How to Create Effective Unit Testing Test Cases
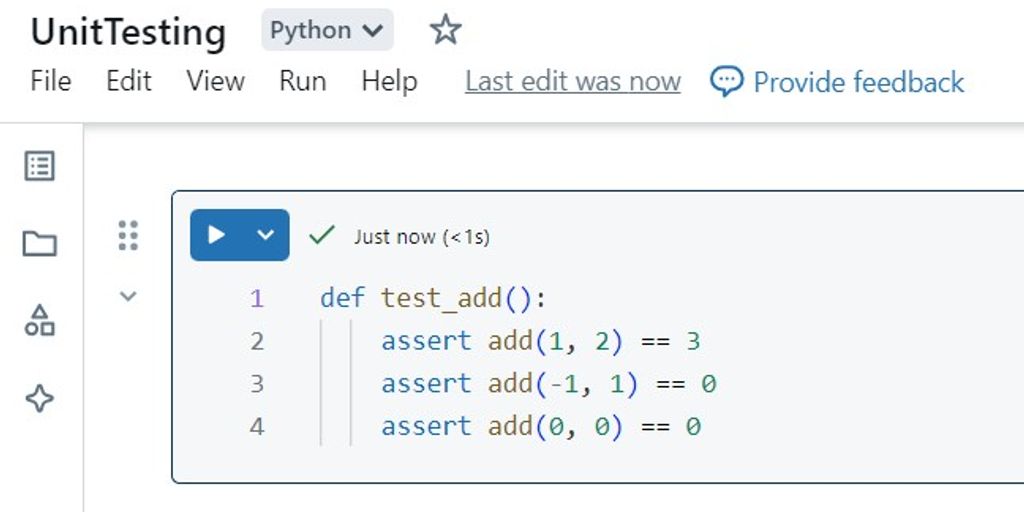
Creating effective unit testing test cases is crucial for ensuring that your code works correctly and is easy to maintain. Simple and readable tests help you understand why something fails and make it easier to fix. This article will guide you through the best practices for writing unit tests that are trustworthy and easy to manage.
Key Takeaways
- Write simple and readable tests to easily identify and fix issues.
- Decouple your code to make it easier to test and maintain.
- Use automated tests to save time and ensure repeatability.
- Follow the Arrange, Act, Assert pattern for clear test structure.
- Cover different scenarios, including edge cases, to ensure robustness.
Writing Readable and Simple Unit Tests
Creating unit tests that are easy to read and simple is crucial for effective testing. Readable tests help other developers understand how the code works and what went wrong if a test fails. This makes it easier to troubleshoot without extensive debugging.
Importance of Simplicity
Simple tests are easier to write, maintain, and understand. When tests are straightforward, they tend to have fewer bugs. If issues do arise, they are much easier to fix. Additionally, simple tests are easier to refactor. If the test is complex and you need to refactor some code, the tests might break.
Decoupling Code Through Testing
Writing tests for your code will naturally decouple your code, because it would be more difficult to test otherwise. Decoupled code is more modular and easier to manage. This separation makes it easier to identify and fix issues in specific parts of the code.
Refactoring with Confidence
When you have simple and readable tests, you can refactor your code with confidence. You know that if something breaks, your tests will catch it. This allows you to improve and optimize your code without fear of introducing new bugs.
Characteristics of Effective Unit Testing Test Cases
Automation and Repeatability
Effective unit tests should be automated and repeatable. Automation ensures that tests can be run with minimal human intervention, making it easier to catch bugs early. Repeatability means that the same tests can be run multiple times with the same results, ensuring consistency.
Ease of Implementation
Unit tests should be easy to write and implement. This encourages developers to write tests more frequently, leading to better code quality. Simple tests are also easier to understand and maintain.
Trustworthiness and Maintainability
For unit tests to be effective, they must be trustworthy. This means they should reliably indicate whether the code is working as expected. Maintainability is also crucial; tests should be easy to update as the code evolves. This ensures that the tests remain useful over time.
Best Practices for Creating Unit Testing Test Cases
Creating effective unit testing test cases is crucial for ensuring your code works as intended. Here are some best practices to follow:
Arrange, Act, Assert Pattern
The Arrange, Act, Assert (AAA) pattern is a simple and clear way to structure your tests. First, set up your test data and environment (Arrange). Next, execute the code being tested (Act). Finally, verify the results (Assert). This pattern helps keep your tests organized and easy to understand.
BDD-Style Test Cases
Behavior-Driven Development (BDD) encourages writing tests in a way that describes the behavior of the application. This makes tests more readable and closer to natural language. For example, you might write, "Given a user logs in, when they enter correct credentials, then they should see the dashboard." This style helps non-developers understand what the tests are doing.
