Unit Test Like a Pro: An Example to Improve Your Coding Process
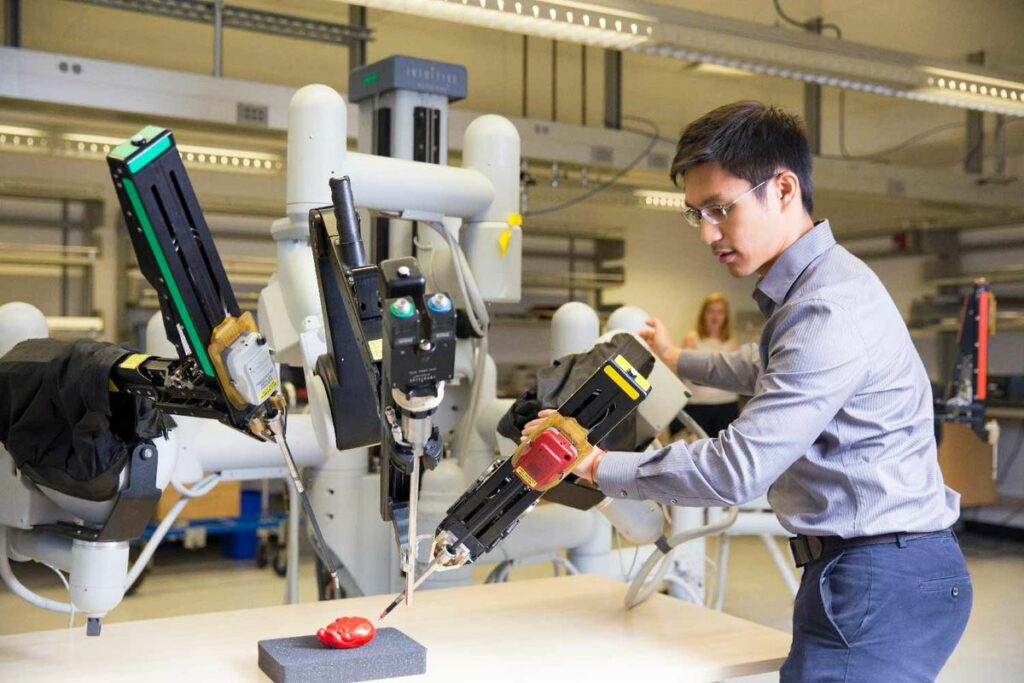
Unit testing is a fundamental practice in software development that ensures each piece of code, or ‘unit’, functions as intended. By isolating and testing individual units, developers can catch and resolve bugs early, preventing them from escalating into larger issues. This article, ‘Unit Test Like a Pro: An Example to Improve Your Coding Process’, will guide you through the best practices, tools, and mindsets needed to integrate effective unit testing into your coding process. We’ll explore how unit testing contributes to code quality, early bug detection, and overall software robustness, providing you with actionable insights to enhance your development workflow.
Key Takeaways
- Effective unit testing involves writing clear, concise test cases and embracing automation to streamline the testing process.
- Unit testing plays a crucial role in software development by ensuring code quality and facilitating early bug detection.
- Adopting a shift-left approach to testing allows for quicker resolution of bugs and continuous improvement of the codebase.
- Selecting the right unit testing tools and frameworks is essential for integrating unit tests into the development and build processes.
- Unit testing should be viewed not just as a practice but as a mindset, integral to the development workflow and documentation of the project.
Best Practices for Effective Unit Testing
Writing Clear and Concise Test Cases
Crafting test cases is akin to storytelling, where each step unfolds part of the narrative leading to the resolution of bugs. The clarity of test cases is paramount; they should guide the developer through the testing process without ambiguity. A well-written test case not only identifies the conditions necessary for the test but also outlines the expected outcomes in a manner that is easy to understand and execute.
To ensure that test cases are both clear and concise, consider the following points:
- Define the objective of each test case clearly.
- Use simple and understandable language.
- Break down complex tests into smaller, more manageable steps.
- Include the input data required, along with the expected result.
- Avoid unnecessary details that do not contribute to the test’s objective.
By adhering to these guidelines, developers can create test cases that are not only effective in catching bugs but also efficient in terms of time and resources spent on testing. This approach leads to a more streamlined and productive testing process, ultimately contributing to the overall quality of the software product.
Implementing Test Automation
Embracing test automation is a transformative step in unit testing, ensuring tests are not only repeatable but also efficient and reliable. Automation allows for the consistent execution of tests, providing immediate feedback on the health of the codebase.
To get started with test automation, follow these steps:
- Identify tests that are good candidates for automation, typically those that are run frequently and require precision.
- Utilize a Test Case Management tool to organize and maintain your test cases, saving time and reducing manual errors.
