A Guide to Crafting a Unit Testing Sample
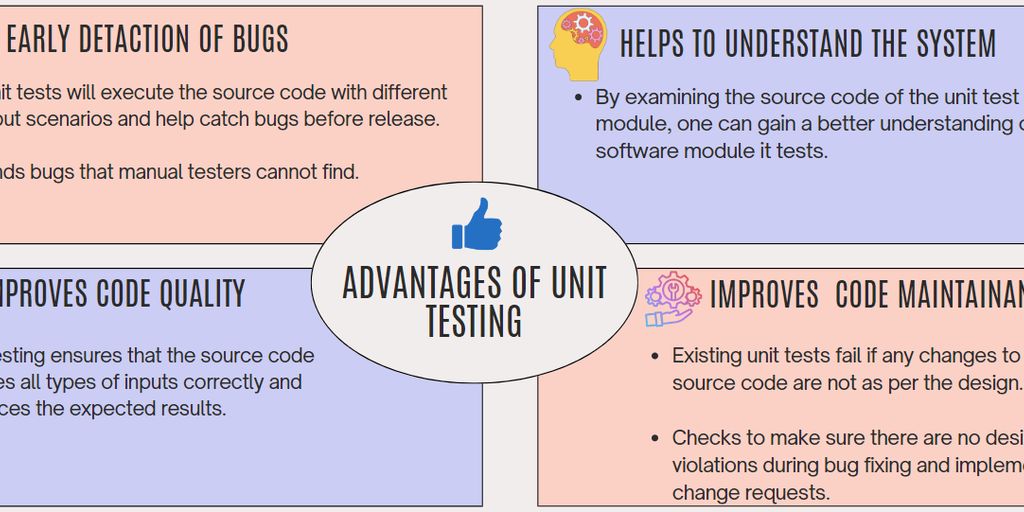
Unit testing is a key part of making sure software works well. It helps find bugs early and makes code easier to manage. This guide will teach you how to write good unit tests, so your code stays reliable and your team works better together.
Key Takeaways
- Unit testing helps catch bugs early and makes code more reliable.
- Good unit tests make it easier to change and improve code over time.
- Using the right tools and setup is important for effective unit testing.
- Writing clear and independent tests helps avoid problems later.
- Unit testing supports teamwork and continuous improvement in software projects.
Understanding the Importance of Unit Testing
Benefits of Unit Testing
Unit testing is crucial to ensure software quality. It helps make sure that the software application meets its functional requirements and works as intended. Unit tests play a crucial role in ensuring that individual components of code, known as units, perform as intended and integrate seamlessly within the larger application. These tests serve as a shield against bugs, enable smooth code refactoring, provide living documentation, and act as a safety net for preventing regressions.
Common Misconceptions About Unit Testing
Many believe that unit testing is time-consuming and unnecessary if the code works. However, this is a misconception. Unit tests can save time in the long run by catching bugs early and making the code easier to maintain. Another common myth is that unit tests are only for large projects. In reality, even small projects can benefit from unit testing by ensuring each part works correctly.
Unit Testing vs. Other Testing Methods
Unit testing focuses on individual components, while other methods like integration testing and system testing look at the bigger picture. Integration testing checks how different parts of the application work together, and system testing evaluates the entire system’s functionality. Each type of testing has its place, but unit testing is the foundation that ensures each piece of the puzzle works as it should.
Setting Up Your Unit Testing Environment
Setting up your unit testing environment is a crucial step in ensuring your tests run smoothly and effectively. In this guide, I will walk you through practical steps to set up your test environment, ensuring a smooth and effective process.
Choosing the Right Tools
Selecting the right tools for unit testing can make a significant difference. Choose tools that integrate well with your development environment and support the programming languages you use. Popular options include Jest for JavaScript, JUnit for Java, and NUnit for .NET.
Configuring Your Development Environment
Configuring your development environment properly is essential. Create a new test file or function specifically for the unit you are testing. Import the necessary testing framework or libraries. Ensure that the unit tests execute the same on all environments by correctly stubbing or mocking any dependencies and setting any state or external data.
Integrating Unit Testing into Your Workflow
Integrating unit testing into your workflow helps maintain code quality. Use an interactive wizard to customize settings according to your preferences. Make sure that there is no dependency on other tests. Each test should run in isolation and should not need the result of a previous test in order to execute correctly. This ensures that your standard development workflow remains unaffected.
Writing Your First Unit Test
Identifying Test Cases
Before you start writing your first unit test, it’s crucial to identify what you need to test. Look for key functionalities in your code that are critical to its operation. Focus on the core logic and edge cases. This will ensure that your tests cover the most important parts of your application.
Structuring Your Test Code
Organizing your test code is just as important as writing the tests themselves. Follow a consistent structure to make your tests easy to read and maintain. Typically, a unit test should include the following sections:
- Setup: Prepare the environment and inputs for the test.
- Execution: Run the code being tested.
- Verification: Check that the results are as expected.
- Teardown: Clean up any resources used during the test.
