Unraveling Test Approaches in Software Testing: Strategies and Methods
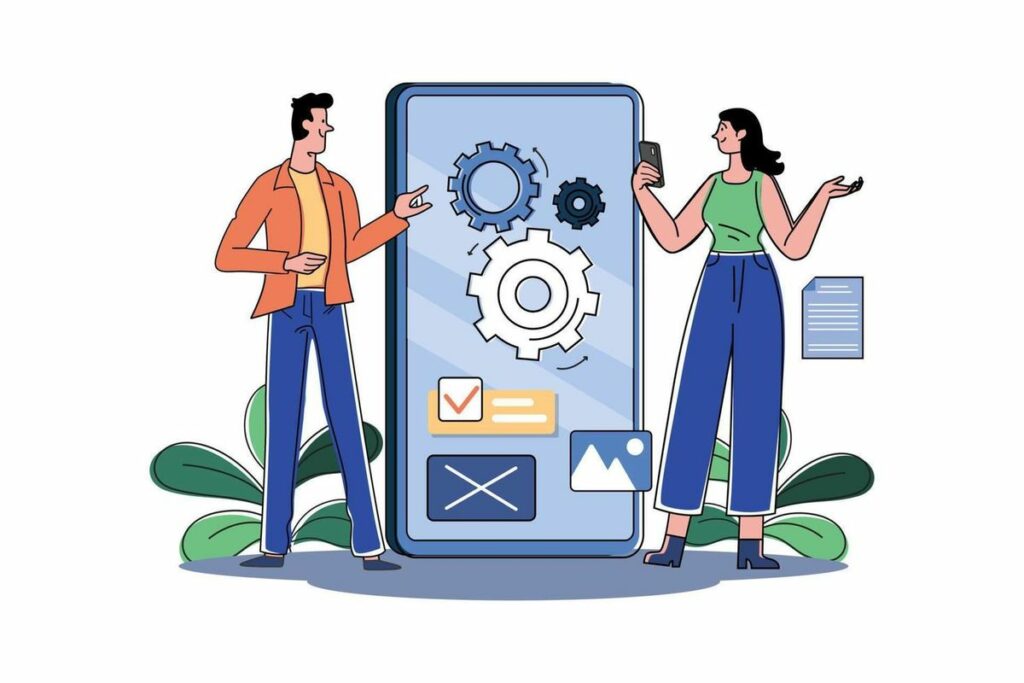
In the dynamic world of software development, testing stands as a critical phase, ensuring the reliability and quality of the final product. This article delves into various testing approaches, from the granular level of unit testing to the strategic application of probability in anticipating software risks. We explore the best practices and common pitfalls in unit testing, the theoretical and practical aspects of probability in software testing, and the efficient use of testing tools and frameworks. Additionally, we discuss advanced debugging strategies using tools like Git Bisect and the iterative improvement of test automation. Join us as we unravel the intricacies of these software testing strategies and methods.
Key Takeaways
- Unit testing is a foundational practice in software development, with tools like JUnit and Assert aiding in writing clean tests, avoiding false positives, and properly handling NullPointerException checks.
- Probability theory is a powerful ally in software testing, enabling better understanding of software behavior, optimizing test coverage, and mitigating risks through concepts like conditional probability and Bayes’ theorem.
- Choosing the right testing tools and frameworks is essential for ensuring software readiness for deployment, with a focus on tailoring to development needs and leveraging practices for thorough verification and validation.
- Advanced debugging strategies, such as the use of Git Bisect, can significantly enhance the efficiency of identifying and resolving code issues, especially when dealing with regression testing and flaky tests.
- Iterative approaches in test automation, including the application of statistical and machine learning techniques, can improve test script quality and contribute to more robust and reliable software systems.
Unit Testing: Best Practices and Pitfalls
Writing Clean and Readable Tests with JUnit and Assert
Writing clean and readable tests is crucial for maintaining and understanding test suites. JUnit and AssertJ frameworks provide powerful features to enhance test clarity. For instance, AssertJ’s fluent API allows for chaining assertions, making tests more expressive and easier to read.
To ensure tests are both clean and informative, consider the following best practices:
- Use descriptive test method names that clearly state what is being tested.
