional testing tests a larger portion of the system.
Improving Code Quality Through Unit Testing
Writing Modular and Loosely Coupled Code
To achieve high-quality software, it’s essential to design code that is both modular and loosely coupled. This approach not only facilitates easier unit testing but also enhances the overall maintainability and extensibility of the codebase. By adhering to the SOLID principles, developers can create systems that are more resilient to change and easier to understand.
Dependency injection is a powerful technique that supports this goal by allowing components to be more independent from one another. When dependencies are injected rather than created within the component, it becomes simpler to replace or mock these dependencies during testing. This results in a more flexible and testable code structure.
Here are some key points to consider when aiming for modularity and loose coupling:
- Design with testability in mind to facilitate effective unit testing.
- Apply the SOLID principles to improve cohesion and reduce coupling.
- Utilize dependency injection to decouple components and ease testing.
Unit Testing as a Catalyst for Single Responsibility Functions
Unit testing not only verifies the functionality of individual units of code but also promotes the design of modular, maintainable software. By focusing on testing single units, developers are encouraged to write functions that fulfill a single responsibility, adhering to one of the key principles of clean code. This practice leads to a codebase that is easier to understand, debug, and extend.
The benefits of single responsibility functions are numerous. They simplify the creation of unit tests, as each function has a clear, isolated purpose. Moreover, when a function is limited to one task, it becomes significantly easier to identify and fix defects. The table below illustrates the contrast between single and multiple responsibility functions in terms of testability and maintainability:
Function Type | Testability | Maintainability |
---|---|---|
Single Responsibility | High | High |
Multiple Responsibilities | Low | Low |
Incorporating unit tests early in the development process ensures that functions remain focused on a single task, which in turn facilitates easier maintenance and scalability. As a result, unit testing acts as a powerful tool for upholding high standards in software development, driving the creation of more reliable and robust applications.
Maintaining High Standards with Unit Testing
Unit testing is not just about catching bugs early; it’s about upholding a culture of quality within the development team. Consistent application of unit testing practices ensures that code quality remains a top priority throughout the software development lifecycle. By setting a standard for code that must pass unit tests before it is considered complete, developers are encouraged to write cleaner, more reliable code from the outset.
To maintain high standards with unit testing, consider the following best practices:
- Follow coding standards and best practices when creating unit tests to promote consistency and readability.
- Design tests for reusability and maintainability, which facilitates efficient test maintenance and minimizes redundancy.
- Implement continuous integration to receive immediate feedback on the impact of code changes.
While unit testing is a powerful tool for maintaining code quality, it is important to recognize its limitations. In cases where the codebase is frequently changing, updating unit tests can become time-consuming. For small or straightforward projects, the cost of writing and maintaining unit tests may not be justified, and a more practical approach might prioritize other testing methods to ensure overall system functionality and reliability.
Unit Testing in the Context of Test-Driven Development
The TDD Cycle: Red, Green, Refactor
The Test-Driven Development (TDD) cycle is a rigorous approach that emphasizes writing tests before code. Developers start by writing a failing test (Red), then implement the minimal amount of code to pass the test (Green), and finally refactor the code for optimization and simplicity (Refactor). This cycle ensures that code is thoroughly tested and designed with quality in mind from the outset.
Refactoring is a critical step in the TDD cycle. It involves improving the structure and clarity of the code without changing its external behavior. The presence of unit tests provides a safety net that allows developers to refactor confidently, knowing that any regressions will be caught immediately. Here are some key benefits of refactoring within the TDD cycle:
- Enhances code readability and maintainability.
- Improves performance by reducing complexity and duplication.
- Facilitates collaborative improvement among developers.
By integrating TDD with Continuous Integration (CI), developers can automate the testing process, ensuring that all changes are validated promptly. This integration leads to a more disciplined development process and a codebase that is continuously evolving without sacrificing reliability.
Quick Feedback and Iterative Improvement
The essence of quick feedback in the realm of unit testing cannot be overstated. It serves as an immediate signal to developers about the impact of their recent code changes, particularly during the refactoring phase. This rapid response mechanism is integral to the agile methodology, fostering an environment where adjustments are made swiftly and efficiently.
Incorporating unit tests into a Continuous Integration (CI) pipeline amplifies this benefit. As code is pushed to the repository, tests are automatically triggered, providing developers with instant feedback. This practice is crucial for identifying and addressing regressions or bugs early, before they escalate into larger issues.
The iterative nature of this process encourages a culture of continuous improvement. Developers are prompted to refine their code and tests in response to feedback, leading to a more robust and reliable codebase. This cycle of test, feedback, and improvement is a cornerstone of Agile development and is particularly evident in Test-driven Development (TDD) strategies.
