Step-by-Step Unit Test Example for Your Code
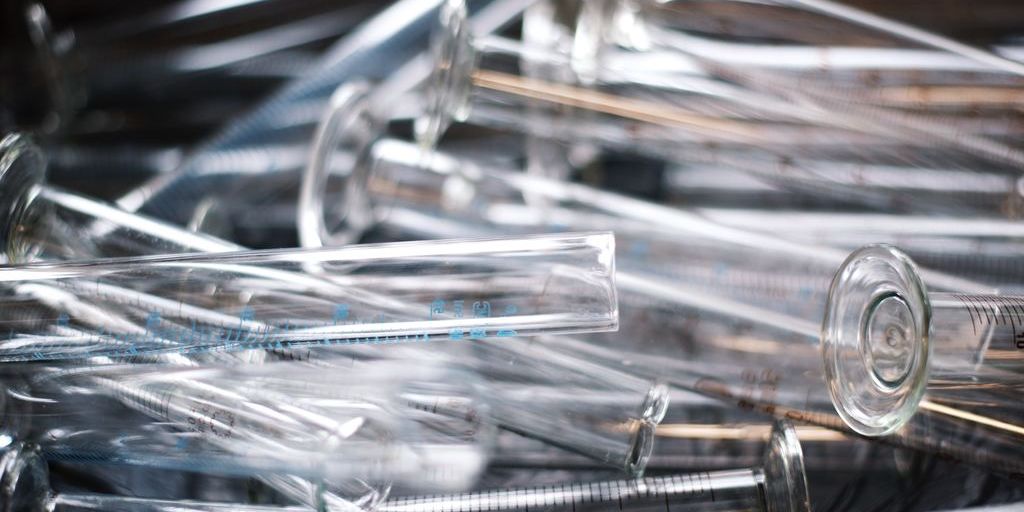
Unit testing helps developers ensure that each part of their code works correctly. By focusing on small, individual units, you can catch bugs early and make your code more reliable. This guide will walk you through the steps of creating unit tests, from picking the right parts to test, to writing and running your tests effectively.
Key Takeaways
- Unit testing focuses on checking small, specific parts of your code.
- Effective test cases should include a variety of inputs, including edge cases.
- Assertions are used to compare the actual output of your code to the expected result.
- Tools like Pytest make it easier to write and run unit tests in Python.
- Regularly updating and refactoring your tests helps keep your codebase healthy.
Choosing the Right Unit to Test
Understanding Unit Boundaries
To grasp unit testing, you first need to define what a "unit" is. A unit is the smallest part of your code that you can test. It could be a single function, a method, or even a class. Understanding these boundaries helps you focus your tests and make them more effective.
Selecting Critical Functions
Not all parts of your code are equally important. Focus on testing the critical functions that are essential for your application. These are the parts that, if they fail, could cause the most damage. Prioritizing these functions ensures that your tests are both efficient and effective.
Avoiding Overlapping Tests
When writing tests, it’s important to avoid overlap. Overlapping tests can waste time and resources. Make sure each test is unique and covers a specific part of your code. This way, you can ensure that your tests are comprehensive without being redundant.
Writing Effective Test Cases
Defining Test Inputs
When creating test cases, the first step is to define the inputs. These inputs should cover a wide range of scenarios, including typical cases, edge cases, and invalid inputs. By testing various inputs, you ensure your code handles all possible situations.
Creating Test Functions
Test functions should be simple and focused. Each function should test a single aspect of the code. This makes it easier to identify what is broken if a test fails. Use clear and logical names for your test functions to make them easy to understand.
Handling Edge Cases
Edge cases are unusual situations that might not occur often but can cause your code to fail. It’s important to identify and test these cases to ensure your code is robust. Examples of edge cases include empty inputs, very large numbers, and unexpected data types.
Using Assertions for Validation
Assertions are the backbone of unit testing. They help you check if your code works as expected. When you write a test, you use assertions to compare the actual output with the expected result. Assertions will ultimately be the end goal of a test case. If the assertion is true, the test passes; if not, it fails.
Example Unit Test in Python with Pytest
Setting Up Pytest
To start, you need to install Pytest. You can do this by running pip install pytest
in your terminal. Pytest looks for tests in files named starting with test_
or ending with _test.py
. This makes it easy to organize your tests.
Writing a Test for a Simple Function
Let’s say you have a simple function to test:
def add(a, b):
return a + b
You can write a test for this function like this:
def test_add():
assert add(2, 3) == 5
assert add(-1, 1) == 0
assert add(-1, -1) == -2
In this test, we are checking if the add
function returns the correct sum for different inputs.
Running and Analyzing Test Results
To run your tests, simply execute pytest
in your terminal. Pytest will find and run all the tests in your project. It will then report which tests passed and which failed. If a test fails, Pytest will show you what went wrong, making it easier to fix the issue.
Best Practices for Unit Testing
Maintaining Test Independence
Unit tests should be self-contained. Any dependencies can negatively impact the tests, making them hard to debug. Each test should run independently to ensure that one test’s outcome does not affect another.
Ensuring Test Coverage
Test many use cases. A single unit can be associated with multiple use cases, so you should test each in different test cases. This allows you to refactor code more efficiently. Aim for high test coverage to catch as many issues as possible.
Refactoring Tests Regularly
Regularly update your tests to keep them in sync with the codebase. As your code evolves, your tests should too. This helps in maintaining the relevance and accuracy of your tests.
Advanced Unit Testing Techniques
In this section, we will explore some advanced techniques that can take your unit testing skills to the next level. These methods will help you handle more complex scenarios and ensure your code is robust and reliable. Mastering these techniques can significantly improve your debugging efficiency and support agile practices.
Mocking Dependencies
Mocking is a technique used to simulate the behavior of complex objects or external systems. This is especially useful when you need to test a unit that interacts with a database, web service, or another external dependency. By using mocks, you can isolate the unit under test and focus on its behavior without worrying about the external systems.
Parameterizing Tests
Parameterizing tests allows you to run the same test with different inputs. This is a powerful way to ensure your code works correctly under various conditions. You can use frameworks like Pytest to easily parameterize your tests and cover more scenarios with less code.
Testing Asynchronous Code
Testing asynchronous code can be challenging, but it’s essential for applications that rely on asynchronous operations. Tools like Asyncio in Python can help you write tests for asynchronous functions, ensuring they behave as expected. This technique is crucial for modern applications that require high performance and responsiveness.
Conclusion
Unit testing is a powerful tool that helps you make sure your code works correctly. By breaking your program into smaller parts and testing each one, you can find and fix problems early. This step-by-step guide showed you how to write unit tests, from picking a function to test, to writing test cases, and using assertions. Remember, while unit tests can’t catch every bug, they are a great way to improve your code’s reliability. Keep practicing, and soon writing unit tests will become a natural part of your coding process. Happy coding!
Frequently Asked Questions
What is a unit test?
A unit test checks if a small part of a program, like a function, works as it should.
Why are unit tests important?
Unit tests help find and fix bugs early, making sure each part of the program works right.
How do I write a unit test?
Pick a function to test, write a test function with different inputs, and use assertions to check the results.
What is Pytest?
Pytest is a tool in Python that makes writing and running tests easy.
What are assertions?
Assertions are statements in tests that check if the output is what you expected.
How often should I run unit tests?
Run unit tests often, especially after making changes to the code, to catch any new bugs.