- Employ AssertJ’s fluent API for chaining assertions and producing more readable tests.
- Focus on asserting values directly rather than the results of boolean expressions.
For example, rather than asserting that a boolean expression is true, assert the expected value directly:
// Bad Practice
assertThat(argument.contains("o")).isTrue();
// Good Practice
assertThat(argument).contains("o");
By adhering to these practices, developers can create a suite of unit tests that are not only effective but also maintainable and easy to understand.
Avoiding False Positives in Unit Testing
False positives in unit testing can lead to a false sense of security and undermine the reliability of your test suite. Ensuring that each test case accurately reflects the intended behavior of the code is crucial. To avoid false positives, consider the following points:
-
Assert Values, Not Just Results: It’s important to check for specific values rather than just a pass/fail status. This approach provides more informative test failures and helps pinpoint issues.
-
Avoid Overusing NPE Checks: Non-essential
isNotNull
assertions can clutter tests and mask real issues. Only include null checks when they are relevant to the behavior being tested. -
Group Related Assertions: Organizing assertions that are related can enhance test clarity and readability. This practice also makes it easier to understand the test’s intent and the component’s behavior.
-
Chain Assertions: Utilize fluent assertion APIs like AssertJ to create more readable and maintainable tests. This can help in expressing complex assertions in a clear and concise manner.
Common Mistakes to Avoid in Unit Testing
When it comes to unit testing, one of the most common mistakes developers make is writing inadequate tests. These are tests that fail to cover all possible scenarios and edge cases, leading to a false sense of security. To ensure comprehensive test coverage, consider the following points:
- Write tests for both expected behavior and potential edge cases. This helps in uncovering issues that might only occur under specific conditions.
- Avoid overusing
isNotNull
assertions unless they are directly related to the test’s purpose. Overuse can mask real issues and lead to misleading test results. - Assert values, not just the test outcomes. Providing detailed assertions can help pinpoint the exact cause of a test failure, making debugging more straightforward.
- Group related assertions to improve test clarity and readability. This practice aids in understanding the test’s intent and the component’s behavior.
By addressing these points, developers can enhance the reliability and effectiveness of their unit tests, avoiding the pitfalls of inadequate testing.
The Role of NullPointerException (NPE) Checks in Unit Testing
In the realm of unit testing, the practice of checking for NullPointerException
(NPE) can be a double-edged sword. While it’s crucial to ensure that your code is robust against null references, overusing NPE checks in your tests can lead to bloated and less maintainable code. It’s important to remember that a unit test’s primary goal is to assert the expected behavior of a component in a clear and reliable manner, not just to avoid NPEs.
Instead of littering your tests with redundant isNotNull
assertions, focus on the actual values and behaviors that need to be verified. For instance, consider the difference between the following assertions in a test case for a getMessage
method:
-
Original assertion with unnecessary NPE check:
assertThat(service).isNotNull();
assertThat(service.getMessage()).isEqualTo("Hello world!");
-
Refined assertion without the NPE check:
assertThat(service.getMessage()).isEqualTo("Hello world!");
The latter approach not only simplifies the test but also provides a more meaningful error message in case of failure, directly pointing to the root cause. By avoiding superfluous checks, you maintain the test’s purpose and enhance its readability and maintainability.
Probability in Software Testing: A Theoretical and Practical Guide
Defining Probability in the Context of Software Testing
In the realm of software testing, probability represents the likelihood of a particular event occurring, such as executing a specific sequence of statements within our code. For instance, the probability of a function being called, or an exception being thrown, can be quantified and used to inform testing strategies.
Probability plays a crucial role in helping us understand the likelihood of certain events, like encountering specific paths within the code, and assessing the effectiveness of test coverage. It starts from a theoretical foundation and extends to practical applications in software testing.
Understanding the basics of probability is essential for optimizing testing efforts and building more reliable software. Here are some key points to consider:
- Probability helps estimate the likelihood of specific events.
- Conditional probability considers the influence of one event on another.
- Bayes’ theorem offers a framework for updating probabilities based on new information.
Applying Conditional Probability to Test Coverage
In the realm of software testing, conditional probability is a powerful tool that refines our understanding of test coverage. It allows us to quantify the likelihood of one event occurring in the presence of another, thereby offering a more nuanced approach to risk assessment and test prioritization.