- Integrate automated tests into your continuous integration pipeline to ensure they are run at every code commit.
Remember, while automation can significantly improve your testing process, it’s not a silver bullet. Challenges such as maintaining the test suite and ensuring the relevance of tests over time still persist. However, with the right strategies, such as those outlined in the article ‘Top 10 Test Automation Strategies and Best Practices‘, you can overcome these hurdles and reap the benefits of automation.
Handling Dependencies and Integration Issues
When unit testing, handling dependencies can be as crucial as the tests themselves. A test case dependency exists when the outcome of one test relies on another. This can lead to brittle tests that fail when unrelated changes are made, complicating maintenance and obscuring the true source of issues.
To manage dependencies effectively, consider these strategies:
- Isolate the unit under test by using mock objects or stubs.
- Employ dependency injection to replace real dependencies with test doubles.
- Organize tests to run independently, ensuring no order dependency.
Integration issues arise when combining units that work well in isolation but falter together. To address this, ensure that:
- Integration points are well-defined and tested separately.
- Continuous Integration (CI) systems are in place to detect issues early.
- Test environments closely mirror production to catch environment-specific bugs.
By meticulously addressing dependencies and integration issues, developers can create a more stable and reliable codebase, paving the way for smoother integrations and fewer surprises during the development process.
Ensuring Code Quality Through Unit Testing
The Role of Unit Testing in Software Development
Unit testing serves as the foundation for ensuring that software behaves as specified, even when the source code undergoes modifications. It is a critical component of methodologies like Test Driven Development (TDD) and DevOps, which prioritize testing as an integral part of the development cycle.
The primary responsibility of unit testing lies with the developers, who are expected to write test cases in parallel with their code. This practice promotes comprehensive coverage and contributes to the overall quality of the software. By catching bugs early, developers can address issues before they escalate, making the process more efficient and cost-effective.
The importance of unit testing in software development can be summarized through its key benefits:
- Improves code quality
- Identifies bugs at an early stage
- Accelerates the development process
- Provides a safety net for code changes
- Facilitates confident code refactoring
Measuring Code Stability Over Time
Understanding the effectiveness of unit tests is crucial in ensuring the longevity and reliability of software. One way to measure this is through the Test Case Effectiveness (TCE) metric, which evaluates the quality and potency of test cases. This metric helps in identifying the stability of the code over time and the efficiency of the testing process.
To illustrate, consider the following table showing a simplified view of TCE over several iterations:
Iteration | TCE Score | Bugs Detected | Bugs Fixed |
---|---|---|---|
1 | 85% | 10 | 10 |
2 | 88% | 8 | 8 |
3 | 90% | 5 | 5 |
As the iterations progress, an increasing TCE score and a decreasing number of bugs detected and fixed can indicate a more stable and mature codebase. This data not only reflects the current health of the software but also guides future testing strategies.
Moreover, tracking the time taken to fix bugs provides additional insight into the responsiveness and agility of the development process. A shorter bug fixing time suggests that the unit tests are effectively identifying issues early, allowing for quicker resolutions and a smoother development cycle.
Utilizing Code Coverage Tools
Code coverage tools are essential in assessing the effectiveness of unit tests by quantifying the extent to which the codebase is tested. These tools act as a metric, providing a clear picture of untested areas and helping developers focus their testing efforts where it’s most needed.
Automated tools are available for code coverage analysis, employing various techniques such as Statement Coverage and Branch Coverage. Statement Coverage ensures that every statement in the code is tested at least once, while Branch Coverage verifies every possible path through the code, including conditional loops.
To achieve comprehensive code coverage, developers may use a combination of techniques, including Condition Coverage, Multiple Condition Coverage, Path Coverage, and Function Coverage. Typically, aiming for 80-90% code coverage using Statement and Branch coverage is considered sufficient for most projects.
Here’s a list of popular code coverage tools across different programming languages:
- Java: JaCoCo, Clover
- JavaScript: Istanbul, Jest
- C/C++: gcov, lcov
- C#: dotCover, OpenCover
- PHP: PHPUnit, phpdbg
- Eclipse: EclEmma
- .Net: NCover, Visual Studio Code Coverage
Early Bug Detection and Resolution
The Shift-Left Approach to Testing
Embracing the Shift-Left approach in testing is a strategic move that involves integrating testing early into the software development lifecycle. This proactive stance ensures that testing is not an afterthought but a fundamental aspect of the development process. By focusing on customer experience from the outset, testers can design tests that are more aligned with user expectations and requirements.
The benefits of Shift-Left testing are numerous, including the ability to detect and resolve bugs earlier, which can significantly reduce the cost and time required for fixing issues. Moreover, this approach encourages collaboration between developers, testers, and other stakeholders, fostering a more cohesive and efficient workflow.
To effectively implement Shift-Left testing, consider the following steps:
- Define clear testing objectives based on customer needs.