Facilitating Refactoring and Code Adaptability
Unit testing is integral to the refactoring process, providing a robust safety net that allows developers to enhance and modify code with confidence. Refactoring aims to improve the internal structure of code without altering its external behavior, and unit tests help ensure that these internal changes do not introduce regressions.
The iterative nature of unit testing complements agile development practices, encouraging continuous code improvement. When tests are run after each refactoring step, they offer quick feedback, signaling whether the changes are successful or if further adjustments are needed. This cycle of testing and refining leads to a more adaptable and maintainable codebase.
Key benefits of unit testing in refactoring include:
- Ensuring code reliability during structural changes
- Prompt detection of regressions
- Supporting a culture of continuous code enhancement
By integrating unit testing with Continuous Integration (CI) systems, developers can automate the testing process, further streamlining refactoring efforts and maintaining high code quality throughout the development lifecycle.
Best Practices for Effective Unit Testing
Creating a Comprehensive Test Suite
A comprehensive test suite is the cornerstone of any robust unit testing strategy. It encompasses a wide range of test cases that cover various scenarios, from common use cases to edge cases and potential error conditions. Each test case should clearly describe the expected outcomes, aligning with the unit’s specifications and requirements.
To achieve this, a collaborative approach is essential. Developers should work together to identify potential test cases, including those that may not be immediately obvious. This collaboration ensures a diverse perspective on the code and its possible failure points.
Organizing test files is also crucial for maintaining a clean and modular test suite. Tests should be grouped based on the corresponding modules or components they are testing, with a clear naming convention that differentiates test files from production code. This structure not only aids in clarity but also in the maintainability of the test suite. Consider the following table for organizing test files:
Module | Test File Name | Description |
---|---|---|
User Authentication | auth.test.js |
Tests related to user login and registration |
Payment Processing | payment.test.js |
Tests for transaction handling and validation |
Data Export | export.test.js |
Tests for data export functionalities |
Furthermore, adhering to coding standards and best practices when writing tests ensures consistency, readability, and maintainability. Tests should be designed for reusability and maintainability, which not only streamlines test development but also reduces redundancy and facilitates easier updates. Continuous integration plays a pivotal role here, providing immediate feedback and ensuring that tests are run automatically with every code change.
Automating the Execution of Unit Tests
Automating unit tests is a cornerstone of modern software development, ensuring that tests are run consistently and efficiently. Automated unit tests are integral to maintaining quality and are easily integrated into continuous integration (CI) pipelines. This automation allows for quick execution of tests, which is essential for agile development and frequent code changes.
Integrating unit tests into CI/CD pipelines is not just about automation; it’s about creating a reliable validation process. Tools like Jenkins, Travis CI, or GitHub Actions can orchestrate automated test runs, with notifications set up to alert the team of any failures. This proactive approach to testing ensures that issues are addressed promptly, preventing the integration of faulty code.
Best practices for test automation include automated test generation, dependency management for isolating tests, and coverage analysis to identify untested code. These practices contribute to a robust testing strategy, optimizing resource utilization and reducing human error.
Ensuring Test Coverage and Continuous Integration
Achieving comprehensive test coverage and integrating unit tests into a Continuous Integration/Continuous Deployment (CI/CD) pipeline are pivotal for maintaining software quality. Automated unit tests play a crucial role in this process, providing consistent and repeatable validation of code changes. By automating tests, teams can improve test coverage and efficiency, ensuring that all aspects of the application are tested thoroughly.
Integrating unit tests into the CI/CD pipeline allows for immediate feedback on code changes. Tools such as Jenkins, Travis CI, or GitHub Actions can orchestrate automated test runs, with notifications alerting the team to any failures. This seamless integration is essential for preventing the introduction of defects into the main codebase and for enabling rapid issue resolution.
To further enhance test coverage, data-driven testing approaches can be utilized. These approaches involve using varied datasets to validate different scenarios, including potential edge cases, which might otherwise be missed. Running tests in parallel is another strategy that can significantly reduce execution time, making the testing process more efficient.
- Improve Test Coverage: Automate tests at different levels (unit, integration, system, acceptance) to detect defects early.
- Increase Efficiency: Parallel test execution and reduced regression testing time accelerate development.
- Enhance Quality: Early defect detection improves software quality and reduces bug-fixing costs.
Advanced Unit Testing Techniques and Tools
Mocking and Stubbing in Unit Tests
Mocking and stubbing are essential techniques in unit testing that allow developers to simulate the behavior of external dependencies. Mock objects can be created to mimic the behavior of real objects, ensuring that the unit tests can run in isolation without the unpredictability of external systems. This is particularly useful when dealing with databases, APIs, or file systems.
To effectively mock a dependency, tools like Jest provide functions such as jest.mock()
which streamline the process. For instance, Jest’s jest.fn()
can be used to create a mock function that tracks calls and return values, while jest.spyOn
allows spying on existing functions to alter their behavior during tests.