For instance, consider a scenario where a component’s functionality is dependent on an external service. By calculating the conditional probability of the component failing when the external service is down, we can better assess the system’s robustness and direct our testing efforts more effectively. Similarly, in complex systems with a multitude of potential error states, conditional probability aids in estimating the chances of encountering specific errors under certain conditions, such as high load or particular data volumes.
Here are some practical applications of conditional probability in software testing:
- Risk assessment: Evaluating system risk by considering the probability of component failure due to external dependencies.
- Test case prioritization: Identifying and focusing on scenarios with a higher likelihood of error occurrence.
- Performance testing: Estimating the probability of performance issues under realistic usage conditions, such as high concurrency or large data sets.
By mastering conditional probability, testers can make informed decisions that enhance the effectiveness and efficiency of their test strategies.
Utilizing Bayes’ Theorem in Software Testing
Bayes’ theorem is a cornerstone in the realm of probability, offering a mathematical framework for updating the likelihood of a hypothesis as more evidence becomes available. In the context of software testing, it allows us to refine our understanding of the likelihood of defects as we accumulate test results. Bayes’ theorem transforms subjective intuition into quantitative analysis, providing a structured approach to decision-making.
When applying Bayes’ theorem to software testing, we follow a systematic process. First, we establish the prior probability of a defect based on historical data or expert judgment. As we execute test cases and observe outcomes, we update this probability to reflect the new evidence. This updated probability, known as the posterior probability, helps us make informed decisions about where to focus our testing efforts.
For instance, consider a scenario where a test case that often catches bugs passes. Using Bayes’ theorem, we can calculate the updated probability that a bug still exists despite the passing result. This is particularly useful when dealing with complex systems where interactions can be unpredictable. The table below illustrates how Bayes’ theorem can be applied to update our beliefs about the presence of a bug:
| Test Case Result | Prior Probability (P(B)) | Likelihood (P(A | B)) | Updated Probability (P(B | A)) |
|——————|————————–|———————–|——————————–|
| Pass | 10% | 30% | 3% |
| Fail | 10% | 70% | 7% |
In conclusion, Bayes’ theorem is not just a theoretical concept; it is a practical tool that enhances our ability to predict and manage software risks. By continuously updating our data with new bugs and test results, we can maintain a dynamic and effective testing strategy.
Predicting and Mitigating Software Risks with Probability
In the quest to build resilient software systems, probability serves as a guiding light, illuminating the path to predict and mitigate potential risks. By understanding the likelihood of various events, developers and testers can prioritize their efforts to focus on the most impactful issues.
One practical tool in this endeavor is the Risk Assessment Matrix. This matrix categorizes risks based on their probability of occurrence and the potential impact on the project. Here’s a simplified example:
Risk Event | Probability | Impact | Priority |
---|---|---|---|
Service Outage | High | Severe | Critical |
Data Corruption | Medium | High | High |
UI Inconsistency | Low | Moderate | Medium |
By systematically assessing risks, teams can allocate resources more effectively, ensuring that high-priority risks are addressed promptly. This strategic approach to risk management not only saves time but also safeguards the project’s bottom line from the adverse effects of unforeseen issues.
Testing Tools and Frameworks: Setting Up for Success
Navigating the Testing, Tools, and Frameworks Zone
In the realm of software development, the Testing, Tools, and Frameworks Zone is pivotal, marking one of the final checkpoints before deployment. This stage is not just about finding bugs; it’s about ensuring that the application performs as expected in the real world. To navigate this zone effectively, one must understand the variety of tools and frameworks available and how they align with the project’s needs.
Selecting the right tools is crucial. For instance, Tricentis Tosca has been highlighted as a top choice for those seeking a test automation platform that can expedite and enhance end-to-end testing with its AI-powered, codeless automation capabilities. It’s important to assess tools not just on their features but also on how they integrate with your existing processes and systems.
Ultimately, the goal is to leverage these tools to verify and validate the product, ensuring readiness for deployment. This involves a strategic approach where testing is not an afterthought but an integral part of the development lifecycle, continuously informing and improving the product.
