Comprehensive Guide to Tools for Unit Testing
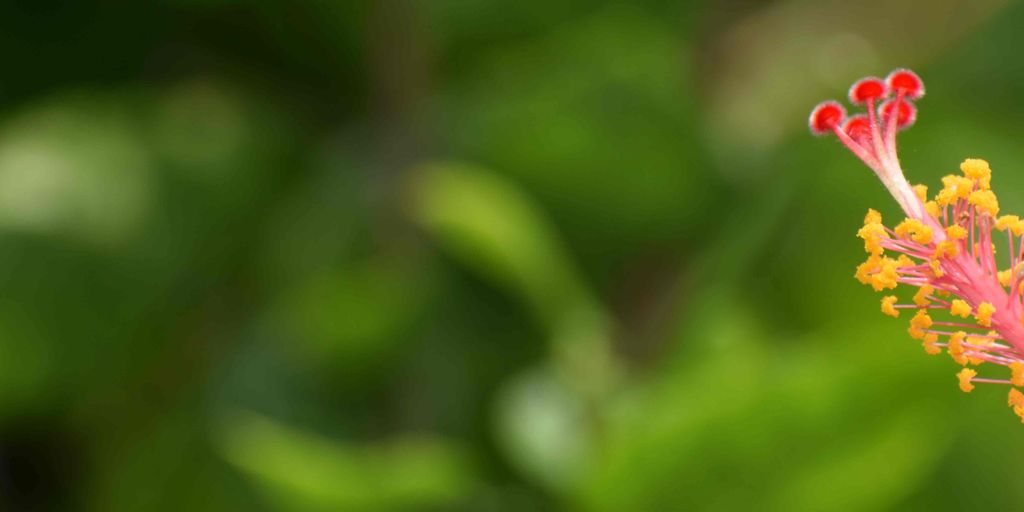
Unit testing is a fundamental aspect of software development that focuses on verifying the functionality of individual components or units of code. By ensuring that each part of the codebase works as expected, unit testing helps developers catch bugs early, facilitates refactoring, and increases confidence in the overall quality of the software. This comprehensive guide will explore the top tools for unit testing, key features to consider, best practices, advanced techniques, and real-world case studies to help you master the art of unit testing.
Key Takeaways
- Unit testing is essential for verifying the functionality of individual code components.
- Popular unit testing tools include JUnit, NUnit, and xUnit, each offering unique features and benefits.
- Key features to look for in unit testing tools include ease of use, integration capabilities, and automation support.
- Best practices such as writing effective test cases and maintaining test code quality are crucial for successful unit testing.
- Advanced techniques like mocking, stubbing, and continuous integration can further enhance your unit testing efforts.
Top Tools for Unit Testing
When it comes to ensuring the quality and reliability of your code, selecting the right unit testing tool is crucial. These tools use various AI techniques to automate and optimize different aspects of code review, test generation, and quality assurance. Below, we explore some of the top tools for unit testing that can help streamline your development process and improve your software quality.
JUnit
JUnit is an open-source unit testing tool in Java. It does not require the creation of class objects or the definition of the main method to run tests. It has an assertion library for evaluating test results. Annotations in JUnit are used to execute test methods. JUnit is commonly used to run automation suites with multiple test cases.
NUnit
NUnit is a popular unit testing framework for .NET languages. It supports data-driven tests, allowing you to run a set of tests with different inputs. NUnit also integrates well with various CI/CD pipelines, making it a versatile choice for automated testing.
xUnit
xUnit is another open-source unit testing tool that is widely used for .NET applications. It offers a rich set of features, including support for parallel test execution and extensive assertion libraries. xUnit is designed to be extensible, allowing developers to create custom test behaviors and assertions.
Features to Look for in Unit Testing Tools
When selecting a unit testing tool, it’s essential to consider several key features to ensure it meets your project’s needs effectively. Ease of use is paramount, as a tool that is difficult to navigate can hinder productivity and lead to frustration among team members. Look for tools that offer intuitive interfaces and comprehensive documentation.
Ease of Use
A user-friendly unit testing tool can significantly streamline the testing process. Tools that are easy to set up and configure allow developers to focus on writing tests rather than wrestling with the tool itself. Additionally, tools that provide clear and concise error messages can help in quickly identifying and resolving issues.
Integration Capabilities
Integration capabilities are crucial for a seamless development workflow. A good unit testing tool should integrate well with your existing development environment, including your IDE, build tools, and version control systems. This ensures that testing becomes a natural part of the development process rather than an afterthought.
Automation Support
Automation support is another critical feature to look for. Automated unit testing can save a significant amount of time and effort by running tests automatically as part of your continuous integration pipeline. This not only helps in maintaining code quality but also in catching issues early in the development cycle. Look for tools that offer robust automation features and can easily integrate with your CI/CD pipeline.
How to Choose the Right Unit Testing Tool
Project Requirements
When selecting a unit testing tool, it’s crucial to consider the specific needs of your project. Different tools offer various features, and some may be better suited for certain types of projects than others. Ensure the tool you choose aligns with your project’s technology stack and testing requirements.
Team Expertise
The proficiency of your team with a particular tool can significantly impact the effectiveness of your unit testing efforts. If your team is already familiar with a specific tool, it might be beneficial to continue using it to avoid a steep learning curve. Conversely, if your team is open to learning, you might consider a tool that offers more advanced features.
