Mastering Unit Testing: A Comprehensive Guide
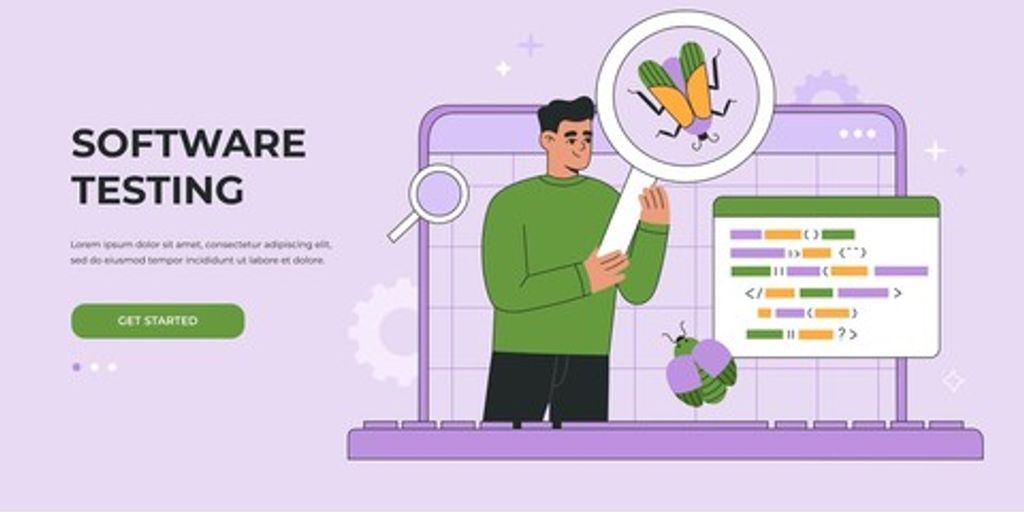
Unit testing is a key part of making sure software works well. In this guide, we’ll walk you through everything you need to know about unit testing. Whether you’re new to this or have some experience, you’ll find useful tips and best practices to help you write better tests. Let’s get started on our journey to mastering unit testing!
Key Takeaways
- Unit testing helps catch bugs early, saving time and effort later.
- There are many tools and frameworks available to make unit testing easier.
- Writing clear and simple tests makes your code easier to maintain.
- Advanced techniques like parameterized tests can make your testing more powerful.
- Integrating unit tests into your workflow ensures continuous quality.
Understanding the Basics of Unit Testing
Definition and Importance
Unit testing is a method where individual parts of a software are tested separately. The main aim is to check that each part works correctly. This is very important because it helps find problems early, making the software better and easier to maintain.
Key Concepts and Terminology
Knowing the common terms in unit testing is key:
- Test Case: A set of conditions to check if a part of the software works.
- Test Suite: A collection of test cases.
- Mock Object: A fake object that mimics the behavior of real objects in controlled ways.
- Assertion: A statement that checks if a condition is true.
Common Misconceptions
There are some misunderstandings about unit testing:
- Unit testing is time-consuming: While it takes time to write tests, it saves time by finding bugs early.
- Only testers write tests: Developers should also write unit tests to ensure their code works.
- Unit tests are hard to maintain: Good practices make unit tests easier to keep up-to-date.
Essential Tools and Frameworks for Unit Testing
In the world of software development, ensuring the quality and reliability of your code is paramount. Unit testing has emerged as a crucial practice that allows developers to validate the functionality of individual units or components of their applications. This section will provide an in-depth exploration of these tools, their features, and how they can be leveraged to write effective and maintainable unit tests.
Best Practices for Writing Unit Tests
Writing Readable and Maintainable Tests
When writing unit tests, it’s important to keep them clear and easy to understand. Each test should focus on a single unit of work. This makes it easier to find and fix bugs. Use descriptive names for your tests so you know what each one is checking. Follow the AAA pattern: Arrange, Act, Assert. This helps organize your test logic. Avoid hardcoding values; use variables and constants instead to make your tests more flexible.
Mocking and Stubbing Dependencies
Mocking and stubbing are techniques used to simulate the behavior of real objects. This is useful when you want to test a unit of code without relying on external systems. By using mocks and stubs, you can isolate the code you’re testing and ensure that your tests are reliable. This approach helps in verifying the behaviors of an individual unit of source code without interference from other parts of the system.
Ensuring Test Coverage
Test coverage is a measure of how much of your code is tested by your unit tests. Aim for high test coverage to catch as many bugs as possible. However, remember that 100% coverage doesn’t guarantee bug-free code. Focus on covering both normal and boundary conditions. This means testing not just the usual cases, but also the edge cases that might cause unexpected behavior. By doing this, you can uncover issues that might not be obvious at first glance.
Advanced Unit Testing Techniques
Parameterized Tests
Parameterized tests let you run the same test with different inputs, making your test suite more thorough and cutting down on repeated code. This method is especially handy for testing functions with many input scenarios. By using parameterized tests, you can check that your code works correctly under different conditions without writing separate test cases for each one.