Tailoring Tools and Frameworks to Development Needs
Selecting the right automation framework is crucial for the success of any software development project. Automation frameworks are essential tools for streamlining the testing process and ensuring the quality of software applications. They provide a structure that can be tailored to the specific needs of a development team, allowing for more efficient and effective testing.
When considering which tools and frameworks to adopt, it’s important to assess the compatibility with existing processes and the ability to integrate with other tools. For example, a project involving database changes might benefit from tools that support build automation, such as Maven or Gradle, and continuous integration tools like Jenkins or TravisCI.
The choice of tools should also align with the organization’s priorities. A thoughtful selection process that considers the long-term data alignment and integration across tools will facilitate a smoother workflow and support the organization’s overall goals. As the platform matures, the levels of automation and the capabilities of the tools can be expanded, leading to increased efficiency and productivity.
Leveraging Testing Practices for Verification and Validation
In the realm of software engineering, verification and validation are critical components that ensure a product not only meets the requirements but also fulfills its intended purpose effectively. Verification, often referred to as Static Testing, is the process of evaluating work-products of a development phase to determine whether they meet the specified requirements. Validation, on the other hand, is the dynamic testing of the actual product under various conditions to ensure it delivers the expected experience to the end-users.
To effectively leverage testing practices, it is essential to understand the distinction between these two approaches. Verification is concerned with the question, ‘Are we building the product right?’ whereas validation seeks to answer, ‘Are we building the right product?’ A comprehensive testing strategy will include both elements, with verification often taking place earlier in the software development life cycle (SDLC) and validation occurring closer to the deployment phase.
Here are some steps to ensure that both verification and validation are effectively leveraged:
- Establish clear and measurable requirements to serve as a benchmark for verification.
- Implement a variety of testing methods, such as unit tests, integration tests, and system tests, to cover different aspects of verification.
- Conduct thorough validation testing, including functional, non-functional, and user acceptance tests, to confirm the product’s fitness for use.
- Utilize feedback from validation testing to inform further development and refinement of the product.
Ensuring Readiness for Deployment with Effective Testing
As the final step in the SDLC, ensuring readiness for deployment is a critical phase that determines the success of an application in production. This stage is not just about confirming that the application ‘works’ but also about validating that it meets all the necessary requirements and can handle real-world use.
Effective testing at this stage involves a series of checks and balances. It’s essential to have a robust deployment checklist that includes both automated and manual testing processes. The checklist should cover various aspects such as functionality, performance, security, and usability. Here’s an example of what such a checklist might include:
- Functional correctness and completeness
- Performance benchmarks met
- Security vulnerabilities addressed
- User experience and interface validation
- Compatibility with different devices and browsers
- Data integrity and migration checks
It’s important to note that deployment is not a one-time event but a continuous process that often involves multiple environments (e.g., development, QA, staging). Each environment serves as a stepping stone, ensuring that by the time the application reaches production, it is thoroughly tested and ready for users. The deployment process, including the application and database migrations, should be automated as much as possible to minimize human error and ensure consistency across environments.
Advanced Debugging Strategies: Making the Most of Git Bisect
Automating Regression Testing with Git Bisect
Git bisect is a powerful tool for identifying regressions, which are steps backward in functionality where features that previously worked now fail. Automating regression testing with Git Bisect can significantly streamline the debugging process. By using Git Bisect, developers can perform a binary search within the repository, systematically narrowing down the search space until the problematic commit is found.
To effectively automate regression testing with Git Bisect, follow these steps:
- Start a bisect session with
git bisect start
. - Mark the known good and bad commits using
git bisect good [commit]
andgit bisect bad [commit]
. - Write a script that automatically tests each commit and integrates it with Git Bisect.
- Let Git Bisect run the script on each commit, automatically marking them as good or bad.
- Once the culprit commit is identified, end the bisect session with
git bisect reset
.
Remember, the goal is not just to find the commit where the regression was introduced but to do so in the most time-efficient manner possible. With these steps and the ability to handle flaky tests and skip untestable commits, Git Bisect becomes an indispensable part of your debugging toolkit.