- Integrate testing into the initial stages of development.
- Prioritize testing of high-risk or critical paths.
- Continuously refine test cases based on feedback and iterative development.
Tracking and Fixing Bugs Efficiently
Efficient bug tracking and resolution are pivotal in maintaining a high-quality codebase. By monitoring bug fixing time, developers can assess the responsiveness of their unit testing strategies. A streamlined process for identifying and addressing bugs not only accelerates development but also enhances the stability of the software over time.
Unit testing enables the detection of the majority of bugs early in the software development lifecycle, making them less costly and easier to fix. This proactive approach to bug management is a cornerstone of effective software development, aligning with best practices that advocate for continuous improvement and automation.
To further refine bug tracking, consider the following steps:
- Centralize bug tracking to ensure visibility and accountability.
- Automate the replication and retesting of bugs to save time and reduce human error.
- Implement regular code reviews to catch bugs that may slip through automated tests.
- Foster a culture of quality where every team member is vigilant about detecting and resolving bugs swiftly.
Leveraging Unit Tests for Continuous Improvement
Continuous improvement in software development is pivotal, and unit tests play a crucial role in this iterative process. By consistently applying unit tests, developers can ensure that each change or addition to the codebase maintains or enhances the quality of the application. Regular refactoring becomes safer, as unit tests serve as a safety net, catching regressions or unintended side effects.
Unit tests also provide valuable metrics for assessing the progress of a project. For instance, tracking the time taken to fix bugs can highlight the efficiency of the testing strategy. Below is a table showing a simplified example of how bug resolution times can be tracked over several iterations:
Iteration | Number of Bugs | Average Resolution Time (hours) |
---|---|---|
1 | 10 | 4 |
2 | 8 | 3 |
3 | 5 | 2 |
4 | 3 | 1.5 |
As the table suggests, a decrease in the average resolution time indicates an improvement in the codebase’s stability and the effectiveness of the unit testing process. This quantitative feedback loop is essential for teams aiming to optimize their development practices and deliver high-quality software consistently.
Unit Testing Tools and Frameworks
Choosing the Right Unit Testing Framework
Selecting the appropriate unit testing framework is a pivotal decision that can influence the efficiency and effectiveness of your testing process. Frameworks differ in their capabilities, ease of use, and integration with other tools, making it crucial to evaluate them based on your project’s specific needs.
When considering a unit testing framework, take into account factors such as language compatibility, community support, and the framework’s ability to handle the complexity of your codebase. For instance, JavaScript developers might refer to resources like ‘JavaScript unit testing frameworks in 2024: A comparison – Raygun’ which examines the most popular frameworks to aid in decision-making.
Here’s a brief overview of some considerations when choosing a framework:
- Language Compatibility: Ensure the framework supports the programming language used in your project.
- Community Support: Look for a framework with a strong community for troubleshooting and sharing best practices.
- Feature Set: Assess the features offered, such as test case generation, mocking, and integration with CI/CD pipelines.
- Performance: Consider the execution speed and resource consumption of the framework.
Remember, the right framework is one that aligns with your team’s workflow and enhances your unit testing strategy.
Integrating Unit Tests into the Build Process
Integrating unit tests into the build process is a critical step in ensuring that code quality is maintained throughout the development lifecycle. Automated unit tests should be executed as part of the continuous integration (CI) pipeline, providing immediate feedback on the health of the codebase.
To effectively integrate unit tests, follow these steps:
- Configure the build system to trigger unit tests after each commit.
- Ensure that tests are fast and reliable to avoid bottlenecks.
- Treat failing tests as a priority and fix them before proceeding.
- Use test sessions to group and manage tests efficiently.
By making unit tests a core part of the development workflow, developers are encouraged to write tests immediately after delivering code. This practice helps in identifying and resolving bugs early, which is not only cost-effective but also enhances the stability of the application over time.
Exploring Advanced Tools for Test Case Management
Advanced tools for test case management are essential for streamlining the software quality assurance process. They offer a range of features that can significantly reduce the time spent on manual testing. For instance, JUnit and NUnit are frameworks that facilitate advanced test case management and integration with other tools.
Choosing the right tool can be a daunting task, but it’s crucial for enhancing your testing workflow. The QA Lead’s review titled ‘17 Best Test Management Tools Reviewed For 2024‘ provides a comprehensive list of tools that cater to various needs. Whether you’re working on a small project or a large enterprise, tools like TestLink offer features to manage test cases, keywords, requirements, and more, making them a good fit for projects of any size.
Here are some popular test case management tools to consider:
- Bugzilla Testopia: An extension to the Bugzilla defect tracking tool.
- TestCube: A web-based test case management system.
- RTH-turbo: Another web-based test management tool.