Testing Asynchronous Code
Testing asynchronous code can be tricky, but it’s important to make sure your code works as expected when dealing with tasks that don’t run immediately. Using the right tools and techniques can help you manage this complexity. For example, many frameworks offer built-in support for testing async functions, allowing you to wait for promises to resolve before making assertions.
Property-Based Testing
Property-based testing is a technique where you define properties that your code should always satisfy, and then the testing framework generates a wide range of inputs to check these properties. This approach can uncover edge cases that you might not have thought of. It’s a powerful way to ensure your code behaves correctly across a broad spectrum of inputs.
Integrating Unit Tests into CI/CD Pipelines
Integrating unit tests into CI/CD pipelines is a crucial step in modern software development. Automating tests in CI/CD pipelines ensures that tests are automatically executed whenever changes are made to the codebase, catching issues early in the development process. This practice aligns with most DevOps principles and helps maintain code quality.
Setting Up Continuous Integration
Unit testing becomes even more powerful when integrated with continuous integration (CI) systems. By incorporating unit tests into your CI workflow, you can automatically run the tests whenever changes are made to the codebase. Here’s a typical workflow for integrating unit tests with CI:
- Developers write unit tests for their code and commit the changes to a version control system (e.g., Git).
- The CI tool detects the changes and triggers the build process.
- The CI tool runs the unit tests as part of the build process.
- If the tests pass, the build is marked as successful. If any test fails, the build is marked as failed, and developers are notified.
Automating Test Execution
Automating unit tests within your CI/CD pipeline ensures that every code change is validated through a series of tests. This process typically involves:
- Setting up your CI/CD tool to run your test suite automatically on code changes.
- Configuring the tool to generate reports on test results.
- Ensuring that the pipeline fails if any test does not pass, preventing faulty code from being deployed.
Monitoring and Reporting
Effective monitoring and reporting are essential for maintaining the health of your CI/CD pipeline. By keeping an eye on test results and build statuses, you can quickly identify and address issues. Key practices include:
- Using dashboards to visualize test results and build statuses.
- Setting up alerts to notify developers of test failures.
- Regularly reviewing test reports to identify flaky tests or areas needing improvement.
Maintaining and Refactoring Unit Tests
Identifying Flaky Tests
Flaky tests are those that sometimes pass and sometimes fail without any changes to the code. These tests can be a major headache because they make it hard to know if your code is really working. Regularly review and fix flaky tests to keep your test suite reliable. Use tools to track test stability and identify patterns that might cause flakiness.
Refactoring for Better Performance
Refactoring your tests is as important as refactoring your code. Follow these strategies to keep your tests clean and maintainable:
- Simplify complex tests to make them easier to understand.
- Remove redundant tests that don’t add value.
- Optimize test setup and teardown processes to save time.
Keeping Tests Up-to-Date
Maintaining a robust test suite is essential for effective CI/CD integration. Regularly review and update your tests to ensure they cover new features and edge cases. Use code coverage tools to measure the percentage of code covered by your tests and identify areas that need additional testing. This practice helps in keeping your test suite lean and efficient.
Extending Unit Testing Beyond Functional Tests
Performance Testing
Performance testing ensures that your application runs smoothly under various conditions. It’s crucial to identify bottlenecks and optimize performance. This type of testing helps in understanding how your application behaves under stress, ensuring it can handle high loads without issues.
Load Testing
Load testing involves simulating multiple users accessing your application simultaneously. This helps in identifying the maximum operating capacity of your application and any potential weak points. By doing so, you can ensure that your application remains stable and performs well under heavy traffic.
Security Testing
Security testing is essential to protect your application from vulnerabilities. It involves checking for potential security threats and ensuring that your application is secure from attacks. This type of testing helps in safeguarding sensitive data and maintaining user trust.
Conclusion
Mastering unit testing is a journey that can greatly improve the quality of your software. By understanding and applying the principles and best practices discussed in this guide, you can write tests that are both effective and reliable. Whether you’re just starting out or looking to enhance your skills, unit testing is an essential tool in a developer’s toolkit. Keep practicing, stay curious, and continue to refine your approach. Your efforts will lead to more robust and maintainable code, ultimately making your software better for everyone.
Frequently Asked Questions
What is unit testing?
Unit testing is when you test small parts of your code, like functions or methods, to make sure they work right.
Why is unit testing important?
Unit testing helps catch bugs early, making your code more reliable and easier to fix.
What are some popular tools for unit testing?
Some popular tools are JUnit, NUnit, and Mocha. They help you write and run your tests.
How do I write good unit tests?
Good unit tests are easy to read and understand. They should test one thing at a time and be repeatable.
What is mocking in unit testing?
Mocking is when you create fake objects or functions to test parts of your code without relying on real ones.
How do unit tests fit into CI/CD pipelines?
Unit tests can be automated in CI/CD pipelines to run every time you make changes to your code, ensuring everything still works.