Handling Flaky Tests During Debugging
Flaky tests are a common nuisance in software development, often causing confusion and delays in the debugging process. To crack the case on flaky tests, it’s essential to implement strategies that build confidence in your test suite. One effective approach is to rerun the flaky test multiple times to determine its reliability. For instance, a simple bash script can execute a flaky test three times, considering it a pass if it succeeds in at least two out of three runs. This method helps in differentiating between a true regression and an intermittent failure.
When dealing with flaky tests during git bisect, it’s crucial to incorporate logic into your automation scripts that can handle these uncertainties. Here’s a concise example of how to manage flaky tests within your script:
- Run the test multiple times
- Define success criteria (e.g., two out of three passes)
- Use
git bisect skip
for commits that can’t be tested
By applying these advanced strategies, you enhance the utility of git bisect in your debugging toolkit, ensuring more efficient and accurate results. Remember, the goal is to uncover causes, ask the right questions, and implement strategies that lead to reliable builds and seamless upgrades.
Skipping Untestable Commits Efficiently
In the process of using git bisect
to identify problematic commits, developers may encounter scenarios where certain commits are untestable. This could be due to broken builds, incomplete features, or other issues that prevent the commit from being tested reliably. Using git bisect skip
is crucial in these situations, as it allows developers to bypass these problematic commits without affecting the overall bisect process.
When you come across a commit that needs to be skipped, simply issue the command git bisect skip
. This tells Git to exclude the current commit from the search and continue with the next one. However, it’s important to exercise caution with this command:
- Use
git bisect skip
sparingly to avoid skewing the results. - Only skip commits when it’s absolutely necessary.
- Remember that skipping too many commits can compromise the accuracy of the bisect.
By following these guidelines, you can ensure that your use of git bisect
remains effective and that you’re able to pinpoint the source of a regression in the most time-efficient manner possible.
Time-Efficient Debugging with Git Bisect
Git bisect is a powerful ally in the quest for efficient debugging, especially when dealing with regressions. By systematically halving the search space, git bisect swiftly pinpoints the problematic commit, transforming a potentially lengthy and error-prone process into a manageable task.
To optimize the use of git bisect, consider these advanced strategies:
- Script automation to enhance precision and reduce manual effort.
- Intelligent handling of flaky tests to maintain the integrity of the bisecting process.
- Knowing when to skip untestable commits to avoid unnecessary delays.
These strategies not only save time but also improve the accuracy of your debugging efforts. With git bisect, you’re equipped to uncover even the most elusive regressions, ensuring that your codebase remains robust and reliable.
Iterative Approaches in Test Automation
Improving Test Script Quality Through Iteration
The journey to enhance your QA process begins with recognizing that the first draft of a test script is rarely its final form. Iteration is key to refining and perfecting test scripts. By adopting an iterative approach, teams can incrementally improve script quality, leading to more reliable and maintainable tests.
For instance, consider the iterative prompts that guide the development of a login functionality test script. Starting with a basic script, subsequent iterations can expand to cover a comprehensive range of scenarios, such as valid and invalid credentials, as well as empty fields. This process ensures that scripts are up-to-date with the latest UI elements and include thorough assert statements.
Incorporating logic to handle flaky tests is another aspect of script refinement. A robust automation testing strategy might involve rerunning tests under certain conditions or applying more sophisticated checks to distinguish between true regressions and intermittent failures. Here’s a simplified example of how to approach this:
- Identify known flaky tests.
- Adjust the script to run these tests multiple times.
- Consider a test as failing only if it consistently fails across runs.
Refining Test Automation Practices for Better Outcomes
In the pursuit of refining test automation practices, it’s essential to focus on the iterative improvement of test scripts. The initial results may not always align with expectations, necessitating a cycle of refinement and reevaluation. This process is not only about correcting errors but also about enhancing the effectiveness and efficiency of the test automation suite.
A key aspect of this refinement is the utilization of detailed information in prompts. Providing clear goals, context, and expectations can lead to more comprehensive and relevant test scripts. For instance, specifying sources of information like log files or test reports can guide the automation tool to produce better outcomes.