Advanced testing techniques with Jest also include testing asynchronous code and leveraging snapshot testing features. These methods enhance the robustness of unit tests, making them more comprehensive and maintainable. It’s important to note that while mocking simplifies unit testing, it should be used judiciously to avoid over-reliance on simulated behaviors that may not accurately reflect production scenarios.
Leveraging Code Analysis Tools for Better Test Cases
Code analysis tools play a pivotal role in enhancing the quality of unit tests. Automated test generation and maintenance tools can drastically reduce the effort required to write and upkeep tests. By automating the creation of test cases, developers can focus on more complex tasks while ensuring that the test suite remains robust and up-to-date.
Dependency management features in these tools allow for straightforward mocking or stubbing, which is crucial for achieving test isolation and reliability. This ensures that unit tests do not inadvertently depend on external systems or states, leading to more predictable and accurate test outcomes.
Coverage analysis is another key feature offered by code analysis tools. It provides insights into which parts of the codebase have been tested and which have not, guiding developers towards a more comprehensive testing strategy. This is essential for maintaining high standards of code excellence, as highlighted by resources like Visual Studio’s testing tools.
Here are some benefits of using code analysis tools:
- Improve Test Coverage: Ensure thorough coverage across all test levels.
- Increase Efficiency: Speed up regression testing for faster development cycles.
- Enhance Quality: Detect defects early to improve software quality and reduce bug-fixing costs.
Integrating Unit Testing with End-to-End Testing Strategies
In the realm of software testing, unit testing and end-to-end testing are complementary strategies that together ensure the highest quality and reliability of software products. Unit testing is the practice of testing individual components in isolation to ensure they function correctly on their own. On the other hand, end-to-end testing validates the entire application’s workflow from start to finish, simulating real user scenarios.
While unit tests are quick to write and execute, providing immediate feedback to developers, end-to-end tests are more comprehensive and can catch issues that unit tests might miss, especially those related to the integration of different components. It’s important to understand that neither approach should be used in isolation. A balanced testing strategy that includes both unit and end-to-end tests is crucial for delivering high-quality software.
Here are some key differences between unit and end-to-end testing:
- Unit Testing: Focuses on individual components in isolation.
- End-to-End Testing: Verifies that all components work together in real-world scenarios.
By integrating unit testing with end-to-end testing, teams can enjoy faster development times, improved code quality, and reduced maintenance costs. This integration ultimately leads to a more reliable and robust software product, ready to withstand the complexities of real-world use.
Conclusion
In conclusion, unit testing is an indispensable component of the software development lifecycle, pivotal in ensuring the quality and reliability of code. By isolating and examining the smallest parts of the application, developers can detect and rectify errors early, preventing them from escalating into more significant problems. This practice not only enhances code quality but also supports methodologies like Test-Driven Development (TDD) and simplifies maintenance by serving as a safety net during refactoring. As we’ve explored throughout this article, unit testing is not just a task to be checked off; it’s a fundamental strategy for building robust, maintainable, and high-quality software. Incorporating unit testing into your development process is a step towards more reliable, efficient, and bug-resistant applications.
Frequently Asked Questions
What is the purpose of unit testing in software development?
Unit testing is a software development practice that involves testing individual units of code, such as functions or methods, to ensure their correctness. It helps to identify and fix bugs early on in the development process, improving code reliability and making maintenance more straightforward.
How does unit testing contribute to improved code quality?
Unit testing encourages developers to write modular and loosely coupled code with a focus on single responsibilities. This leads to a more maintainable and readable codebase and upholds high code quality standards throughout the development process.
What is the difference between unit testing and functional testing?
Unit testing focuses on verifying the functionality of individual units of code to ensure their correctness and robustness. Functional testing evaluates the behavior of the entire system based on functional requirements. Together, they provide comprehensive coverage from isolated units to the integrated system.
How does unit testing support Test-Driven Development (TDD)?
In TDD, unit tests are written before the code itself. The TDD cycle of Red (write a failing test), Green (make the test pass), and Refactor (clean up the code) relies on unit testing to provide quick feedback and facilitate iterative improvement, ensuring code adaptability and maintainability.
What are some best practices for effective unit testing?
Effective unit testing involves creating a comprehensive test suite, automating test execution, ensuring high test coverage, and integrating with continuous integration systems. These practices help in identifying issues promptly and maintaining a healthy codebase.
Can you explain the role of mocking and stubbing in unit testing?
Mocking and stubbing are techniques used in unit testing to isolate the unit of code being tested by replacing dependencies with mock objects or stubs. This allows testers to verify the unit’s behavior in a controlled environment without the complexity of its dependencies.