Unit Testing Software: Best Practices and Tools for Developers
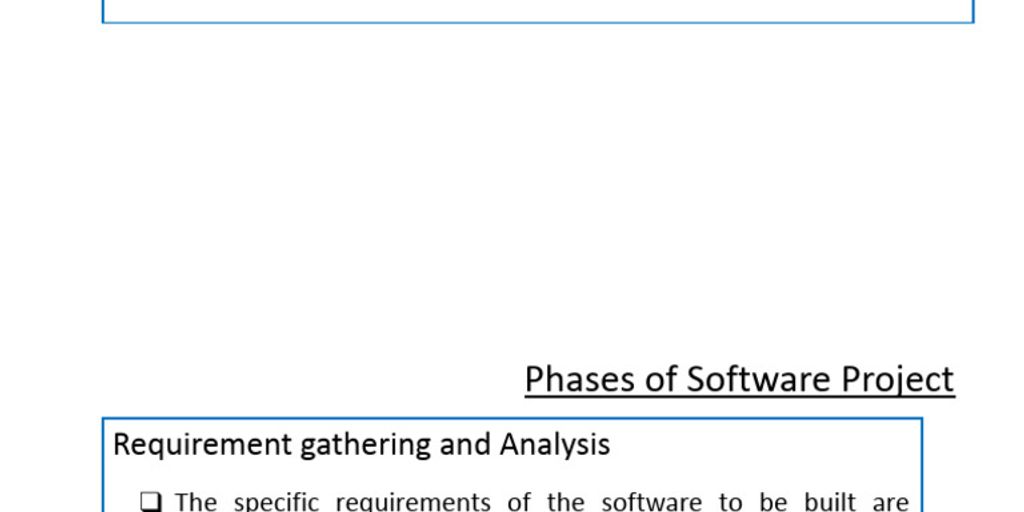
Unit testing is a method where developers test individual parts of their code to make sure everything works correctly. This is the first step in testing before the code is given to a testing team. By doing unit tests, developers can catch errors early and ensure that each part of the code does what it’s supposed to do. This article will explain what unit testing is, the best tools to use, and the best ways to do it.
Key Takeaways
- Unit testing helps developers catch errors early by testing small parts of the code.
- Using the right tools can make unit testing easier and more effective.
- Writing testable code is important for successful unit testing.
- It’s crucial to isolate tests to ensure they are reliable.
- Maintaining test suites helps keep the codebase healthy.
Understanding Unit Testing Software
Definition and Importance
Unit testing, also known as component or module testing, is a type of software testing where individual parts of a software application are tested separately. This method ensures that each part of the software works as expected. By focusing on small, manageable parts of the application, unit testing helps identify and fix bugs early in the development process.
Key Characteristics
Unit tests are usually automated, ensuring that a section of the software meets expectations. They can also be done manually. Key characteristics of unit testing include:
- Testing isolated parts of the code
- Early detection of bugs
- Simplifying debugging
- Providing documentation of the code
Common Misconceptions
There are several misconceptions about unit testing. Some believe it is time-consuming and unnecessary, but in reality, it saves time by catching bugs early. Others think it requires advanced skills, but even beginners can learn and apply unit testing effectively.
Top Tools for Unit Testing Software
Popular Frameworks
There are many tools and frameworks available for unit testing in different programming languages. Here are some of the most commonly used ones:
- JUnit: A popular unit testing framework for Java. It is easy to use and provides annotations to identify test methods, setup and teardown procedures, and test cases. JUnit also integrates well with popular development environments and build tools.
- NUnit: Similar to JUnit but designed for .NET languages. It offers a rich set of features and is widely used in the .NET community.
- pytest: A robust testing framework for Python. It supports fixtures and a variety of plugins to extend its functionality.
- Jest: A delightful JavaScript testing framework with a focus on simplicity. It works well with projects using React.
Tool Comparison
Here’s a quick comparison of some popular unit testing tools:
Tool | Language | Key Features |
---|---|---|
JUnit | Java | Annotations, Integration with IDEs |
NUnit | .NET | Rich feature set, Community support |
pytest | Python | Fixtures, Plugin support |
Jest | JavaScript | Simplicity, React compatibility |
Choosing the Right Tool
Selecting the right unit testing tool depends on several factors:
- Language Compatibility: Ensure the tool supports the programming language you are using.
- Community and Support: A tool with a large community can offer better support and more resources.
- Integration: Check if the tool integrates well with your development environment and build tools.
- Ease of Use: The tool should be easy to set up and use, especially for beginners.
By considering these factors, you can choose a unit testing tool that best fits your project’s needs.
Best Practices for Effective Unit Testing
Writing Testable Code
To ensure your code is easy to test, it’s crucial to write it in a way that makes testing straightforward. Each unit test should focus on a small piece of functionality. This means your tests should be clear and concentrate on a single concept or condition. This approach makes it easier to understand what is being tested and why a test might fail.