To systematically improve test automation practices, consider the following metrics as part of your reporting strategy:
- Test coverage percentage
- Pass/fail rate of test cases
- Average time to run tests
- Number of defects found by automated tests
- Test script reliability (flakiness)
- Return on investment (ROI) for test automation
By comparing different prompts and their effectiveness, Test Automation Engineers can identify which approaches yield the most valuable results. Structuring prompts strategically and observing the differences in generated test cases can highlight the best practices for your specific environment.
Incorporating Statistical and Machine Learning Testing Techniques
The integration of statistical methods and machine learning into test automation is transforming the landscape of software quality assurance. Statistical testing allows us to analyze hypothesis testing results and p-values, ensuring that our assumptions and data sets align with the specific conditions of our software system.
Machine learning, on the other hand, enhances the precision of test automation by evaluating the conditional probability of model predictions. This is particularly important when determining the likelihood of errors under specific input conditions. Remember, selecting the appropriate "given" conditions is essential for obtaining meaningful results.
The synergy of these techniques provides a robust framework for optimizing testing efforts. By understanding the dependencies between events, we can prioritize test cases that are more likely to trigger critical errors, thereby improving the efficiency and reliability of our testing processes.
Understanding Conditional Probability in Test Automation
In the realm of test automation, conditional probability is a pivotal concept that enhances our understanding of how certain conditions affect the likelihood of events during testing. It is particularly useful when we aim to predict the probability of defects or errors under specific circumstances.
Conditional probability, denoted as P(A|B), is the probability of an event A occurring given that event B has already taken place. For instance, in test automation, we might be interested in the probability of a test failing (event A) after a particular code change (event B). This approach allows us to tailor our testing efforts more effectively, focusing on areas with higher risks.
To illustrate the application of conditional probability in test automation, consider the following table which outlines the likelihood of encountering certain errors given different user inputs:
User Input | Error Type | Conditional Probability |
---|---|---|
Input A | Error X | 0.4 |
Input B | Error Y | 0.25 |
Input C | Error Z | 0.1 |
By integrating conditional probability into our testing strategies, we can prioritize test cases and optimize our resources. This ensures that we focus on the most critical aspects of the system, thereby improving the overall quality and reliability of the software.
Conclusion
Throughout this article, we have explored the intricate tapestry of software testing, delving into various strategies and methods that serve as the bulwark against the unpredictable tides of software bugs. From the foundational principles of unit testing with JUnit and Assert frameworks to the advanced applications of probability in predicting and mitigating risks, we have journeyed through the realms of conditional probabilities and Bayes’ theorem, and examined the pivotal role of testing tools and frameworks in the software development lifecycle (SDLC). The iterative process of refining test approaches underscores the continuous pursuit of optimization in our quest to build robust and reliable software. As we conclude, remember that the essence of software testing lies not only in the tools and theories but in their judicious application to enhance the quality and dependability of our digital creations.
Frequently Asked Questions
What are some best practices for writing unit tests with JUnit and Assert?
Best practices include writing clean, readable tests that clearly state their purpose, using descriptive test names, and structuring tests for easy maintenance. Utilize Assert’s various methods to make assertions more expressive and ensure tests are focused and test only one aspect at a time.
How can false positives be avoided in unit testing?
To avoid false positives, ensure that your tests are accurately testing the right conditions, and that test data is properly isolated. Mock dependencies to test components in isolation and use assertions that match the intended outcomes precisely.
What are common mistakes to avoid in unit testing?
Common mistakes include writing tests without clear objectives, overusing mocks, neglecting to test edge cases, and not keeping tests updated as the code evolves. Avoid excessive NPE checks that can mask real issues and ensure tests remain relevant and effective.
How does probability influence software testing strategies?
Probability helps in understanding the likelihood of various events, such as defects or usage patterns, which informs test coverage and risk management. It guides the prioritization of test scenarios based on their impact and likelihood to ensure a more effective testing process.
What role does conditional probability play in test automation?
Conditional probability is used to assess the likelihood of certain outcomes given specific preconditions in test automation. It helps in refining test cases to be more targeted and in understanding dependencies between different parts of the software system.
How can Git Bisect be used to enhance debugging in software development?
Git Bisect automates the process of finding the specific commit that introduced a regression by systematically testing commits between a known good and bad state. It streamlines the debugging process, making it more efficient by identifying the source of issues quickly.