Avoiding Magic Numbers
Magic numbers are hard-coded values that have no clear meaning. Instead of using magic numbers, use constants or variables with descriptive names. This makes your tests easier to read and maintain. For example, instead of writing assertEqual(5, result)
, you could write assertEqual(MAX_USERS, result)
, where MAX_USERS
is a constant defined elsewhere in your code.
Ensuring Deterministic Unit Tests
Importance of Determinism
For a test to be reliable, it must be deterministic. This means that if the code hasn’t changed, the test should always produce the same result. If a test sometimes passes and sometimes fails without any code changes, it becomes unreliable. Developers will lose trust in such tests, making them less useful.
Handling Non-Deterministic Algorithms
Non-deterministic algorithms can be tricky to test. To manage this, you should isolate your tests. Ensure they don’t depend on external factors like system time, environment variables, or other test cases. Use setup and teardown methods provided by your testing framework to control the environment before and after each test.
Custom Assertion Functions
Creating custom assertion functions can help in making your tests more deterministic. These functions allow you to define specific conditions that must be met for the test to pass. This way, you can handle edge cases and ensure that your tests are both reliable and meaningful.
Covering Different Testing Scenarios
Happy Path Testing
When you start testing new features, it’s smart to begin with the happy path. This means you test the most common and expected use of the feature. By doing this, you ensure that the main functionality works as intended. Once the happy path is confirmed, you can move on to more complex scenarios.
Testing Edge Cases
Edge cases are the unusual or extreme conditions that might break your code. These are important to test because they help you find bugs that wouldn’t show up in normal use. Examples include very large inputs, empty inputs, or unexpected data types. Testing these cases makes your code more robust.
Combining Unit and Integration Testing
While unit tests focus on individual parts of your code, integration tests check how these parts work together. Combining both types of tests gives you a more complete picture of your software’s health. Unit tests catch small issues early, while integration tests ensure that everything works well as a whole.
Automating and Scaling Unit Tests
Automated Test Execution
Automating unit tests is crucial for maintaining software quality. Setting up an automated process for unit testing ensures that tests run regularly, whether daily, hourly, or through a CI/CD pipeline. This helps teams quickly identify and fix issues, improving overall efficiency. Automated tests should be easy to run, repeatable, and provide clear reports that all team members can access and discuss.
Scalability of Test Cases
As your codebase grows, so should your unit tests. It’s important to write tests that are isolated and cover specific scenarios. This makes it easier to identify issues when tests fail. Using tools like AI-driven test generators can help create a wide range of test cases, covering various inputs and edge cases. This approach ensures that your tests remain effective and manageable as your project scales.
Continuous Integration
Integrating unit tests into your CI pipeline is essential for continuous delivery. This practice ensures that every code change is tested automatically, reducing the risk of introducing bugs. A well-configured CI system can run tests quickly and provide immediate feedback, allowing developers to address issues promptly. This continuous testing cycle helps maintain high code quality and accelerates the development process.
Conclusion
Creating effective unit tests is key to making sure your code works well and is easy to maintain. By writing simple and readable tests, you can quickly find and fix problems. Remember to test small parts of your code one at a time and keep your tests short and clear. Use patterns like Arrange, Act, Assert to organize your tests. Also, make sure your tests can run automatically and give the same results every time. Following these tips will help you write better tests and build stronger software.
Frequently Asked Questions
What is the Arrange, Act, Assert pattern in unit testing?
The Arrange, Act, Assert pattern is a way to structure your tests. First, you set up your test data (Arrange). Then, you run the code you’re testing (Act). Finally, you check the results (Assert). This pattern helps make tests clear and easy to understand.
Why should unit tests be simple and readable?
Simple and readable tests are easier to write and maintain. They help you understand why a test fails and make it easier to fix the problem. Complex tests can break when you change your code, making them hard to manage.
What makes a unit test trustworthy and maintainable?
A trustworthy unit test gives reliable results every time you run it. To make tests maintainable, write them in a way that is easy to update when your code changes. This includes using clear names and avoiding hard-to-understand logic.
Why is it important for unit tests to be automated and repeatable?
Automated and repeatable tests save time and effort. You can run them with a push of a button, and they give the same results every time. This makes it easier to catch bugs early and ensures your code works as expected.
What are magic numbers and why should they be avoided in unit tests?
Magic numbers are hard-coded values with no explanation. They make tests hard to understand and maintain. Instead, use variables or constants with meaningful names to make your tests clearer.
How can you handle non-deterministic algorithms in unit tests?
Non-deterministic algorithms can give different results each time you run them. To handle this in tests, you can use mock data or set fixed conditions. This makes sure your tests give consistent results.