- Testitool: Manages test plans and test cases.
It’s recommended to use these tools and determine which one best suits your project’s needs. Remember, the goal is to enhance efficiency and ensure a robust testing process.
Cultivating a Unit Testing Mindset
Unit Testing as a Core Part of Development Workflow
Incorporating unit testing as an integral part of the development workflow is not just a best practice; it’s a paradigm shift that can lead to significant improvements in software quality. By treating tests as a fundamental aspect of coding, rather than an afterthought, developers can catch issues early and ensure that each piece of code performs as expected before it is integrated into the larger codebase.
Unit testing should be conducted as soon as a new function, method, or class is written. This immediate feedback loop allows for the early detection of bugs, which are often less costly and simpler to resolve at this stage. Moreover, unit tests serve as a living documentation for the code, clarifying the intended behavior and making future changes safer and easier to implement.
To effectively embed unit testing into the development process, consider the following steps:
- Define clear testing criteria for each unit of code.
- Integrate unit tests into the automated build process.
- Regularly review and update test cases to reflect code changes.
- Encourage a culture where writing tests is as valued as writing the feature code itself.
Documenting Projects Through Test Cases
Effective documentation is a cornerstone of successful unit testing. Test cases serve not only as a means to verify code functionality but also as a project’s documentation. They provide a clear narrative of the software’s capabilities and limitations, which is invaluable for both current team members and future maintainers.
A well-documented test case should include the test setup, inputs, expected results, and actual results. This structured approach ensures that anyone reviewing the test cases can understand the intent and outcome without ambiguity. Here’s a simple template for documenting a test case:
Test Case ID | Description | Setup | Input | Expected Result | Actual Result |
---|---|---|---|---|---|
TC001 | User login | Setup user environment | Username and password | Successful login | As expected |
By consistently documenting test cases, teams can streamline the onboarding process for new developers and reduce the learning curve. Moreover, it aids in maintaining a high level of code quality throughout the project’s lifecycle, as the documentation can be used to identify areas of improvement and ensure that all necessary test scenarios are covered.
Adopting a Proactive Approach to Testing
Adopting a proactive approach to testing involves anticipating potential issues and addressing them before they manifest into larger problems. Shift-left testing is a key strategy in this approach, emphasizing the importance of testing early and often in the development cycle. By integrating testing into the initial stages of software development, teams can identify and resolve defects more efficiently, leading to a more stable and reliable product.
A proactive testing mindset encourages continuous improvement and learning. It’s not just about finding bugs; it’s about understanding the root causes and refining the development process to prevent similar issues in the future. This approach aligns with the evolving landscape of software testing, as highlighted in the article ‘Software Testing in 2024: Innovations and Transformations’, which discusses the paradigm shift towards more proactive testing methods.
To effectively adopt this mindset, consider the following points:
- Engage in regular code reviews to catch issues early.
- Implement automated testing to ensure consistency and save time.
- Foster collaboration between testers and developers to share insights and improve code quality.
- Utilize test case management tools to organize and track testing efforts, as suggested by DZone contributors.
Conclusion
In summary, unit testing is an indispensable component of the software development lifecycle. It not only ensures code quality and facilitates early bug detection but also serves as a living documentation for your project. By adopting best practices such as writing clear and concise test cases, implementing test automation, and overcoming common challenges like time constraints and dependencies, developers can greatly enhance the robustness and maintainability of their code. Remember, unit testing is not merely a task to be checked off; it’s a mindset that, when embraced, can transform your code into a reliable and efficient masterpiece. As we conclude this guide, take these insights and integrate unit testing into your coding process like a pro, and may your development journey be smooth and virtually bug-free.
Frequently Asked Questions
What are the benefits of writing clear and concise test cases?
Clear and concise test cases improve test readability, make maintenance easier, and ensure that tests are focused on specific functionalities, which aids in early bug detection and efficient resolution.
How does implementing test automation benefit the development process?
Test automation speeds up the testing process, allows for frequent and consistent test execution, reduces manual effort, and helps in catching regressions quickly, thereby improving code quality.
What is the role of unit testing in software development?
Unit testing plays a critical role in software development by verifying the functionality of individual units of code, ensuring they work as intended, which leads to a more stable and reliable software product.
How can code coverage tools enhance unit testing?
Code coverage tools measure the extent to which the source code is executed by the tests, helping developers identify untested parts of the codebase and ensuring thorough testing for better quality assurance.
What is the shift-left approach to testing?
The shift-left approach involves integrating testing early and often in the development lifecycle, allowing teams to detect and fix bugs sooner, which can reduce costs and improve the software’s overall quality.
Why is it important to integrate unit tests into the build process?
Integrating unit tests into the build process ensures that tests are run automatically with every build, helping to identify issues early, maintain code quality, and facilitate continuous integration practices.