Isolating Tests
Isolating tests is key to effective unit testing. When tests are isolated, they do not depend on external systems or other tests. This isolation ensures that the tests are reliable and their results are consistent. One way to achieve this is by using the AAA pattern: Arrange, Act, Assert. This pattern helps in structuring tests clearly and makes the objective of testing quite clear.
Maintaining Test Suites
Maintaining test suites is essential for long-term success in unit testing. Regularly update your tests to reflect changes in the codebase. This practice helps in catching bugs early and ensures that the tests remain relevant. Additionally, use descriptive test names to make it easier for others to understand what each test is doing.
Challenges in Unit Testing Software
Common Pitfalls
Many software teams struggle with unit testing. They often start by making mistakes that could be avoided with proper education on the subject. Quickly, their unit testing strategy descends into a mess, and the team decides it’s no longer worth the trouble and gives up on the effort. That’s a shame since unit testing doesn’t have to be hard if you do it right, by following best practices from the start.
Overcoming Obstacles
It can also be challenging to isolate and test independent units, making discerning the source of faults elicited during the testing process very difficult. The developer frequently performs manual testing and assesses the software’s stability after adding or removing lines of code.
Real-World Examples
One of the obstacles in the way of teams trying to adopt software testing is test maintenance. When tests—unit and otherwise—are too fragile and fail all the time due to the slightest change to the codebase, maintaining the tests becomes a burden.
Integrating Unit Testing into Development Workflow
Continuous Integration
Continuous Integration (CI) is a practice where developers frequently merge their code changes into a shared repository. Each merge triggers an automated build and test process, ensuring that new code integrates smoothly with the existing codebase. This helps catch defects early and maintains the overall health of the project. Developing a comprehensive plan for the integration testing process is crucial, outlining the scope, objectives, resources, and methodologies that work for your project.
Automated Testing Pipelines
Automated testing pipelines streamline the testing process by automatically running tests whenever code changes are made. This reduces the need for manual testing and speeds up the development cycle. Automated pipelines can include unit tests, integration tests, and other types of tests to ensure comprehensive coverage. They help in maintaining a consistent and reliable testing process, which is essential for delivering high-quality software.
Collaboration Between Teams
Effective unit testing requires collaboration between different teams, including developers, testers, and operations. By working together, teams can ensure that tests are comprehensive and cover all aspects of the application. Unit tests can also help onboard new team members by providing a clear understanding of the codebase. This collaboration fosters a culture of quality and continuous improvement, making it easier to identify and fix issues early in the development process.
Advanced Techniques in Unit Testing Software
Mocking and Stubbing
Mocking and stubbing are essential techniques in unit testing. They help simulate the behavior of complex objects. Mocking involves creating fake objects that mimic real ones, while stubbing provides predefined responses to method calls. These techniques are crucial for isolating tests and ensuring they run independently of external systems.
Behavior-Driven Development
Behavior-Driven Development (BDD) is a collaborative approach that enhances communication between developers, testers, and business stakeholders. BDD focuses on the behavior of the software from the user’s perspective. It uses simple language to describe the expected behavior, making it easier to create test cases that align with business requirements.
Test-Driven Development
Test-Driven Development (TDD) is a practice where developers write tests before writing the actual code. This approach ensures that the code meets the test requirements from the start. TDD helps in identifying issues early and promotes writing cleaner, more maintainable code. It also encourages developers to think about the input-output specifications without knowing the implementation details.
Evaluating the Impact of Unit Testing
Measuring Code Coverage
Code coverage is a key metric in unit testing. It shows how much of the code is tested by unit tests. High code coverage means more of the code is tested, which can lead to fewer bugs. Tools like JaCoCo and Istanbul can help measure this.
Assessing Test Effectiveness
It’s not just about how much code is tested, but how well it’s tested. Effective tests catch bugs early and ensure the software works as expected. Developers should focus on writing meaningful tests that cover different scenarios.
ROI of Unit Testing
Investing time in unit testing can save money in the long run. By catching bugs early, developers can avoid costly fixes later. This leads to a more stable and reliable software product. The return on investment (ROI) of unit testing is seen in reduced maintenance costs and improved software quality.
Conclusion
Unit testing is a key part of making sure software works well. By breaking down code into small parts and testing each one, developers can find and fix problems early. Using the right tools and following best practices makes this process easier and more effective. Remember, good unit testing leads to better software and happier users. Keep learning and improving your testing skills to stay ahead in the game.
Frequently Asked Questions
What is unit testing?
Unit testing is when developers test small parts of their code to make sure each part works right. It’s the first step before the testing team checks the whole program.
Why is unit testing important?
Unit testing helps find and fix problems early, which makes the software more reliable and easier to maintain.
Who performs unit testing?
Usually, developers do unit testing themselves.
What are some common tools for unit testing?
Some popular tools for unit testing include JUnit, NUnit, and pytest.
What are best practices for unit testing?
Best practices include writing testable code, keeping tests isolated, and regularly updating test suites.
What are common challenges in unit testing?
Common challenges include dealing with complex code, maintaining test cases, and ensuring tests run fast.