Budget Constraints
Budget is always a consideration when choosing any tool. While some unit testing tools are open-source and free to use, others come with licensing fees. Evaluate the cost against the features and benefits provided to determine if the investment is worthwhile. Here’s a quick comparison of some popular tools:
Tool | Cost | Key Features |
---|---|---|
JUnit | Free | Open-source, widely used, robust |
NUnit | Free | Open-source, .NET support |
xUnit | Free | Open-source, extensible |
Jtest | Paid | IDE plugin, automation capabilities |
Best Practices for Unit Testing
Unit testing is a critical aspect of software development that ensures individual components of the code work as expected. Here are some best practices to follow:
Writing Effective Test Cases
When writing a unit test, it’s essential to follow some best practices. These include:
- Keeping your tests focused and simple.
- Choosing relevant inputs for your tests.
- Paying attention to edge cases and other potential issues that could impact the quality of your test results.
- Ensuring that your tests are repeatable and reliable over time, regardless of any changes you make to your application or system under test.
Maintaining Test Code Quality
- Unit tests should be simple: Each unit test should focus on verifying a specific behavior or functionality (follow the “one assertion per test” rule). Structure your test on the AAA pattern to maintain clarity and readability in your unit tests. Choose descriptive, meaningful, but simple names for your test methods so that you have an easier time managing thousands of them.
- Unit tests should be executed in isolation: Code isolation is a highly recommended practice to eliminate any external dependencies that could affect the test results.
Integrating Testing into Development Workflow
Integrating unit testing into your development workflow is crucial for maintaining code quality and catching issues early. This can be achieved by:
- Automating tests to run with every build.
- Using continuous integration tools to ensure tests are executed regularly.
- Encouraging a test-driven development (TDD) approach where tests are written before the code itself.
Advanced Unit Testing Techniques
Mocking and Stubbing
Mocking and stubbing are essential techniques in unit testing. They allow you to simulate the behavior of complex, real objects, making it easier to test individual units of code in isolation. Mocking involves creating objects that mimic the behavior of real objects, while stubbing provides predefined responses to method calls.
Code Coverage Analysis
Code coverage analysis helps you measure the extent to which your codebase is tested. It provides insights into which parts of your code are covered by tests and which are not. This technique is crucial for identifying untested parts of your application and ensuring thorough test coverage.
Continuous Integration
Continuous integration (CI) is a practice where code changes are automatically tested and integrated into the main codebase. This technique helps catch bugs early and ensures that new code does not break existing functionality. CI tools can automate the execution of unit tests, making the process more efficient and reliable.
Common Challenges in Unit Testing and How to Overcome Them
Unit testing is a crucial part of the software development process, but it comes with its own set of challenges. Understanding these challenges and knowing how to overcome them can significantly improve the effectiveness of your unit tests.
Case Studies: Successful Unit Testing Implementations
Case Study 1: E-commerce Platform
In this case study, we explore how an e-commerce platform successfully implemented unit testing to enhance their software quality. By breaking down applications into smaller units and testing each unit independently, the team was able to catch bugs early and facilitate refactoring. The challenges faced included dealing with legacy code and managing test data, but these were overcome through strategic planning and the use of advanced unit testing techniques.
Case Study 2: Financial Application
This case study delves into the unit testing strategies employed by a financial application. The team focused on ensuring thorough test coverage by using various unit testing techniques. They also integrated testing into their development workflow, which helped in maintaining test code quality. The key takeaway from this case study is the importance of handling dependencies effectively to ensure reliable test results.
Case Study 3: Mobile App
In the final case study, we examine a mobile app’s journey in implementing unit testing. The team faced unique challenges, such as testing microservices and ensuring compatibility across different devices. However, by leveraging automation support and integration capabilities, they were able to achieve a robust unit testing framework. This case study highlights the significance of continuous integration in maintaining code quality and reliability.
Conclusion
Unit testing is an essential practice for ensuring the reliability and quality of your codebase. By leveraging the right tools, such as JUnit, JTest, NUnit, and xUnit, you can automate and streamline your testing process, making it more efficient and effective. This guide has provided a comprehensive overview of the best tools available, along with actionable insights and recommendations to help you master unit testing. Whether you are a beginner or looking to enhance your existing practices, adopting the techniques and tools discussed in this article will enable you to catch bugs early, facilitate refactoring, and increase your confidence in your code. Embrace these best practices to improve your development workflow and deliver robust, high-quality software.
Frequently Asked Questions
What are the best tools for unit testing?
The best tools for unit testing include JUnit, NUnit, xUnit, and Parasoft Jtest. These tools offer various features such as ease of use, integration capabilities, and automation support.
How do I perform unit testing?
Unit testing involves breaking down application code into smaller components or units and testing each unit individually to ensure it works as expected. Tools like JUnit and NUnit can help automate this process.
What features should I look for in a unit testing tool?
When choosing a unit testing tool, look for features such as ease of use, integration capabilities, automation support, and the ability to handle dependencies and manage test data.
How do I choose the right unit testing tool for my project?
To choose the right unit testing tool, consider your project requirements, team expertise, and budget constraints. Evaluate different tools to see which one aligns best with your needs.
What are some best practices for unit testing?
Best practices for unit testing include writing effective test cases, maintaining test code quality, and integrating testing into the development workflow. Additionally, using techniques like mocking and stubbing can improve test accuracy.
What are common challenges in unit testing and how can I overcome them?
Common challenges in unit testing include dealing with legacy code, handling dependencies, and managing test data. Overcoming these challenges involves using advanced techniques like mocking, stubbing, and continuous integration, as well as maintaining good test code practices.