Running and Evaluating Tests
Once your tests are written, the next step is to run them and evaluate the results. Use a testing framework to automate this process. Pay attention to any test failures and investigate the causes. A failed test is an opportunity to improve your code. Make sure to fix any issues and re-run the tests to ensure everything is working correctly.
Advanced Unit Testing Techniques
Mocking Dependencies
Mocking is a technique used to simulate the behavior of complex objects or external systems. This is especially useful when you need to test a unit in isolation. By using mocks, you can focus on the unit under test without worrying about the dependencies. This makes your tests more reliable and easier to maintain.
Testing Edge Cases
Edge cases are scenarios that occur at the extreme ends of operating conditions. Testing these cases ensures that your software can handle unexpected inputs or situations. For example, you might test how your application behaves with very large or very small numbers, or with empty inputs. This helps in identifying potential bugs that might not be obvious during regular testing.
Performance Testing in Unit Tests
Performance testing within unit tests helps you measure how efficiently your code runs. This can be done by timing how long certain operations take and ensuring they meet performance criteria. While unit tests are primarily for functionality, adding performance checks can help you catch inefficiencies early in the development process.
Best Practices for Effective Unit Testing
Maintaining Test Readability
Clear and readable tests are essential. Use descriptive test names to make it obvious what each test is checking. Avoid using magic strings and keep your tests simple. This helps others understand and maintain the tests easily.
Ensuring Test Independence
Each test should be able to run on its own without relying on other tests. This means avoiding shared states between tests. Use helper methods instead of setup and teardown functions to keep tests isolated.
Continuous Integration and Unit Testing
Integrate your unit tests into a continuous integration (CI) system. This ensures that tests run automatically whenever code changes are made, catching issues early. CI systems support code refactoring and continuous improvement by providing quick feedback on code changes.
By following these best practices, developers can write effective unit tests that provide reliable feedback, promote code maintainability, and contribute to the overall quality and stability of the software. Effective unit tests are an investment in the long-term success of the application, enabling developers to confidently make changes and deliver a robust and dependable product.
Common Pitfalls and How to Avoid Them
Over-Mocking
Over-mocking can make your tests fragile and hard to maintain. Relying too much on mocks can lead to tests that don’t reflect real-world scenarios. Instead, use mocks sparingly and only when necessary. Aim to test the actual behavior of your code as much as possible.
Ignoring Test Failures
Ignoring test failures is a dangerous habit. When a test fails, it indicates a potential issue in your code. Always investigate and resolve test failures promptly to maintain code quality. Ignoring them can lead to bigger problems down the line.
Writing Tests for Non-Testable Code
Writing tests for code that is difficult to test can be a sign of poor design. If you find it hard to write tests for your code, consider refactoring it to make it more testable. Good design and testability often go hand in hand.
Leveraging Unit Testing for Continuous Improvement
Refactoring with Confidence
Unit testing allows developers to refactor code with confidence. By having a suite of tests that verify the functionality of the code, developers can make changes knowing that any issues will be quickly identified. This process not only improves the code quality but also ensures that the software remains reliable over time.
Collaborative Testing Practices
Encouraging a culture of collaborative testing within the team can lead to better outcomes. When developers, testers, and other stakeholders work together, they can share insights and identify potential issues early. This collaboration fosters a sense of shared responsibility for the quality of the code.
Automating Test Execution
Automating the execution of unit tests is crucial for maintaining a high level of code quality. By integrating automated tests into the continuous integration (CI) and continuous delivery (CD) pipelines, teams can ensure that tests are run consistently and efficiently. This automation helps in catching bugs early and reduces the manual effort required for testing.
Benefits of Automating Test Execution:
- Enhances code reliability and reduces bugs.
- Facilitates early detection of issues in the development process.
- Simplifies debugging by pinpointing specific failures.
- Supports code refactoring and continuous integration.
- Improves documentation and serves as executable specifications.
Conclusion
Unit testing is a key part of making sure your code works well and is easy to maintain. By following the steps and tips in this guide, you can write tests that help catch bugs early and make your code more reliable. Good unit tests also make it easier for your team to work together and keep improving the code. Remember, investing time in writing solid unit tests now will save you a lot of trouble in the future. Happy testing!
Frequently Asked Questions
What is unit testing?
Unit testing is when you check small parts of your code to make sure they work correctly. This helps catch bugs early and makes your code more reliable.
Why is unit testing important?
Unit testing is important because it helps you find and fix bugs early. It also makes your code easier to understand and maintain.
How do I start writing a unit test?
To start writing a unit test, first pick a small part of your code to test. Then, write a test case that checks if that part of the code works as expected.
What tools do I need for unit testing?
There are many tools for unit testing, like JUnit for Java or pytest for Python. Choose one that works best with your programming language.
What are common mistakes in unit testing?
Common mistakes include writing tests that are too complex, not running tests often, and ignoring test failures. It’s important to keep tests simple and run them regularly.
How can unit testing improve teamwork?
Unit testing improves teamwork by making the code more reliable and easier to understand. This helps team members work together more effectively and catch issues early.